In Python, a tuple is an ordered, immutable collection of elements, typically used to group related data together. Tuples are very similar to lists, with the main difference being that tuples are immutable, meaning that once they are created, their elements cannot be modified. Because of this, tuples can be used in situations where data should not be modified after creation, or where it is desirable to protect the data from being accidentally changed. The index value of tuple starts from 0.
Tuples use parentheses (), whereas lists use square brackets [ ].
Examples:

- Creation of Tuples
Creating Empty Tuples :
To create an empty tuple in Python, you can simply use a pair of parentheses with nothing inside:
empty_tuple = ()
This creates an empty tuple named empty_tuple
. Alternatively, you can use the tuple()
constructor function to create an empty tuple:
empty_tuple = tuple()
This has the same effect as using empty parentheses. Empty tuples can be useful as placeholders for values that will be filled in later, or in cases where you need to pass a tuple to a function but don’t have any data to include in it. Note that once a tuple is created, you cannot add or remove elements from it, so an empty tuple will remain empty unless you assign values to it.
Creating tuple with single element
To create a tuple with a single element in Python, you need to be careful because using just parentheses to enclose the element will create a different type of object, a string, integer or float. For example, if you write:
my_tuple = (4)
The my_tuple
variable will not be a tuple but an integer value of 4. To create a tuple with a single element, you can add a trailing comma after the element within parentheses:
my_tuple = (4,)
This creates a tuple with a single element of value 4. It is important to add the comma to distinguish the tuple from an integer value. Here is another example:
my_tuple = ("apple",)
This creates a tuple with a single element of value “apple”.
Without the trailing comma, Python will interpret it as a string, like this:
my_string = ("apple")
In this case, my_string
will be a string variable, not a tuple.
Creation of Tuples with Multiple Values
To create a tuple with multiple values in Python, you can separate each element with a commmy_tuple = (1, 2, “three”, 4.0)a, and enclose them all in parentheses:
my_tuple = (1, 2, "three", 4.0)
This creates a tuple named my_tuple
with four elements: the integers 1 and 2, the string “three”, and the floating-point number 4.0. You can create a tuple with any number of elements, and each element can be of a different type. It is also possible to create a tuple without explicitly using parentheses, as long as there are commas between the values:
my_tuple = 1, 2, "three", 4.0
This has the same effect as the previous example. You can also use variables to create a tuple:
x = 1
y = 2
my_tuple = (x, y)
In this case, my_tuple
will be a tuple containing the values of x
and y
, which are both integers. Tuples are flexible data structures that can hold any combination of values, and are often used to group related data together in a way that is easy to work with.
Example :
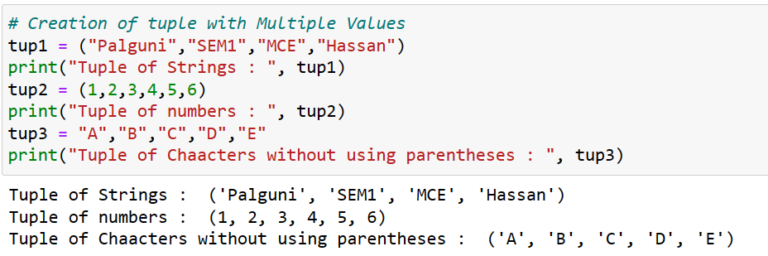
Example :
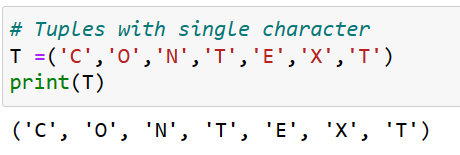
Example :
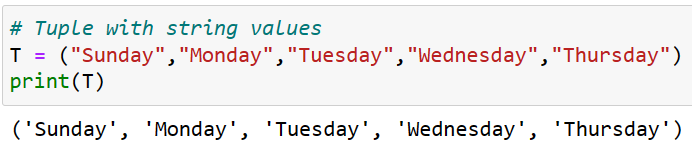
2. Add new element to Tuple
In Python, tuples are immutable data structures, which means that once a tuple is created, you cannot add, remove, or modify its elements. If you need to add a new element to a tuple, you will need to create a new tuple that includes the original tuple’s elements as well as the new element.
Here’s an example of how to create a new tuple by adding a new element to an existing tuple:
original_tuple = (1, 2, 3)
new_tuple = original_tuple + (4,)
In this example, we start with an original tuple (1, 2, 3)
. We create a new tuple new_tuple
by concatenating the original tuple with a new tuple containing the single element 4
using the +
operator. Note that we also include a comma after the 4
to ensure that it is interpreted as a tuple with a single element, rather than just a number.
After running this code, new_tuple
will contain (1, 2, 3, 4)
.
It’s important to note that creating a new tuple in this way can be inefficient if you are working with very large tuples, since it involves creating a new copy of the entire tuple. If you need to add or remove elements frequently, you might want to consider using a different data structure, such as a list, which allows you to modify its contents.
Example :
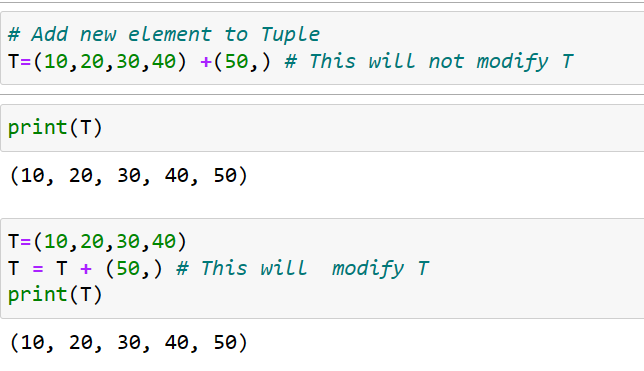
Accessing Values in Tuples
The values in tuples can be accessed using squared brackets , index number and slicing . You can access individual values within a tuple in Python using indexing. Indexing starts at 0 for the first element of the tuple, 1 for the second element, and so on. For example, given the tuple:
my_tuple = ('apple', 'banana', 'cherry', 'date')
You can access the first element using:
my_tuple[0] # returns 'apple'
The second element can be accessed using:
my_tuple[1] # returns 'banana'
And so on. You can also use negative indexing to access elements from the end of the tuple. The last element can be accessed using:
my_tuple[-1] # returns 'date'
The second-last element can be accessed using:
my_tuple[-2] # returns 'cherry'
And so on.
If you try to access an index that is out of range, you will get an IndexError. For example, if you try to access my_tuple[4]
in the example above, you will get an IndexError because the tuple only has four elements.
In addition to indexing, you can also use slicing to access a subset of the elements in a tuple. Slicing allows you to extract a range of elements from the tuple, and returns a new tuple containing those elements.
For example, to get the second and third elements of the my_tuple
tuple, you can use slicing:
my_slice = my_tuple[1:3] # returns ('banana', 'cherry')
In this example, the slice notation [1:3]
indicates that we want to extract elements starting at index 1 (the second element) up to, but not including, index 3 (the fourth element). The result is a new tuple containing the elements ‘banana’ and ‘cherry’.
Example :
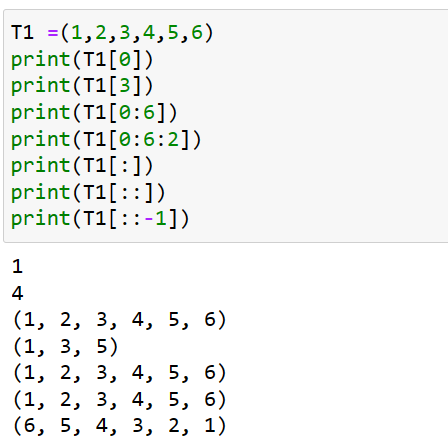
Updating Tuples
In Python, tuples are immutable, which means that once a tuple is created, its contents cannot be modified. This means that you cannot add, remove or update elements in a tuple once it has been created.
However, you can create a new tuple with updated values based on the existing tuple. To do this, you can use slicing and concatenation to extract the parts of the tuple you want to keep, and add or replace the elements you want to update. Here’s an example:
my_tuple = (1, 2, 3, 4)
new_tuple = my_tuple[:2] + (5,) + my_tuple[3:]
In this example, we start with a tuple my_tuple
that contains the values (1, 2, 3, 4)
. We create a new tuple new_tuple
by extracting the first two elements of my_tuple
using slicing (my_tuple[:2]
), adding the value 5
as a new element in a tuple ((5,)
), and then concatenating the remaining elements of my_tuple
after the fourth element using slicing (my_tuple[3:]
).
After running this code, new_tuple
will contain the values (1, 2, 5, 4)
. Note that we are not actually modifying the original tuple, but creating a new tuple with the desired values.
Although tuples are immutable, they are useful data structures because they allow you to group related data together in a way that is easy to work with, and can be passed around in your code without fear of the contents being modified.
Example :
Tuples are immutable and hence cannot change the value of tuple elements . Consider the following example :
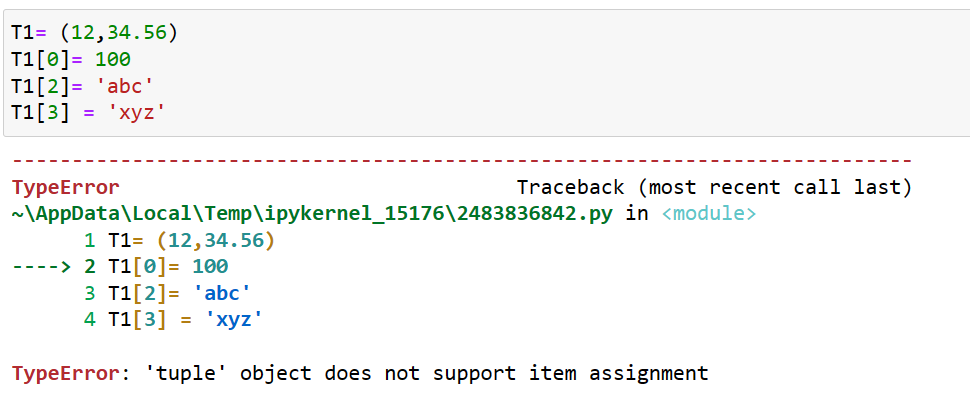
In the above example attempt is made to create tuple of the form (100, 34.56, ‘abc’, ‘xyz’) by item assignment . Type error is generated because python does not support item assignment for tuple objects. However a new tuple can be generated using concatenation as illustrated below :
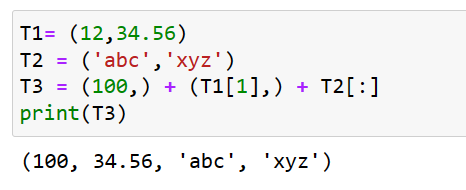
Deleting Tuple Elements
In Python, tuples are immutable, which means that once a tuple is created, its contents cannot be modified. This means that you cannot delete elements from a tuple. However, you can create a new tuple that contains all the elements of the original tuple except for the one you want to remove.
To remove an element from a tuple, you can use slicing and concatenation to create a new tuple that contains all the elements before the one you want to remove, as well as all the elements after the one you want to remove. Here’s an example:
my_tuple = (1, 2, 3, 4)
new_tuple = my_tuple[:2] + my_tuple[3:]
In this example, we start with a tuple my_tuple
that contains the values (1, 2, 3, 4)
. We create a new tuple new_tuple
by extracting the first two elements of my_tuple
using slicing (my_tuple[:2]
), and concatenating the remaining elements of my_tuple
after the third element using slicing (my_tuple[3:]
).
After running this code, new_tuple
will contain the values (1, 2, 4)
, which is the original tuple with the third element (3
) removed. Note that we are not actually modifying the original tuple, but creating a new tuple with the desired values.
Although tuples are immutable and you cannot delete elements from them, they are still useful data structures because they allow you to group related data together in a way that is easy to work with, and can be passed around in your code without fear of the contents being modified.
Example :
Tuple object does not support item or range of items deletion :
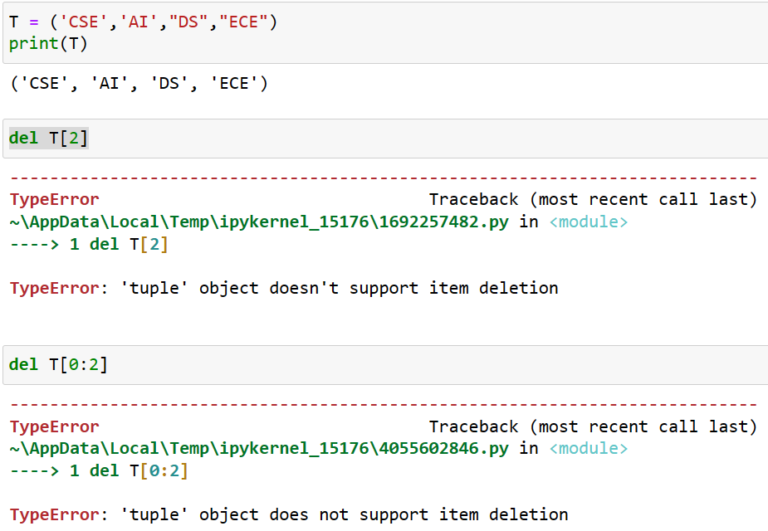
However the entire tuple can be deleted as illustrated below :
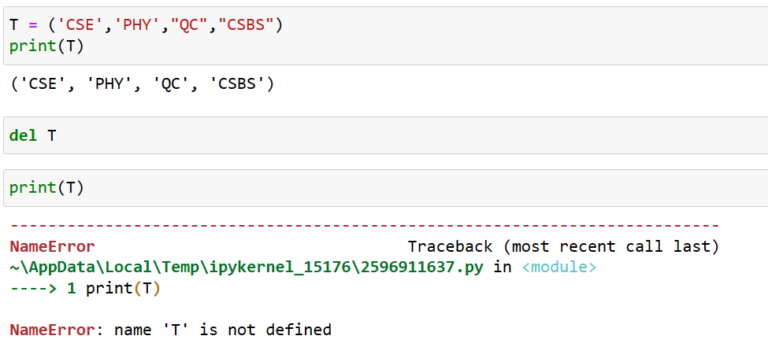
Basic Tuple Operations
Here are some basic tuple operations in Python:
- Concatenation: You can concatenate two tuples using the
+
operator. The result is a new tuple that contains all the elements from both tuples.
tup1 = (1, 2, 3)
tup2 = (4, 5, 6)
tup3 = tup1 + tup2
print(tup3) # Output: (1, 2, 3, 4, 5, 6)
2. Repetition: You can repeat a tuple multiple times using the *
operator. The result is a new tuple that contains the original tuple repeated the specified number of times.
tup1 = (1, 2, 3)
tup2 = tup1 * 3
print(tup2) # Output: (1, 2, 3, 1, 2, 3, 1, 2, 3)
3. Membership Test: You can check if an element is present in a tuple using the in
operator. The result is a Boolean value indicating whether the element is present in the tuple.
tup1 = (1, 2, 3)
print(2 in tup1) # Output: True
print(4 in tup1) # Output: False
4. Length: You can find the length of a tuple using the len()
function. The result is an integer representing the number of elements in the tuple.
tup1 = (1, 2, 3)
print(len(tup1)) # Output: 3
5. Index: You can find the index of an element in a tuple using the index()
method. The result is an integer representing the position of the element in the tuple.
tup1 = (1, 2, 3)
print(tup1.index(2)) # Output: 1
6. Count: You can count the number of occurrences of an element in a tuple using the count()
method. The result is an integer representing the number of times the element appears in the tuple.
tup1 = (1, 2, 3, 2)
print(tup1.count(2)) # Output: 2
7. Iteration : To iterate over a tuple, you can use a for loop, which will iterate over each element of the tuple in order. Here is an example:
my_tuple = (1, 2, 3, 4, 5)
for item in my_tuple:
print(item)
# Output :
1
2
3
4
5
In the above example, we first create a tuple called my_tuple
with five elements. We then use a for loop to iterate over each element of the tuple and print it to the console.
You can also use the enumerate()
function to iterate over a tuple and get the index of each element. Here is an example:
my_tuple = ('apple', 'banana', 'orange')
for index, item in enumerate(my_tuple):
print(index, item)
# output :
0 apple
1 banana
2 orange
In the above example, we use the enumerate()
function to iterate over the my_tuple
tuple and get the index and value of each element. The enumerate()
function returns a tuple with two values: the index of the current item and the item itself. We then use two variables, index
and item
, to capture these values and print them to the console.
Example :
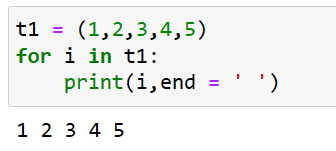
Example :
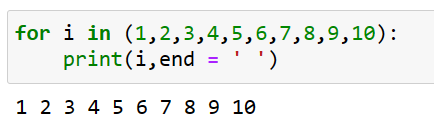
Tuple Assignment
Tuple assignment is a feature in Python that allows you to assign multiple variables at once using a single tuple. The syntax for tuple assignment involves putting the values you want to assign in a tuple on the right-hand side of the equals sign (=), and the variables you want to assign them to on the left-hand side of the equals sign. Here is an example:
x, y = 3, 4
In this example, we are assigning the value 3 to the variable x and the value 4 to the variable y. This is equivalent to writing:
x = 3
y = 4
Another example:
a, b, c = "apple", "banana", "cherry"
Here, we are assigning the string “apple” to the variable a, the string “banana” to the variable b, and the string “cherry” to the variable c.
You can also use tuple unpacking to swap the values of two variables, like this:
a, b = b, a
This code will swap the values of the variables a and b.
Tuple assignment can also be used to assign values from a function that returns a tuple. For example:
def rectangle_info(width, height):
area = width * height
perimeter = 2 * (width + height)
return area, perimeter
width = 5
height = 10
area, perimeter = rectangle_info(width, height)
print("Width:", width)
print("Height:", height)
print("Area:", area)
print("Perimeter:", perimeter)
In this example, we define a function rectangle_info
that takes in the width and height of a rectangle and returns a tuple containing the area and perimeter of the rectangle. We then call the function and use tuple assignment to assign the area and perimeter values to the variables area
and perimeter
.
Examples on Swapping two numbers / tuples:
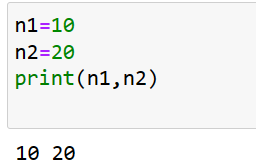
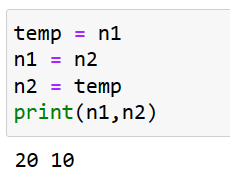
Example2 :

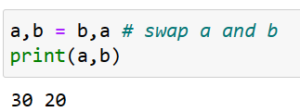
Example 3:
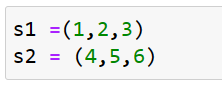
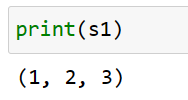
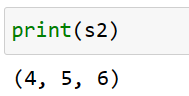
The left side is a tuple of variables, while the right side is a tuple of expressions. Each value is assigned to its respective variable. All the expressions on the right side are evaluated before any of the assignments. The number of variables on the left and the number of values on the right have to be the same:
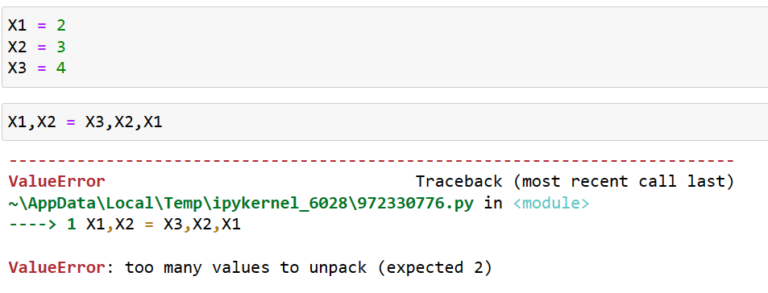
Tuple Slicing
Slice operator works on Tuple also. This is used to display more than one selected value on the output screen. Slices are treated as boundaries and the result will contain all the elements between boundaries. Tuple slicing is a way to extract a subset of elements from a tuple. Tuple slicing is done using the slicing operator :
. The syntax for tuple slicing is as follows:
tuple[start:stop:step]
where start is the index of the first element to be included in the slice, stop is the index of the first element to be excluded from the slice, and step is the stride between elements. Where start, stop & step all three are optional. If we omit first index, slice starts from ‘0’. On omitting stop, slice will take it to end. Default value of step is 1.
Here are some examples of tuple slicing in Python:
# create a tuple
my_tuple = (1, 2, 3, 4, 5)
# get a slice of the first three elements
first_three = my_tuple[0:3]
print(first_three) # Output: (1, 2, 3)
# get a slice of the last three elements
last_three = my_tuple[-3:]
print(last_three) # Output: (3, 4, 5)
# get a slice of every other element
every_other = my_tuple[::2]
print(every_other) # Output: (1, 3, 5)
# reverse the tuple
reverse_tuple = my_tuple[::-1]
print(reverse_tuple) # Output: (5, 4, 3, 2, 1)
In the first example, we get a slice of the first three elements of the tuple my_tuple
using the start index 0
and the stop index 3
. In the second example, we get a slice of the last three elements of the tuple my_tuple
using the negative start index -3
. In the third example, we get a slice of every other element of the tuple my_tuple
using a step size of 2
. In the fourth example, we reverse the order of the tuple my_tuple
using a negative step size.
Example :
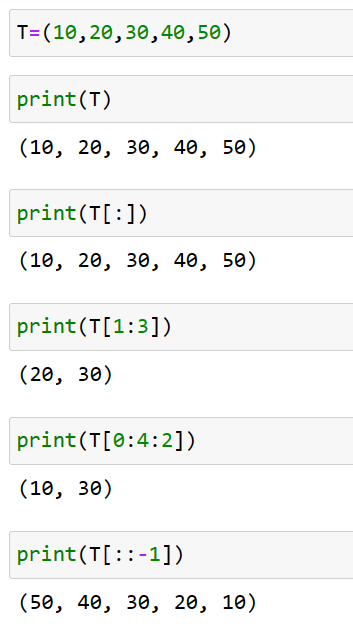
Comparing Tuples
In Python, tuples are compared element-wise, starting from the first element. The comparison stops as soon as a mismatch is found between two elements or one of the tuples has been fully compared. If all the elements in the tuples are equal, then the tuples are considered equal.
The comparison of tuples is done based on the following rules:
- If both tuples have the same length, then the comparison is done element-wise until a mismatch is found or all elements have been compared.
- If the first mismatched element in the tuples is a numeric type (integer, float, complex), then the comparison is done based on the numeric value.
- If the first mismatched element in the tuples is a string, then the comparison is done based on the ASCII value of the characters.
- If the first mismatched element in the tuples is a tuple, then the comparison is done recursively.
- If all elements in the tuples have been compared and are equal, then the tuples are considered equal.
Here are some examples of comparing tuples in Python( Tuples can be compared using comparison operators like <, >, and == as illustrated below) :
# comparing tuples with numeric values
tuple1 = (1, 2, 3)
tuple2 = (1, 2, 4)
print(tuple1 < tuple2) # Output: True
# comparing tuples with string values
tuple3 = ('apple', 'banana')
tuple4 = ('apple', 'cherry')
print(tuple3 < tuple4) # Output: True
# comparing tuples with nested tuples
tuple5 = (1, 2, (3, 4))
tuple6 = (1, 2, (3, 5))
print(tuple5 < tuple6) # Output: True
In the first example, the tuples tuple1
and tuple2
are compared element-wise, and since the third element in tuple1
is less than the third element in tuple2
, the result is True
.
In the second example, the tuples tuple3
and tuple4
are compared element-wise, and since the second element in tuple3
is less than the second element in tuple4
based on the ASCII value, the result is True
.
In the third example, the tuples tuple5
and tuple6
are compared element-wise, and since the third element in tuple5
is less than the third element in tuple6
, the result is True
.
Example :
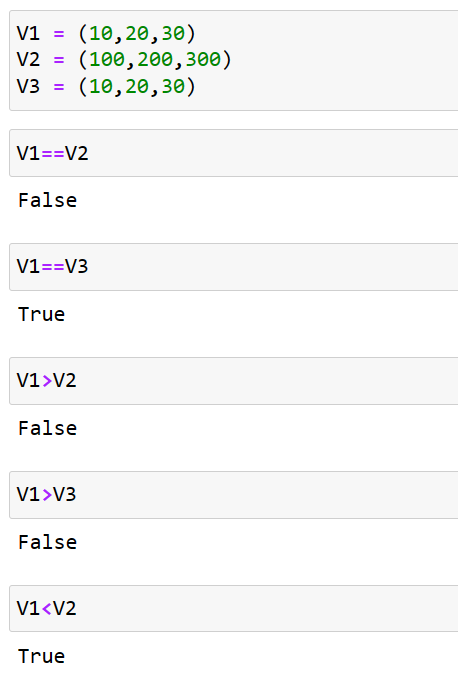
max( ) and min() functions
In Python, the max()
and min()
functions can be used to find the maximum and minimum values in a tuple. The max()
function returns the largest element in a tuple, while the min()
function returns the smallest element in a tuple. Both functions can be used with tuples containing numeric values or with tuples containing strings. However, if a tuple contains a mix of numeric and string values, the max()
and min()
functions will raise a TypeError
exception. Here are some examples of using the max()
and min()
functions with tuples:
# using max() and min() with tuples containing numeric values
tuple1 = (1, 5, 3, 7, 2, 8, 4)
print(max(tuple1)) # Output: 8
print(min(tuple1)) # Output: 1
# using max() and min() with tuples containing strings
tuple2 = ('apple', 'banana', 'cherry', 'date')
print(max(tuple2)) # Output: 'date'
print(min(tuple2)) # Output: 'apple'
In the first example, the max()
function returns the largest element in tuple1
, which is 8
. The min()
function returns the smallest element in tuple1
, which is 1
.
In the second example, the max()
function returns the element with the largest ASCII value in tuple2
, which is 'date'
. The min()
function returns the element with the smallest ASCII value in tuple2
, which is 'apple'
.
Note that the max()
and min()
functions work with any iterable object in Python, not just tuples.
Questions for Practice:
- Define Tuple , List , Strings and Dictionary in Python.
- Compare tuples with Lists. Explain how tuple can be converted into list and list into tuples.
- Write a program to input 5 subject names and put it in tuple and display that tuple information on the output screen.
- Write a program to input any two tuples and interchange the tuple values.
- Write a program to input ‘n’ numbers and store it in a tuple and find maximum & minimum values in the tuple.
- Find the output from the following code:
- T=(10,30,2,50,5,6,100,65)
- print max(T)
- print min(T)
- Find the output from the following code:
- t=tuple()
- t = t +(PYTHON,)
- print t
- print len(t)
- t1=(10,20,30)
- print len(t1)
- Write a program to input two set values and store it in tuples and also do the comparison.
- Write a program to input ‘n’ employees’ salary and find minimum & maximum salary among ‘n’ employees.
- Write a program to input ‘n’ customers’ name and store it in tuple and display all customers’ names on the output screen
- What is a tuple in Python?
- How do you create a tuple in Python?
- Can you change the elements in a tuple after it has been created?
- How do you access the elements in a tuple?
- How do you find the length of a tuple?
- Can a tuple contain duplicate elements?
- How do you concatenate two tuples?
- How do you create a tuple with a single element?
- How do you create an empty tuple?
- What is tuple packing and unpacking?
- How do you swap two values using tuple packing and unpacking?
- How do you iterate over the elements in a tuple?
- How do you convert a tuple into a list?
- How do you convert a list into a tuple?
- What is the difference between a tuple and a list?
- Can a tuple be a dictionary key in Python?
- How do you count the number of occurrences of an element in a tuple?
- How do you check if an element is in a tuple?
- How do you find the index of an element in a tuple?
- How do you slice a tuple?
- How do you compare two tuples?
- How do you find the maximum and minimum values in a tuple?
- How do you delete a tuple in Python?
- How do you copy a tuple in Python?
- How do you sort a tuple in Python?
Programming Questions on Tuples
- Write a Python program to create a tuple with numbers from 1 to 5 and print it.
- Write a Python program to create a tuple with the names of the months and print it.
- Write a Python program to create a tuple with the first five odd numbers and print it.
- Write a Python program to create a tuple with the first five even numbers and print it.
- Write a Python program to create a tuple with the first five prime numbers and print it.
- Write a Python program to create a tuple with the first five Fibonacci numbers and print it.
- Write a Python program to concatenate two tuples and print the result.
- Write a Python program to convert a tuple into a list.
- Write a Python program to convert a list into a tuple.
- Write a Python program to check if an element exists in a tuple.
- Write a Python program to count the number of occurrences of an element in a tuple.
- Write a Python program to find the index of an element in a tuple.
- Write a Python program to slice a tuple and print the result.
- Write a Python program to find the largest and smallest elements in a tuple.
- Write a Python program to create a tuple with five random numbers and print it.
- Write a Python program to create a tuple with five random words and print it.
- Write a Python program to create a tuple with the first ten positive integers and print the sum of its elements.
- Write a Python program to create a tuple with the first ten positive integers and print the product of its elements.
- Write a Python program to create a tuple with the first ten positive integers and print the average of its elements.
- Write a Python program to create a tuple with the first ten positive integers and print the median of its elements.
- Write a Python program to create a tuple with the first ten positive integers and print the mode of its elements.
- Write a Python program to create a tuple with the first ten positive integers and print the range of its elements.
- Write a Python program to create a tuple with the first ten positive integers and print the variance of its elements.
- Write a Python program to create a tuple with the first ten positive integers and print the standard deviation of its elements.
- Write a Python program to sort a tuple in descending order and print the result.
Multiple Choice Questions
- What is a tuple in Python?
- A) A sequence of comma-separated values
- B) A sequence of values enclosed in parentheses
- C) A sequence of values enclosed in square brackets
- D) A sequence of key-value pairs
Answer: B
- Can a tuple contain duplicate elements? A) Yes B) No
Answer: A
- How do you access the elements in a tuple?
- A) By index
- B) By key
- C) By value
- D) By attribute
Answer: A
- How do you find the length of a tuple?
- A) Using the len() function
- B) Using the length() function
- C) Using the count() function
- D) Using the size() function
Answer: A
- How do you create an empty tuple?
- A) Using the tuple() function
- B) Using the empty() function
- C) Using the null() function
- D) Using the None keyword
Answer: A
- What is tuple packing and unpacking?
- A) A way of combining and splitting tuples
- B) A way of converting tuples to lists
- C) A way of finding the length of a tuple
- D) A way of sorting a tuple
Answer: A
- How do you iterate over the elements in a tuple?
- A) Using a for loop
- B) Using a while loop
- C) Using the range() function D) Using the map() function
Answer: A
- How do you convert a tuple into a list?
- A) Using the list() function
- B) Using the tuple() function
- C) Using the convert() function
- D) Using the to_list() function
Answer: A
- What is the difference between a tuple and a list?
- A) Tuples are mutable and lists are immutable
- B) Tuples are ordered and lists are unordered
- C) Tuples can contain elements of different types and lists cannot
- D) Tuples can be used as dictionary keys and lists cannot
Answer: B
- How do you count the number of occurrences of an element in a tuple?
- A) Using the count() function
- B) Using the find() function
- C) Using the index() function
- D) Using the size() function
Answer: A
- How do you find the index of an element in a tuple?
- A) Using the index() function
- B) Using the count() function
- C) Using the find() function
- D) Using the search() function
Answer: A
- How do you slice a tuple?
- A) Using square brackets
- B) Using parentheses
- C) Using curly braces
- D) Using angle brackets
Answer: A
- How do you compare two tuples?
- A) Using the == operator
- B) Using the != operator
- C) Using the > and < operators
- D) All of the above
Answer: D
- How do you find the maximum value in a tuple?
- A) Using the max() function
- B) Using the min() function
- C) Using the maximum() function
- D) Using the find_max() function
Answer: A
- How do you find the minimum value in a tuple?
- A) Using the min() function
- B) Using the max() function
- C) Using the minimum() function
- D) Using the find_min() function
Answer: A
- How do you delete a tuple in Python?
- A) Using the del keyword
- B) Using the remove() function
- C) Using the discard() function
- D) Using the clear() function
Answer: A
- Which of the following is a valid way to access an element in a tuple?
- A) t{0}
- B) t(0)
- C)
- t[0]
- D) t<0>
Answer: C
- Can you add or remove elements from a tuple?
- A) Yes, you can add or remove elements from a tuple
- B) No, you cannot add or remove elements from a tuple
Answer: B
- How do you create a tuple with a single element?
- A) (1)
- B) (1,)
- C) (1,)
- D) [1]
Answer: B
- How do you create a tuple from a string?
- A) Using the str() function
- B) Using the list() function
- C) Using the tuple() function
- D) Using the string() function
Answer: C
- How do you create a tuple from a list?
- A) Using the str() function
- B) Using the list() function
- C) Using the tuple() function
- D) Using the string() function
Answer: C
- How do you reverse the order of a tuple?
- A) Using the reverse() function
- B) Using the reversed() function
- C) Using the flip() function
- D) Using the flipud() function
Answer: B
- How do you sort a tuple?
- A) Using the sort() function
- B) Using the sorted() function
- C) Tuples cannot be sorted
- D) Using the order() function
Answer: C
24. What is the output of the following code?
t = (1, 2, 3)
t[1] = 4
print(t)
A) (1, 2, 3) B) (1, 4, 3) C) (1, 2, 4) D) TypeError: ‘tuple’ object does not support item assignment
Answer: D
- What is the output of the following code?
t = (1, 2, 3, 4)
print(t[-2])
A) 1 B) 2 C) 3 D) 4
Answer: C