In Python, a string is a sequence of characters enclosed within either single quotes (‘ ‘) or double quotes (” “). Strings are one of the basic data types in Python, and they are used to store and manipulate text-based data.
Example : ‘Hello World!’ , “Python Programming“ , ”’apple”’
Creating String
In Python, you can create a string by enclosing a sequence of characters within either single quotes (‘ ‘) or double quotes (” “). Here are some examples:
my_string_1 = 'This is a string created with single quotes'
my_string_2 = "This is a string created with double quotes"
In these examples, my_string_1
and my_string_2
are both strings that contain the text “This is a string created with single quotes” and “This is a string created with double quotes”, respectively.
You can also create strings that contain special characters such as newlines (\n) and tabs (\t):
my_string_3 = "This string\nhas a newline"
my_string_4 = "This string\t has a tab"
In these examples, my_string_3
contains a newline character, which causes the text “has a newline” to appear on a new line, and my_string_4
contains a tab character, which causes a tab space before the text “has a tab”.
Example :
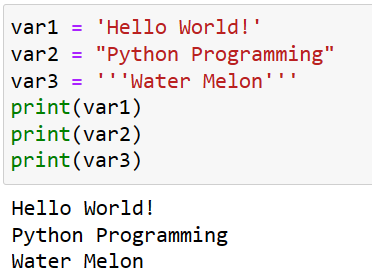
Triple quotes can extend multiple lines as illustrated below :
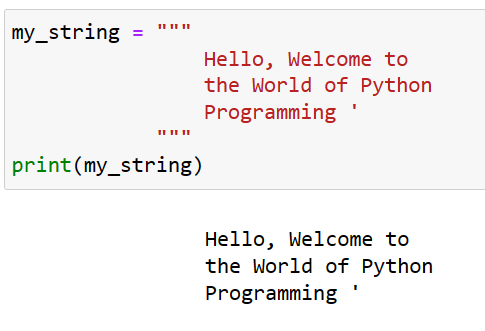
Length of a string
In Python, you can use the built-in len()
function to get the length of a string, which returns the number of characters in the string. Here’s an example:
my_string = "Hello, World!"
print(len(my_string)) # Output: 13
In this example, the len()
function is called on the my_string
string, which returns the number of characters in the string (which is 13 in this case).
Note that the len()
function counts all characters in the string, including spaces and special characters. For example:
my_string = "This string\nhas a newline"
print(len(my_string)) # Output: 22
In this example, the my_string
string contains a newline character (\n), which is counted as a single character by the len()
function.
You can use the length of a string in various ways in your code, such as checking if a string is empty, looping through the characters in a string, or performing string slicing operations.
You can find the length of a string in Python without using the built-in len()
function by iterating through the characters in the string using a loop and counting them. Here’s an example:
my_string = "Hello, World!"
count = 0
for char in my_string:
count += 1
print(count) # Output: 13
In this example, a variable count
is initialized to 0, and then a for
loop is used to iterate through each character in the my_string
string. For each character, the count
variable is incremented by 1. After the loop has finished, the count
variable contains the length of the string (which is 13 in this case).
Note that this method is not as efficient as using the built-in len()
function, especially for longer strings, because it involves iterating through each character in the string. However, it can be a useful exercise in understanding how strings are represented and manipulated in Python.
One can get last letter of string using len( ) function as illustrated below :
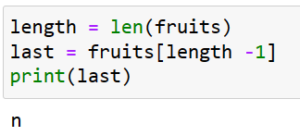
To get the last letter of a string in Python, you can use string indexing with a negative index of -1, which refers to the last character in the string. Here’s an example:
my_string = "Hello, World!"
last_letter = my_string[-1]
print(last_letter) # Output: !
In this example, the my_string
string is indexed using a negative index of -1, which refers to the last character in the string (which is the exclamation mark in this case). The last_letter
variable is then assigned this character using string indexing.
Note that this method assumes that the string is not empty. If the string is empty, attempting to index it with -1 will result in an IndexError
. To avoid this, you should first check if the string is non-empty before indexing it.
String Indexing
In Python, you can use indexing to access individual characters in a string. String indexing is done by specifying the position of the character within square brackets ([]
) immediately following the string. There are two types of indexing in Python: positive indexing and negative indexing.
my_string = "Hello, World!"
print(my_string[0]) # Output: H
print(my_string[1]) # Output: e
print(my_string[6]) # Output: ,
In this example, the my_string
string is indexed using positive indices. The first print
statement outputs the first character of the string (which is “H”), the second print
statement outputs the second character of the string (which is “e”), and the third print
statement outputs the seventh character of the string (which is “,”).
Negative indexing, on the other hand, starts from the rightmost character of the string, with the index of the last character being -1, the second-to-last character being -2, and so on. For example:
my_string = "Hello, World!"
print(my_string[-1]) # Output: !
print(my_string[-2]) # Output: d
print(my_string[-7]) # Output:
In this example, the my_string
string is indexed using negative indices. The first print
statement outputs the last character of the string (which is “!”), the second print
statement outputs the second-to-last character of the string (which is “d”), and the third print
statement outputs the seventh-to-last character of the string (which is a space).
In Python string can be indexed in forward( start to end) and backward(end to start) direction as illustrated in the figure below :
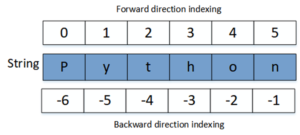
Consider a string name = ” Sophia“. Here string Sophia is made up of 6 characters. Each character of the string can be accessed by using either forward indexing or backward indexing as illustrated below:
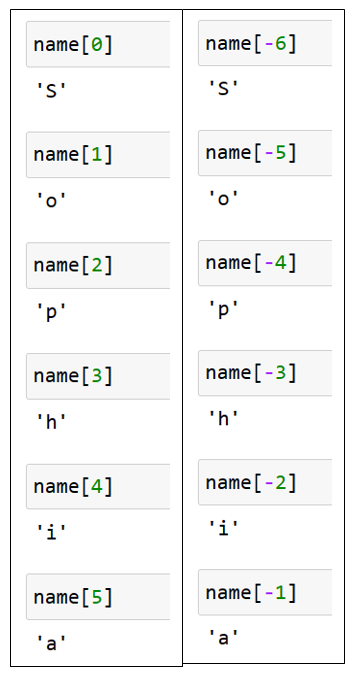
String Traversal
String traversal in Python involves iterating over each character of a string. This can be done in both forward and reverse order using loops.Forward traversal using a while loop:
my_string = "hello world"
i = 0
while i < len(my_string):
print(my_string[i])
i += 1
# Output:
h
e
l
l
o
w
o
r
l
d
In this example, we initialize the index i
to 0 and use a while loop to iterate through each character of the string. The loop continues as long as i
is less than the length of the string. At each iteration, we print the character at the current index i
and then increment i
by 1 to move to the next character.
Forward traversal using a for loop:
my_string = "hello world"
for char in my_string:
print(char)
# output
h
e
l
l
o
w
o
r
l
d
In this example, we use a for loop to iterate through each character of the string. The loop variable char
takes on the value of each character in the string in turn, allowing us to access and print each character.
Reverse traversal using a while loop:
my_string = "hello world"
i = len(my_string) - 1
while i >= 0:
print(my_string[i])
i -= 1
#Output:
d
l
r
o
w
o
l
l
e
h
In this example, we initialize the index i
to the last index of the string (which is the length of the string minus one) and use a while loop to iterate through each character of the string in reverse order. The loop continues as long as i
is greater than or equal to 0. At each iteration, we print the character at the current index i
and then decrement i
by 1 to move to the previous character.
Reverse traversal using a for loop:
my_string = "hello world"
for char in reversed(my_string):
print(char)
#output
d
l
r
o
w
o
l
l
e
h
In this example, we use the built-in reversed()
function to reverse the order of the characters in the string, and then use a for loop to iterate through each character in the reversed order. The loop variable char
takes on the value of each character in the string in turn, allowing us to access and print each character.
Lists aren’t the only data types that represent ordered sequences of values.For example, strings and lists are actually similar, if you consider a string to be a “list” of single text characters. Many of the things you can do with lists can also be done with strings: indexing; slicing; and using them with for loops, with len(), and with the in and not in operators.
String Slicing
String slicing is a technique in Python that allows you to extract a portion of a string by specifying its start and end positions. This is done using the slice operator :
. The basic syntax for string slicing is as follows:
string[start:end:step]
start
is the index of the first character to include in the slice (inclusive)end
is the index of the last character to include in the slice (exclusive)step
is the number of characters to skip between each included character (optional)
Here are some examples of string slicing:
my_string = "Hello, World!"
# Extract the first 5 characters
print(my_string[0:5]) # Output: "Hello"
# Extract the characters from index 7 to the end
print(my_string[7:]) # Output: "World!"
# Extract the last 6 characters
print(my_string[-6:]) # Output: "World!"
# Extract every other character
print(my_string[::2]) # Output: "Hlo ol!"
# Reverse the string
print(my_string[::-1]) # Output: "!dlroW ,olleH"
In the first example, we use the slice operator 0:5
to extract the first 5 characters of the string “Hello, World!”. This includes the characters at indices 0 through 4 (inclusive).
In the second example, we use the slice operator 7:
to extract the characters from index 7 (the ‘W’ in “World!”) to the end of the string. This includes all characters starting at index 7 and continuing to the end of the string.
In the third example, we use negative indexing and the slice operator -6:
to extract the last 6 characters of the string “Hello, World!”. This includes all characters starting 6 positions from the end of the string and continuing to the end of the string.
In the fourth example, we use the slice operator ::2
to extract every other character of the string “Hello, World!”. This includes the first character, then skips the next character, then includes the third character, and so on.
In the fifth example, we use negative step and the slice operator [::-1]
to reverse the string “Hello, World!”. This includes all characters in the string, but they are extracted in reverse order because the step is -1.
Example :
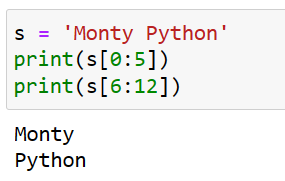
- The : operator in slicing [n:m] returns the part of the string from the “n-eth” character to the “m-eth” character, including the first but excluding the last.
- If you omit the first index (before the colon), the slice starts at the beginning of the string.
- If you omit the second index, the slice goes to the end of the string:
- fruit = ‘banana’
- fruit[:3] gives ‘ban’
- fruit[3:] gives ‘ana’
- If the first index is greater than or equal to the second the result is an empty string, represented by two quotation marks:
- fruit = ‘banana’
- fruit[3:3] gives ‘ ‘
- An empty string contains no characters and has length 0, but other than that, it is the same as any other string.
Example :

Strings are immutable
In Python, strings are immutable objects, which means that once a string is created, it cannot be modified. Immutable objects are objects whose state cannot be changed once they are created.
When we modify a string in Python, what actually happens is that a new string is created with the desired modifications, while the original string remains unchanged. This is different from mutable objects like lists, where you can modify their elements in place.
Here is an example to demonstrate string immutability:
my_string = "hello"
my_string[0] = "H" # This will cause an error
In this example, we try to modify the first character of the string “hello” to be uppercase. However, this will result in a TypeError, because strings are immutable and cannot be modified in place.
Instead, we need to create a new string with the desired modifications. Here is an example:
my_string = "hello"
new_string = "H" + my_string[1:]
print(new_string) # Output: "Hello"
In this example, we create a new string by concatenating the uppercase letter “H” with a slice of the original string that starts at index 1 and goes to the end. This creates a new string “Hello” that has the desired modification.
Example : The proper way to ‘mutate‘ a string is to slice and concatenate to build a new string by copying from parts of the old string as illustrated below :
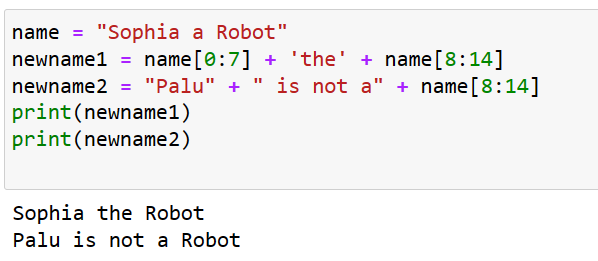
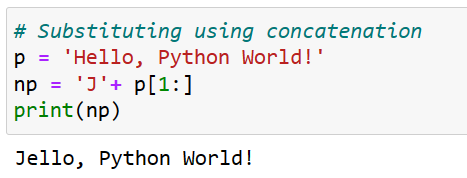
Looping and Counting
Looping over a string: To loop over a string in Python, you can use a for
loop. In each iteration of the loop, the loop variable will take on the value of the next character in the string. Here is an example:
my_string = "hello"
for char in my_string:
print(char)
# Output:
h
e
l
l
o
Counting occurrences of a character in a string: To count the number of occurrences of a specific character in a string, you can use the count()
method of the string. This method takes a single argument, which is the character to count. Here is an example:
my_string = "hello"
count = my_string.count('l')
print(count) # Output: 2
Counting occurrences of a substring in a string:
To count the number of occurrences of a substring in a string, you can use a loop and the count()
method. Here is an example:
my_string = "hello world"
substring = "l"
count = 0
for char in my_string:
if char == substring:
count += 1
print(count) # Output: 3
In this example, we loop over each character in the string and increment a counter if the character matches the substring we are searching for.
Example :
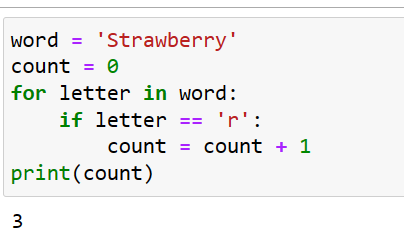
Example : usage of in operator for strings
The in
operator is a built-in operator in Python that is used to check whether a given value is present in a sequence (such as a string, list, or tuple). When used with a string, the in
operator checks whether a substring is present in the string. Here are some examples:
# Check if a substring is present in a string
my_string = "hello world"
substring = "world"
if substring in my_string:
print("Substring found")
else:
print("Substring not found")
# Check if a character is present in a string
my_string = "hello"
char = "l"
if char in my_string:
print("Character found")
else:
print("Character not found")
#Output
Substring found
Character found
In the first example, we use the in
operator to check whether the substring “world” is present in the string “hello world”. Since it is present, the output is “Substring found”.
In the second example, we use the in
operator to check whether the character “l” is present in the string “hello”. Since it is present twice, the output is “Character found”.
Note that the in
operator is case-sensitive. For example, if you search for the substring “World” in the string “hello world”, it will not be found because the capitalization does not match.
Example :
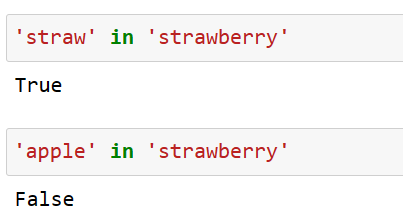
String Comparison using ==,< and > operators
In Python, you can compare strings using the ==
, <
, and >
operators, just like you can with other data types such as numbers. Here’s how each operator works for string comparison:
==
(equal to) operator: The ==
operator checks whether two strings have the same content. It returns True
if the strings are equal and False
otherwise. Here’s an example:
string1 = "hello"
string2 = "hello"
if string1 == string2:
print("The strings are equal")
else:
print("The strings are not equal")
#Output: The strings are equal
<
(less than) and >
(greater than) operators:
The <
and >
operators compare two strings lexicographically (i.e., in dictionary order). They return True
if the first string is less than or greater than the second string, respectively, and False
otherwise. Here’s an example:
string1 = "apple"
string2 = "banana"
if string1 < string2:
print("string1 comes before string2 in dictionary order")
else:
print("string2 comes before string1 in dictionary order")
#Output: string1 comes before string2 in dictionary order
Note that the comparison of strings is case-sensitive, meaning that uppercase letters come before lowercase letters in the ASCII table. Also, the <=
and >=
operators can also be used for string comparison, which check whether the first string is less than or equal to, or greater than or equal to the second string, respectively.
Useful String methods
Strings are an example of Python objects. An object contains both data ( the actual string itself ) and methods which are effectively functions that are built into the object and are available to any instance of the object. Using the command dir(str) one can list all the methods supported by python as illustrated below:
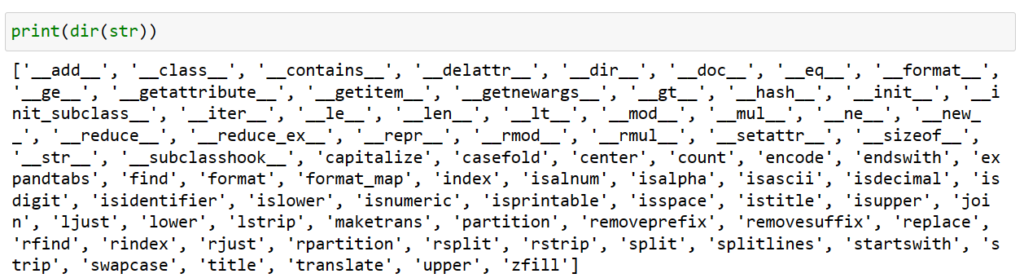
Table : String Methods and Description
Method | Description |
---|---|
capitalize() | Converts the first character to upper case |
casefold() | Converts string into lower case |
center() | Returns a centered string |
count() | Returns the number of times a specified value occurs in a string |
encode() | Returns an encoded version of the string |
endswith() | Returns true if the string ends with the specified value |
find() | Searches the string for a specified value and returns the position of where it was found |
index() | Searches the string for a specified value and returns the position of where it was found |
isalnum() | Returns True if all characters in the string are alphanumeric |
isalpha() | Returns True if all characters in the string are in the alphabet |
isdigit() | Returns True if all characters in the string are digits |
isidentifier() | Returns True if the string is an identifier |
islower() | Returns True if all characters in the string are lower case |
isnumeric() | Returns True if all characters in the string are numeric |
isspace() | Returns True if all characters in the string are whitespaces |
istitle() | Returns True if the string follows the rules of a title |
isupper() | Returns True if all characters in the string are upper case |
ljust() | Returns a left justified version of the string |
lower() | Converts a string into lower case |
lstrip() | Returns a left trim version of the string |
replace() | Returns a string where a specified value is replaced with a specified value |
rjust() | Returns a right justified version of the string |
rsplit() | Splits the string at the specified separator, and returns a list |
rstrip() | Returns a right trim version of the string |
split() | Splits the string at the specified separator, and returns a list |
startswith() | Returns true if the string starts with the specified value |
strip() | Returns a trimmed version of the string |
swapcase() | Swaps cases, lower case becomes upper case and vice versa |
title() | Converts the first character of each word to upper case |
upper() | Converts a string into upper case |
[Source : https://www.w3schools.com/python/python_ref_string.asp]
capitalize() : Converts the first character to upper case
The capitalize()
method is a built-in method in Python that converts the first character of a string to uppercase and leaves the rest of the string unchanged. If the first character of the string is already in uppercase, then the method has no effect on that character.
Here’s an example:
my_string = "hello world"
capitalized = my_string.capitalize()
print(capitalized) # Output: "Hello world"
In this example, the capitalize()
method is used to convert the first character of the string "hello world"
to uppercase, resulting in the string "Hello world"
.
Note that capitalize()
does not modify the original string, but returns a new string with the first character capitalized. Also, if the original string is empty, the capitalize()
method will return an empty string.
lower()
The lower()
method is a built-in string method in Python that returns a new string with all the alphabetic characters in the original string converted to lowercase.
Here’s an example:
my_string = "Hello, World!"
lowercase_string = my_string.lower()
print(lowercase_string)
# Output: hello, world!
In this example, the lower()
method is called on the my_string
variable and the returned value is assigned to the lowercase_string
variable. The original string my_string
is not modified, instead a new string is created with all alphabetic characters in lowercase.
Note that lower()
method only works with alphabetic characters, and any non-alphabetic characters (such as punctuation, numbers, and spaces) are not affected by the method.
Example :
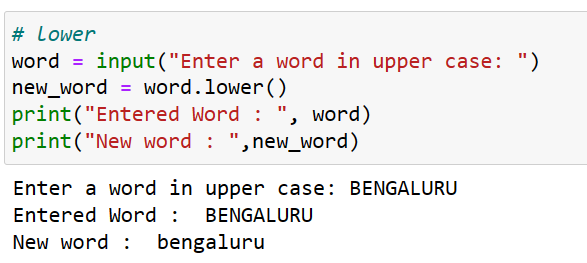
upper()
The upper()
method is a built-in string method in Python that is used to convert all the lowercase characters in a string to uppercase characters. The method takes no arguments and returns a new string with all the characters in uppercase.
Here’s an example:
text = "hello, world!"
uppercase_text = text.upper()
print(uppercase_text)
# Output : HELLO, WORLD!
In the above example, the upper()
method is used to convert all the lowercase characters in the string text
to uppercase characters. The resulting string is then stored in the variable uppercase_text
and printed to the console.
It’s important to note that the upper()
method does not modify the original string, but instead returns a new string with all the characters in uppercase. If you want to modify the original string, you can assign the result of the upper()
method back to the original variable:
text = "hello, world!"
text = text.upper()
print(text)
# Output : HELLO, WORLD!
Example :
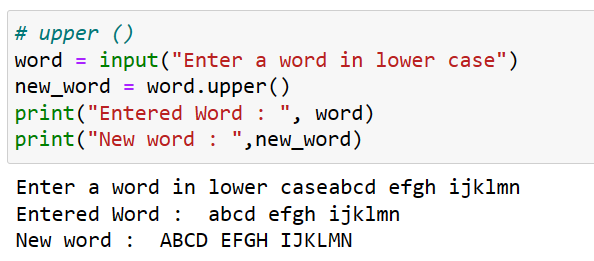
casefold(): Converts string into lower case
Actually, the casefold()
method in Python is used to convert a string into a lowercase string, just like the lower()
method. However, the casefold()
method is more aggressive in its conversion and is often preferred over the lower()
method when dealing with case-insensitive string comparisons.
The casefold()
method removes all case distinctions from a string and converts it into a form that is suitable for case-insensitive comparisons. This method also handles some special cases, such as the German “sharp s” character (ß), which is converted to “ss” when casefolded.
Here’s an example:
text = "Hello, World!"
lowercase_text = text.casefold()
print(lowercase_text)
#output
hello, world!
In the above example, the casefold()
method is used to convert the string text
to lowercase. The resulting string is then stored in the variable lowercase_text
and printed to the console.
Just like the lower()
method, the casefold()
method does not modify the original string, but instead returns a new string with all the characters in lowercase. If you want to modify the original string, you can assign the result of the casefold()
method back to the original variable:
text = "Hello, World!"
text = text.casefold()
print(text)
# Output:
hello, world!
Example:
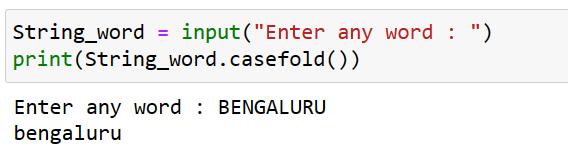
center() : Returns a centered string
The center()
method is a built-in string method in Python that returns a centered string. It takes two arguments: width
, which specifies the total width of the resulting string, and an optional fillchar
argument that specifies the character to be used for padding.
Here’s the syntax for center()
method:
string.center(width[, fillchar])
The width
argument is required, and must be a positive integer. The fillchar
argument is optional, and must be a string of length 1. If fillchar
is not specified, a space character is used for padding.
Here’s an example of how to use the center()
method:
string = "Hello"
width = 10
fillchar = "*"
centered_string = string.center(width, fillchar)
print(centered_string)
# Output:
**Hello***
In this example, the original string "Hello"
is centered in a string of width 10
with the fill character *
, resulting in the string **Hello***
.
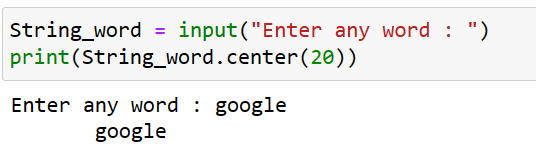
swapcase()
swapcase()
is a built-in string method in Python that returns a new string where the case of each character in the original string is swapped. In other words, all uppercase characters are converted to lowercase, and all lowercase characters are converted to uppercase.
Here’s the syntax for swapcase()
method:
string.swapcase()
Here’s an example of how to use the swapcase()
method:
string = "Hello World"
swapped_string = string.swapcase()
print(swapped_string)
#Output: hELLO wORLD
In this example, the original string "Hello World"
is swapped using the swapcase()
method, resulting in the string "hELLO wORLD"
.
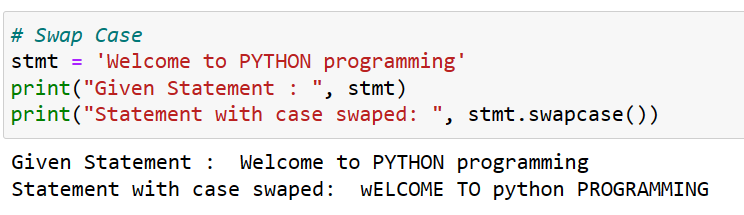
find()
find()
is a built-in string method in Python that returns the lowest index of the first occurrence of a specified substring within a string. If the substring is not found, it returns -1.
Here’s the syntax for find()
method:
string.find(substring[, start[, end]])
The substring
argument is required, and specifies the substring to be searched within the string. The start
and end
arguments are optional, and specify the starting and ending index positions for the search. If start
is not specified, the search starts from the beginning of the string. If end
is not specified, the search goes until the end of the string.
Here’s an example of how to use the find()
method:
string = "Hello World"
substring = "World"
index = string.find(substring)
print(index)
# output : 6
In this example, the find()
method is used to search for the substring "World"
within the string "Hello World"
. The method returns the lowest index of the substring, which is 6
.
If the substring is not found within the string, the find()
method returns -1:
string = "Hello World"
substring = "Universe"
index = string.find(substring)
print(index)
# Output: -1
Example :
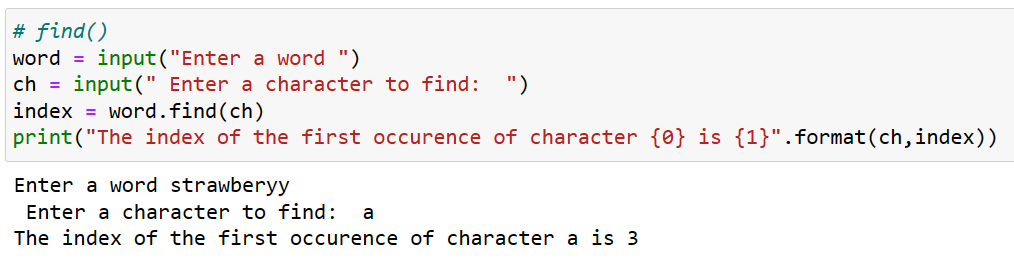
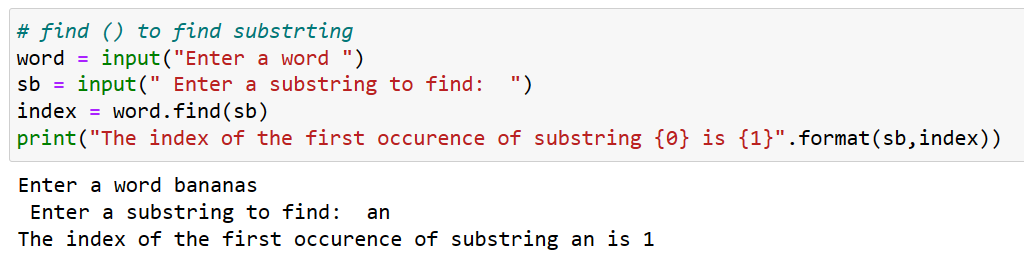
count()
count()
is a built-in string method in Python that returns the number of occurrences of a specified substring within a string.Here’s the syntax for count()
method:
string.count(substring[, start[, end]])
The substring
argument is required, and specifies the substring to be counted within the string. The start
and end
arguments are optional, and specify the starting and ending index positions for the count. If start
is not specified, the count starts from the beginning of the string. If end
is not specified, the count goes until the end of the string.Here’s an example of how to use the count()
method:
string = "Hello World"
substring = "l"
count = string.count(substring)
print(count)
# output : 3
In this example, the count()
method is used to count the number of occurrences of the substring "l"
within the string "Hello World"
. The method returns the number of occurrences, which is 3
.
You can also use the count()
method to count the occurrences of a substring within a specific range of the string:
string = "Hello World"
substring = "l"
start = 3
end = 8
count = string.count(substring, start, end)
print(count)
#Output : 1
In this example, the count()
method is used to count the number of occurrences of the substring "l"
within the range of the string "lo Wo"
, which starts from index 3
and ends at index 8
. The method returns the number of occurrences within the range, which is 1
.
Example :
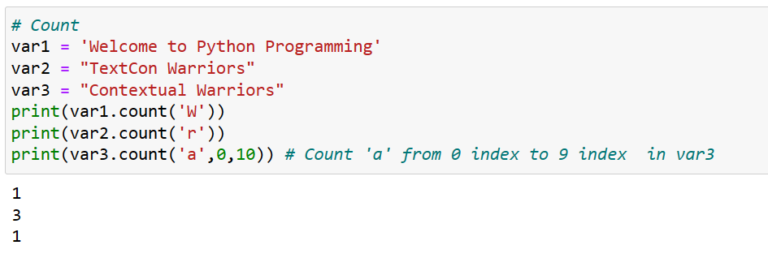
strip(), lstrip() and rstrip()
strip()
, lstrip()
, and rstrip()
are built-in string methods in Python that remove leading and/or trailing characters from a string. strip()
removes leading and trailing whitespace characters (spaces, tabs, newlines) from a string. Here’s the syntax for strip()
strip()
, lstrip()
, and rstrip()
methods:
string.strip([characters])
string.lstrip([characters])
string.rstrip([characters])
The characters
argument is optional, and specifies the characters to be removed from both ends of the string. If characters
is not specified, it removes whitespace characters by default.
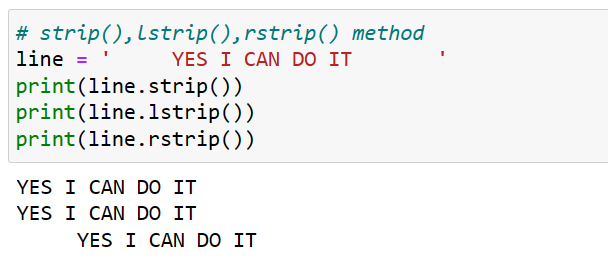
startswith() and endswith()
startswith()
and endswith()
are built-in string methods in Python that are used to check whether a string starts with or ends with a specific substring, respectively.
startswith()
returnsTrue
if the string starts with the specified substring, andFalse
otherwise.endswith()
returnsTrue
if the string ends with the specified substring, andFalse
otherwise.
Here’s the syntax for startswith()
and endswith() method:
string.startswith(substring[, start[, end]])
string.endswith(substring[, start[, end]])
Example :
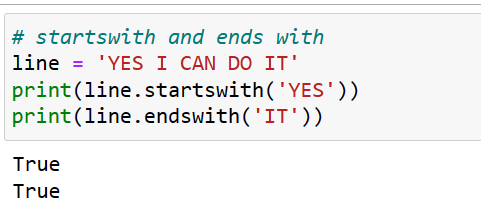
split()
split()
is a built-in string method in Python that is used to split a string into a list of substrings based on a specified separator.
Here’s the syntax for split()
method:
string.split(sep=None, maxsplit=-1)
The sep
argument is optional, and specifies the separator to use for splitting the string. If sep
is not specified, the default separator is whitespace.
The maxsplit
argument is optional, and specifies the maximum number of splits to be performed. If maxsplit
is not specified or set to -1
, all possible splits are performed.
Here’s an example of how to use the split()
method:
string = "Hello World"
words = string.split()
print(words)
# ['Hello', 'World']
Example :
string = "apple,banana,orange"
fruits = string.split(",")
print(fruits)
#output: ['apple', 'banana', 'orange']
Example:
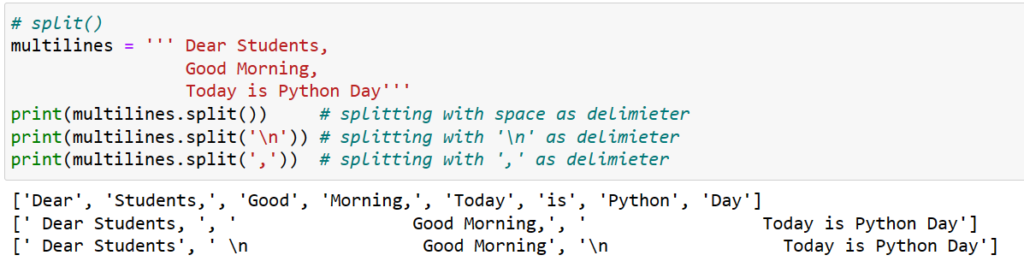
Example :
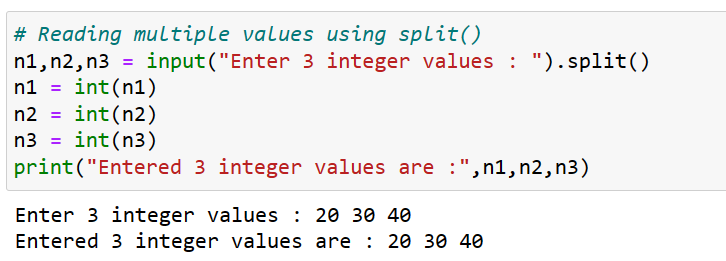
join()
join()
is a built-in method in Python that can be used to concatenate a sequence of strings into a single string. The method takes an iterable, such as a list or tuple, and concatenates each element in the iterable into a single string with a separator between them.
Here’s an example of how to use join()
words = ["hello", "world", "!"]
separator = " "
sentence = separator.join(words)
print(sentence)
#output: hello world !
In this example, we create a list of words and a separator string. We then use the join()
method to concatenate the words in the list into a single string, with the separator between each word. The resulting string is assigned to the sentence
variable and printed to the console.
Note that the join()
method can be used with any iterable that contains strings, not just lists. Additionally, the separator string can be any string value, including an empty string.
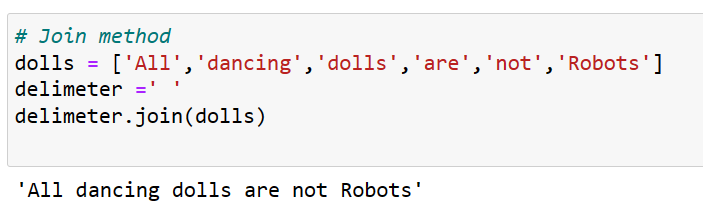
ASCII VALUES
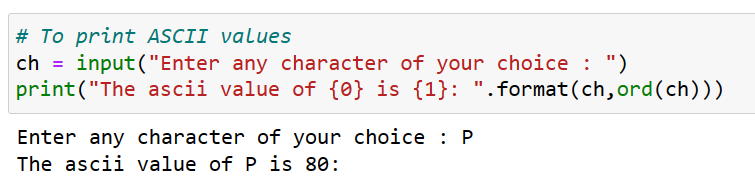
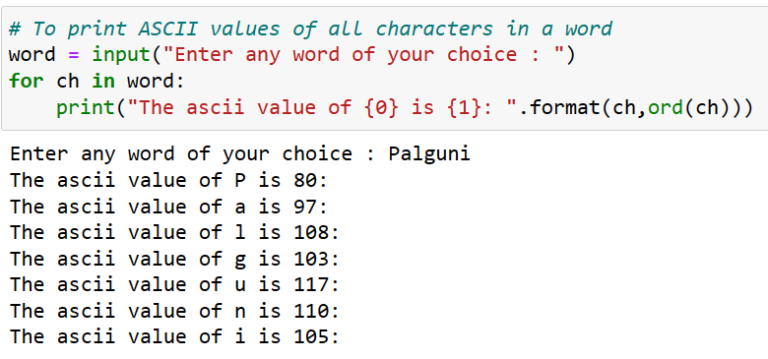
To print ASCII character for given ASCII Value:
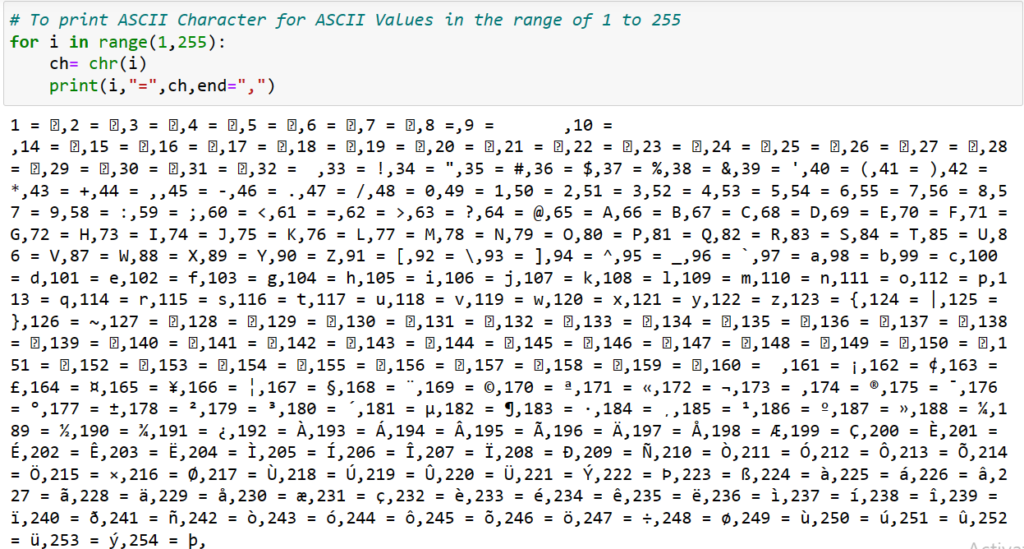
Python String Operators
Python provides several operators that can be used with strings. Here are some of the most commonly used string operators in Python:
+
(concatenation operator): This operator is used to concatenate two or more strings. For example:
string1 = "Hello"
string2 = "world"
result = string1 + " " + string2
print(result)
#Output: Hello world
2. *
(repetition operator): This operator is used to repeat a string a certain number of times. For example:
string = "spam"
result = string * 3
print(result)
# output: spamspamspam
3. in
and not in
(membership operators): These operators are used to check if a string is present in another string. For example:
string = "hello world"
print("hello" in string) # True
print("goodbye" not in string) # True
4. %
(string formatting operator): This operator is used to format a string with variables. For example:
name = "Alice"
age = 30
result = "My name is %s and I'm %d years old." % (name, age)
print(result)
# output: My name is Alice and I'm 30 years old.
5. []
(indexing operator): This operator is used to access individual characters in a string. For example:
string = "hello"
print(string[0]) # 'h'
print(string[-1]) # 'o'
6. Range slicing, denoted by [:]
, is a way to extract a substring from a string in Python. It works by specifying the start and end indices of the substring that you want to extract. For example, consider the following string:
s = "hello world"
To extract the substring "hello", you can use the range slicing syntax s[0:5]:
substring = s[0:5]
print(substring)
Output:
hello
In this example, s[0:5] specifies the range from index 0 (inclusive) to index 5 (exclusive), which corresponds to the substring "hello".
You can also omit the starting or ending index to specify a range that starts from the beginning or ends at the end of the string, respectively. For example, s[:5] extracts the substring "hello", and s[6:] extracts the substring "world".
substring1 = s[:5]
substring2 = s[6:]
print(substring1)
print(substring2)
# output :
hello
world
Range slicing can also be used with negative indices, which count from the end of the string. For example, s[-5:-1] extracts the substring "worl".
substring = s[-5:-1]
print(substring)
# output:
worl
Range slicing is a powerful feature in Python strings that allows you to extract substrings quickly and easily.
Format Operators
Format operators are used in print statement . There are two format operators :
- %
- format()
Both %
and format()
are string formatting operators in Python that allow you to insert values into a string.
% operator:
Syntax :
print("%s1 %s2 %s3 ------"%(arg1,arg2,arg3,------argn)) Here, s1,s2 ,..........sn are conversion specifiers like d(for int ),f(for float),s(for string) ,etc. and arg1,arg2....argn are variables or values. The%
operator is an older way to format strings and is based on C-style string formatting. It uses placeholders in the string, such as%s
and%d
, to indicate where variables should be inserted. Here's an example:
name = "Alice"
age = 30
result = "My name is %s and I'm %d years old." % (name, age)
print(result)
# Output:
My name is Alice and I'm 30 years old.
In this example, %s
is a placeholder for a string value and %d
is a placeholder for an integer value. The variables name
and age
are inserted into the string using the %
operator.
Example :
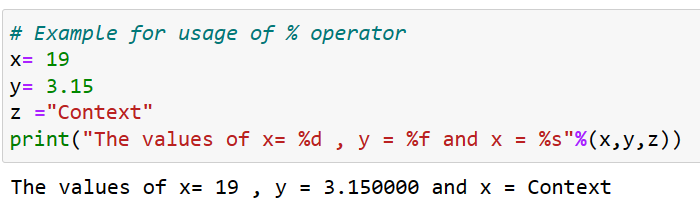
format() function
Syntax :
print('{0} {1} ......{n}'.format(agr1,agr2,agr3....argn) Here, 0,1,2,------are position specifiers and arg1,arg2,-----argn are variables/values
The format()
method is a newer way to format strings and is more versatile than the %
operator. It uses curly braces {}
to indicate where variables should be inserted. Here’s an example:
name = "Alice"
age = 30
result = "My name is {} and I'm {} years old.".format(name, age)
print(result)
#
My name is Alice and I'm 30 years old.
Example :
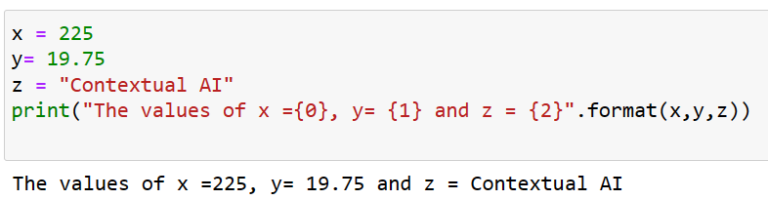
Sample Programs:
1.Write a Python Program to Check if the String is Symmetrical or Palindrome:
A string is said to be symmetrical if both halves of the string are the same, and a string is said to be a palindrome if one half of the string is the opposite of the other half or if the string appears the same whether read forward or backward.
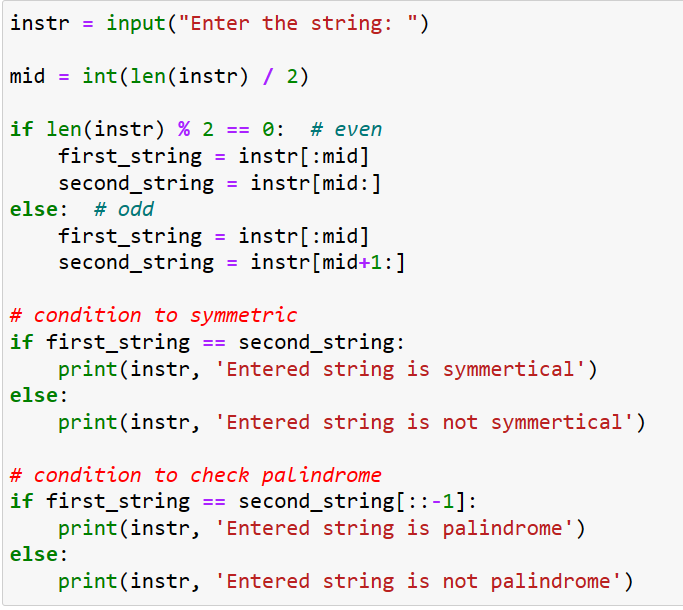
Output 1 :
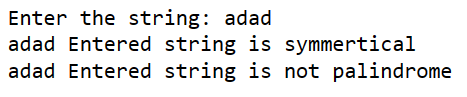
Output 2

2. Write a Python Program to find the length of string
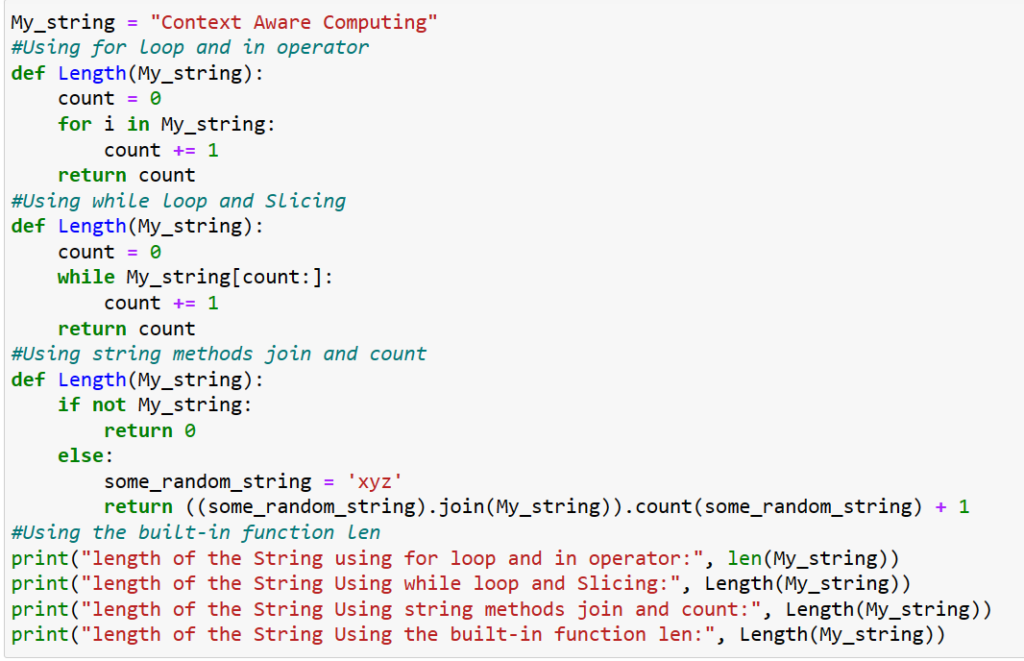
Output :

3. Write a Python Program to Count the Number of Digits, Alphabets, and Other Characters in a String
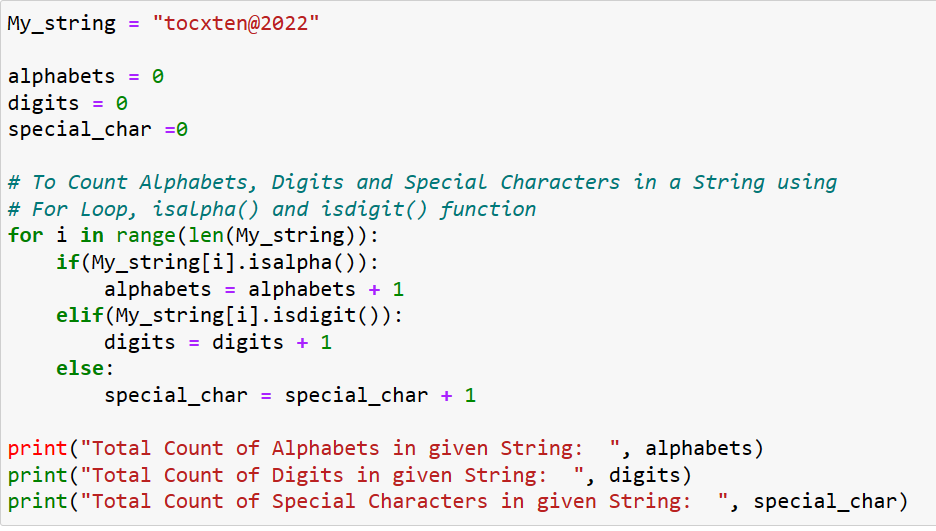
Output :

4. Write a Python Program to Count the Number of Vowels in a String
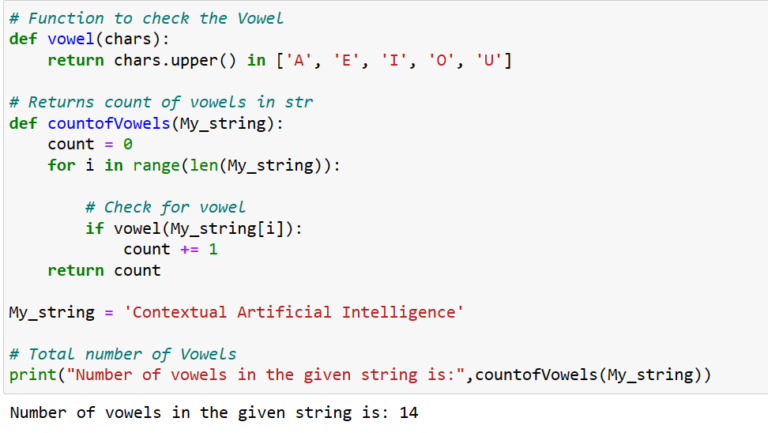
5. Write a Python Program to Split and Join a String
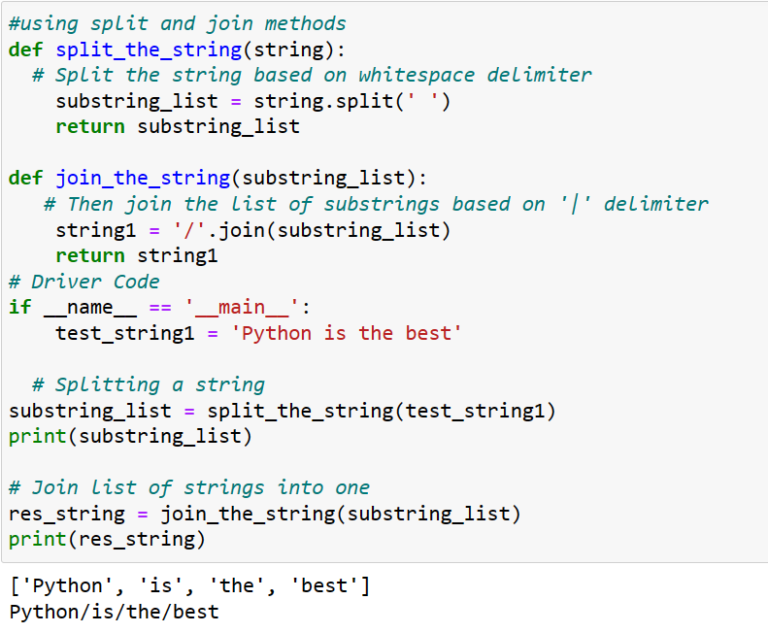
6. Write a Python program to display a largest word from a string
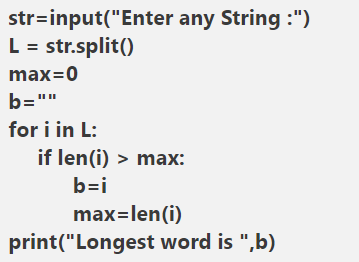
7. Write a Python program to display unique words from a string
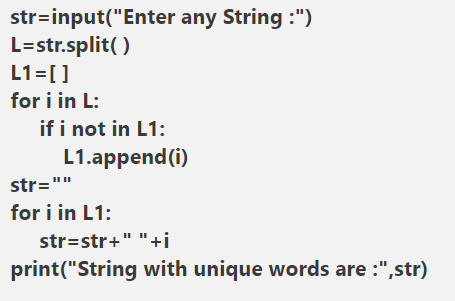
8.Write a Program to accept a word and display a ASCII value of each character of words
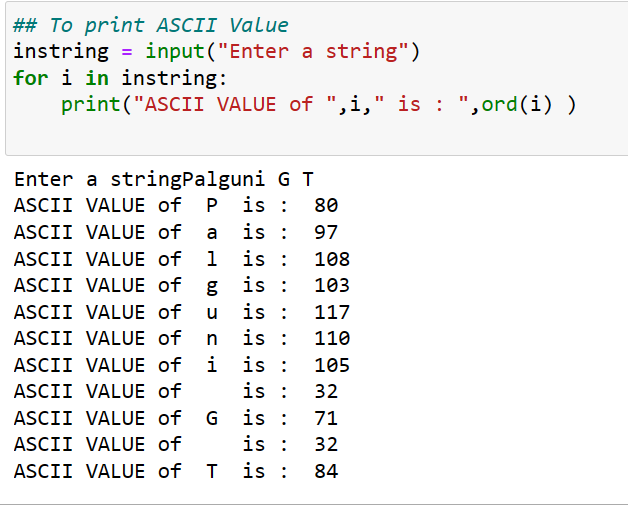
Questions for Practice:
- Define String? Explain at least 5 String functions with examples.
- Write a python program to display presence of given substring in main string.
- Define Tuple , List , Strings and Dictionary in Python.
- List any six methods associated with string and explain each of them with example.
- Write a Python program to swap cases of a given string .
- Input : Java
- Output : jAVA
- Explain the various string methods for the following operations with examples.
- Removing white space characters from the beginning end or both sides of a string
- To right -justify , left justify and center a string
- What are escape characters?
- What do the \n and \t escape characters represent?
- How can you put a \ backslash character in a string?
- The string value “Howl’s Moving Castle” is a valid string. Why isn’t it
a problem that the single quote character in the word Howl’s isn’t
escaped? - If you don’t want to put \n in your string, how can you write a string
with newlines in it? - What do the following expressions evaluate to?
• ‘Hello world!'[1]
• ‘Hello world!'[0:5]
• ‘Hello world!'[:5]
• ‘Hello world!'[3:] - What do the following expressions evaluate to?
- • ‘Hello’.upper()
- • ‘Hello’.upper().isupper()
- • ‘Hello’.upper().lower()
- What do the following expressions evaluate to?
- • ‘Remember, remember, the fifth of November.’.split()
- • ‘-‘.join(‘There can be only one.’.split())
- What string methods can you use to right-justify, left-justify, and center a string?
- How can you trim whitespace characters from the beginning or end of
a string? - Write a Python program to calculate the length of a string.
- Write a program to check if the letter ‘e’ is present in the word ‘Umbrella’.
- Write a program to check if the word ‘orange’ is present in the “This is orange juice”.
- Write a program to find the first and the last occurence of the letter ‘o’ and character ‘,’ in “Hello, World”.
- Write a program that takes your full name as input and displays the abbreviations of the first and middle names
- except the last name which is displayed as it is. For example, if your name is Robert Brett Roser, then the output should be R.B.Roser.
- Write a program to find the number of vowels, consonents, digits and white space characters in a string.
- Write a program to make a new string with all the consonents deleted from the string “Hello, have a good day”
- Write a Python program to count the number of characters (character frequency) in a string.
- Write the string after the first occurrence of ‘,’ and the string after the last occurrence of ‘,’ in the string “Hello, Good, Morning”. World”.
- Write a program to print every character of a string entered by user in a new line using loop.
- Write a program to find the length of the string “refrigerator” without using len function.
- Write a program to check if the letter ‘e’ is present in the word ‘Umbrella’.
- Write a program to check if the word ‘orange’ is present in the “This is orange juice”.
- Write a program to find the first and the last occurence of the letter ‘o’ and character ‘,’ in “Hello, World”.
- Write a program that takes your full name as input and displays the abbreviations of the first and middle names except the last name which is displayed as it is. For example, if your name is Robert Brett Roser, then the output should be R.B.Roser.
- Check the occurrence of the letter ‘e’ and the word ‘is’ in the sentence “This is umbrella”.
- Write a program to find the number of vowels, consonents, digits and white space characters in a string.
- Write a program to make a new string with all the consonents deleted from the string “Hello, have a good day”.