Project 1 : Guess the number Game
Guess the number is a simple game where the player tries to guess a random number within a certain range.
Source Code :
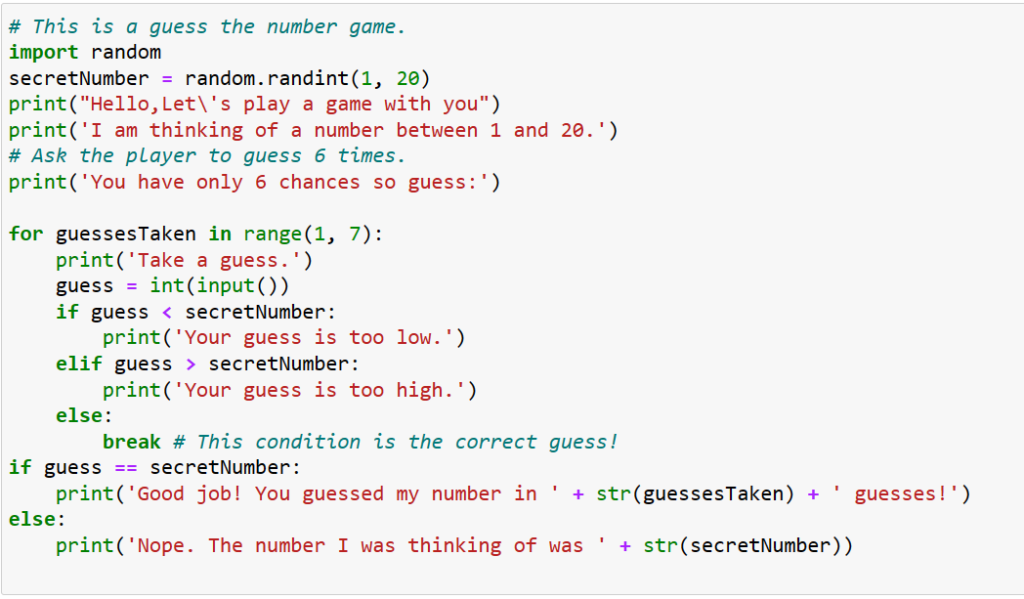
Output :
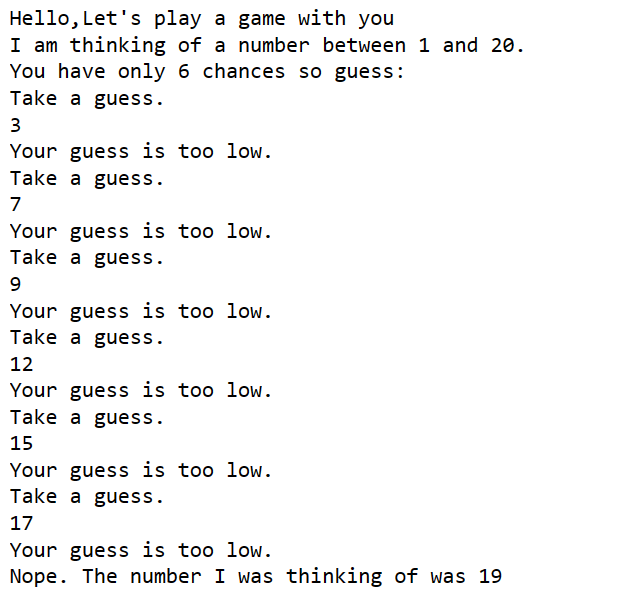
Project 2 : Password Generator
A password generator is a program that automatically creates strong, random passwords that are difficult for others to guess or crack. [ Source : https://www.freecodecamp.org/news/python-program-examples-simple-code-examples-for-beginners/amp/ ]
Source Code :
import random
import string
def generate_password(length):
"""This function generates a random password
of a given length using a combination of
uppercase letters, lowercase letters,
digits, and special characters"""
# Define a string containing all possible characters
all_chars = string.ascii_letters + string.digits + string.punctuation
# Generate a password using a random selection of characters
password = "".join(random.choice(all_chars) for i in range(length))
return password
# Test the function by generating a password of length 10
password = generate_password(10)
print(password
Sample Output:
#Output1:
q<he$,7gj/
#output2:
&X#O+*ij0_
#Output3:
nSj]>V!b59
#Output4:
9K:qE\PTU>
Project 3: Password Checker
A password checker is a program that evaluates the strength of a password based on certain criteria, such as length, complexity, and uniqueness. [ Source : https://www.freecodecamp.org/news/python-program-examples-simple-code-examples-for-beginners/amp/ ]
Source Code :
# Define a function to check if the password is strong enough
def password_checker(password):
# Define the criteria for a strong password
min_length = 8
has_uppercase = False
has_lowercase = False
has_digit = False
has_special_char = False
special_chars = "!@#$%^&*()-_=+[{]}\|;:',<.>/?"
# Check the length of the password
if len(password) < min_length:
print("Password is too short!")
return False
# Check if the password contains an uppercase letter, lowercase letter, digit, and special character
for char in password:
if char.isupper():
has_uppercase = True
elif char.islower():
has_lowercase = True
elif char.isdigit():
has_digit = True
elif char in special_chars:
has_special_char = True
# Print an error message for each missing criteria
if not has_uppercase:
print("Password must contain at least one uppercase letter!")
return False
if not has_lowercase:
print("Password must contain at least one lowercase letter!")
return False
if not has_digit:
print("Password must contain at least one digit!")
return False
if not has_special_char:
print("Password must contain at least one special character!")
return False
# If all criteria are met, print a success message
print("Password is strong!")
return True
# Prompt the user to enter a password and check if it meets the criteria
password = input("Enter a password: ")
password_checker(password)
Sample Output :
#Output1
Enter a password: $%^&2348971
Password must contain at least one uppercase letter!
False
#Ouput2
Enter a password: abc!@#767Thy
Password is strong!
True
#Ouput3
Enter a password: abcdefghi
Password must contain at least one uppercase letter!
False
Project 4: Web Scrapping Links form a given website
Web scraping is the process of extracting data from websites using automated methods. Here are the basic steps to perform web scraping using Python:
- Choose a website to scrape: Identify the website from which you want to extract data.
- Inspect the website: Use the web browser’s developer tools to inspect the website and identify the HTML elements that contain the data you want to extract.
- Install the necessary libraries: You can use Python libraries like
requests
andBeautifulSoup
to extract data from websites. Install them by runningpip install requests
andpip install beautifulsoup4
in the command line. - Send an HTTP request: Use the
requests
library to send an HTTP request to the website and retrieve the HTML content. - Parse the HTML content: Use the
BeautifulSoup
library to parse the HTML content and extract the data you want. - Extract the data: Once you have identified the HTML elements that contain the data you want, you can extract it using methods like
.text
,.get()
,.find_all()
, or.select()
. - Store or process the data: You can store the extracted data in a file or database, or process it further in your Python program.
Note: Be sure to review the website’s terms of use and robots.txt file to ensure that web scraping is allowed and to avoid overloading the website’s servers. Also, be sure to respect the website’s copyright and intellectual property rights.
A web scraper scrapes/gets data from webpages and saves it in any format we want, either .csv or .txt. We will build a simple web scraper in this section using a Python library called Beautiful Soup. [ Source : https://www.freecodecamp.org/news/python-program-examples-simple-code-examples-for-beginners/amp/ ]
Source Code :
import requests
from bs4 import BeautifulSoup
# Set the URL of the webpage you want to scrape
url = 'https://www.tocxten.com'
# Send an HTTP request to the URL and retrieve the HTML content
response = requests.get(url)
# Create a BeautifulSoup object that parses the HTML content
soup = BeautifulSoup(response.content, 'html.parser')
# Find all the links on the webpage
links = soup.find_all('a')
# Print the text and href attribute of each link
for link in links:
print(link.get('href'), link.text)
Output :
#textwp-posts-wrapper Skip to content
https://tocxten.com/
https://tocxten.com/ HOME
https://tocxten.com/index.php/privacy-policy/ ABOUT
https://tocxten.com/ Current Context
Tweets by tocxten
https://www.facebook.com/tocx.ten
https://www.linkedin.com/in/tocxten-ai-bb311a1b9/
https://www.youtube.com/channel/UCEwvOli0KZVgzn-QQekv6uw
mailto:tocxten@gmail.com
https://tocxten.com/wp-login.php?redirect_to=https%3A%2F%2Ftocxten.com%2Findex.php%2F2023%2F03%2F12%2Fintroduction-to-python-programming-python-basics-1-0%2F
#
https://tocxten.com/index.php/2023/03/12/introduction-to-python-programming-python-basics-1-0/
Project 5: Currency Converter
A currency converter is a program that helps users convert the value of one currency into another currency. You can use it for a variety of purposes, such as calculating the cost of international purchases, estimating travel expenses, or analyzing financial data. Note: we will use the ExchangeRate-API to get the exchange rate data, which is a free and open-source API for currency exchange rates. But there are other APIs available that may have different usage limits or requirements. [ Source : https://www.freecodecamp.org/news/python-program-examples-simple-code-examples-for-beginners/amp/ ]
Here’s an example of how to create a currency converter using Python:
- Install the requests library: You can use the
requests
library to retrieve the latest exchange rates from a public API. Install the library by runningpip install requests
in the command line. - Retrieve the exchange rates: Use the
requests
library to retrieve the latest exchange rates from a public API. For example, you can use thefixer.io
API, which provides exchange rates for a variety of currencies. To retrieve the exchange rates, send an HTTP GET request to the API endpoint, passing in your API access key and the base currency you want to convert from. - Implement the currency conversion function: Write a function that takes in the amount to convert, the currency to convert from, and the currency to convert to, and returns the converted amount. To convert the currency, multiply the amount by the exchange rate for the target currency.
- Implement the user interface: Create a simple user interface that prompts the user to enter the amount to convert, the currency to convert from, and the currency to convert to, and displays the converted amount.
- Test the program: Test the program by entering various amounts and currency conversions and verifying that the converted amounts are accurate.
Source Code :
# Import the necessary modules
import requests
# Define a function to convert currencies
def currency_converter(amount, from_currency, to_currency):
# Set the API endpoint for currency conversion
api_endpoint = f"https://api.exchangerate-api.com/v4/latest/{from_currency}"
# Send a GET request to the API endpoint
response = requests.get(api_endpoint)
# Get the JSON data from the response
data = response.json()
# Extract the exchange rate for the target currency
exchange_rate = data["rates"][to_currency]
# Calculate the converted amount
converted_amount = amount * exchange_rate
# Return the converted amount
return converted_amount
# Prompt the user to enter the amount, source currency, and target currency
amount = float(input("Enter the amount: "))
from_currency = input("Enter the source currency code: ").upper()
to_currency = input("Enter the target currency code: ").upper()
# Convert the currency and print the result
result = currency_converter(amount, from_currency, to_currency)
print(f"{amount} {from_currency} is equal to {result} {to_currency}")
Sample Output :
Enter the amount: 100
Enter the source currency code: USD
Enter the target currency code: INR
100.0 USD is equal to 8264.0 INR