Dr.Thyagaraju G S and Palguni G T:
A Python cheat sheet is a quick reference guide that summarizes the most important features of the Python programming language. It typically includes information on syntax, data types, control structures, functions, and modules, among other things.
Python cheat sheets are useful for both beginners and experienced developers who need a quick refresher on certain aspects of the language. They can also be used as study aids for learning Python, or as a handy reference guide when working on a project.
Here are some of the items you might find on a Python cheat sheet:
- Basic syntax: how to define variables, assign values, and use operators
- Control structures: if/else statements, loops (for and while), and conditional expressions
- Data types: integers, floats, booleans, strings, lists, tuples, sets, and dictionaries
- Functions: how to define and call functions, pass arguments, and use return values
- Modules: how to import and use built-in and third-party modules
- File I/O: how to read from and write to files
- Exception handling: how to catch and handle errors and exceptions
- Regular expressions: how to use regular expressions to search and manipulate strings
- Object-oriented programming: how to define and use classes and objects
Overall, a Python cheat sheet is a valuable resource for anyone who works with Python, and can help you save time and be more productive by providing quick and easy access to the most important features of the language.
- Zen of Python
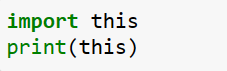
The Zen of Python is a collection of guiding principles for writing computer programs in the Python language. It was written by Tim Peters and is included as an easter egg in Python’s interpreter. Here are the 19 principles of the Zen of Python:
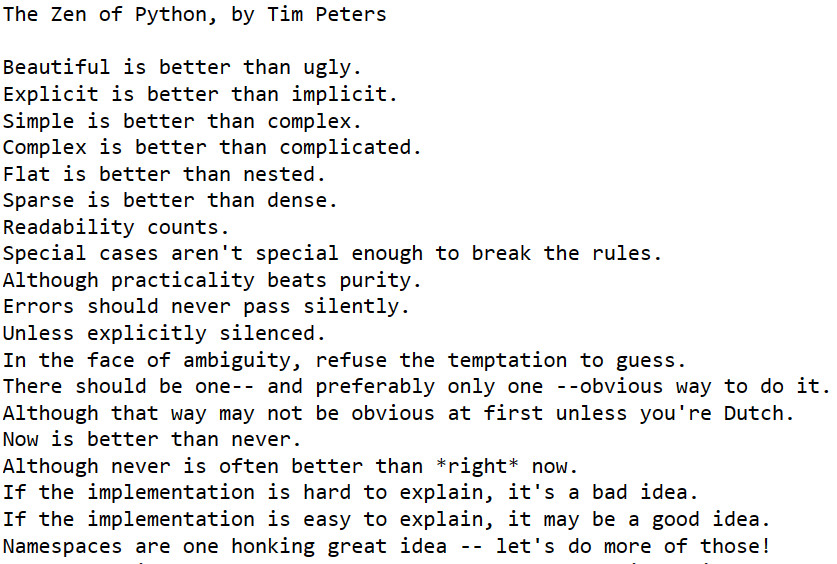
These principles encourage Python programmers to write code that is clear, readable, and maintainable, even at the cost of being slightly more verbose or less “clever.” The Zen of Python emphasizes the importance of simplicity, readability, and elegance in code, as well as the importance of writing code that is easy to understand and modify over time. By following these principles, Python programmers can create programs that are not only functional but also easy to use, understand, and build upon.
2.Python Keywords
Keywords in Python are the reserved words that have a special meaning and cannot be used as variable names or identifiers. Here’s a list of Python keywords:
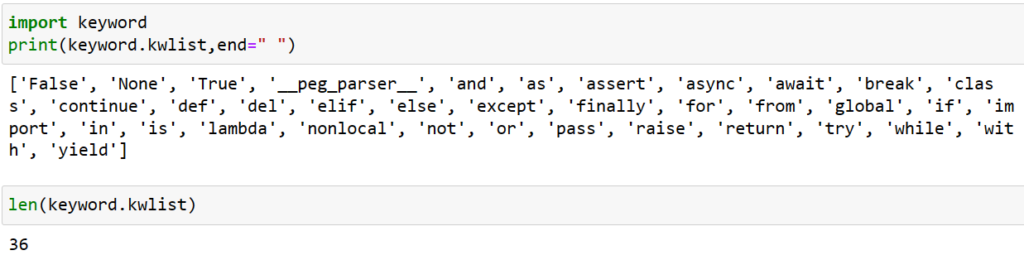
These keywords are used to define the syntax and structure of the Python language. For example, if
, else
, and elif
are used for conditional statements, while
and for
are used for loops, def
is used to define functions, and class
is used to define classes. It’s important to note that keywords cannot be used as variable names, so you should avoid using these words when naming your own variables in Python.
You can get a list of available keywords by using help()
:
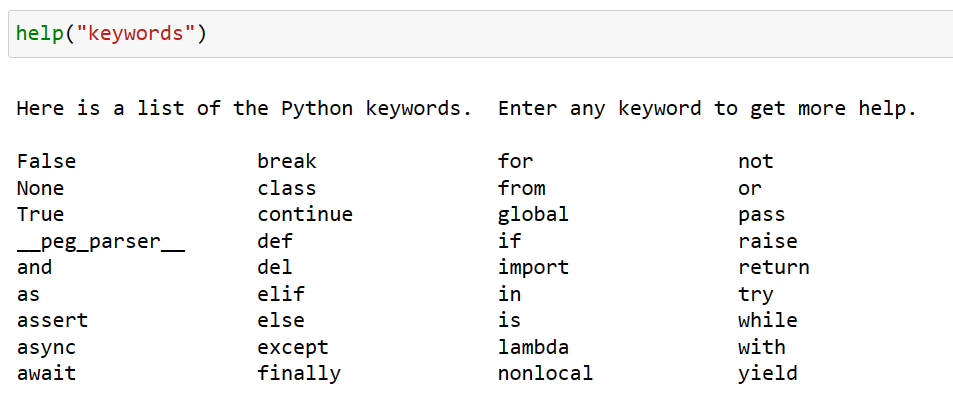
3.Comments
In Python, comments are used to annotate code and provide additional information to the programmer or other readers of the code. Comments are ignored by the Python interpreter and are not executed as part of the program. There are two ways to write comments in Python:
- Single-line comments: Single-line comments start with the hash (#) character and continue until the end of the line. For example:
# This is a single-line comment in Python
print("Hello, world!")
2. Multi-line comments: Multi-line comments are also known as docstrings, and they can span multiple lines of code. They are enclosed in triple quotes (either single or double) at the beginning and end of the comment block. For example:
"""
This is a multi-line comment or docstring
It can span multiple lines
"""
print("Hello, world!")
It is good practice to use comments to explain what your code does, especially for complex or unclear sections. Comments should be concise, clear, and helpful.
4.Indentation
In Python, indentation is used to define the structure and scope of blocks of code. It is a way to visually group statements and indicate the level of nesting within a program. Python uses indentation to indicate the level of code blocks. Code blocks that are at the same level of indentation are considered to be part of the same block of code.
The standard convention in Python is to use four spaces for indentation, although some developers may use a different number of spaces or even tabs. However, mixing tabs and spaces for indentation is not recommended, as it can lead to errors.
Here’s an example of how indentation is used in Python:
if x > 0:
print("x is positive")
else:
print("x is non-positive")
In this code snippet, the if
statement and the else
statement are indented by four spaces, indicating that they are part of the same block of code. The print
statements are also indented by four spaces within their respective blocks.
It’s important to note that indentation is significant in Python, and incorrect indentation can cause syntax errors or change the meaning of a program. Therefore, it’s essential to maintain consistent and correct indentation throughout your code.
5.Statements
In Python, a statement is a unit of code that performs a specific action or operation. In Python, statements can be classified as simple or compound. Simple statements are those that comprise a single line of code and are terminated by a newline character. Compound statements, on the other hand, consist of one or more clauses and use indentation to define the block of code. Here are some examples of simple statements in Python:
x = 5
print("Hello, world!")
Both of these statements are simple, as they consist of a single line of code. Here’s an example of a compound statement in Python:
if x > 0:
print("x is positive")
else:
print("x is non-positive")
This compound statement consists of an if
clause and an else
clause, both of which are indented to indicate that they are part of the same block of code. Here’s another example of a compound statement that uses multiple clauses:
for i in range(10):
if i < 5:
print(i, "is less than 5")
else:
print(i, "is greater than or equal to 5")
This compound statement consists of a for
loop and an if-else
statement, both of which are indented to indicate that they are part of the same block of code.
In general, simple statements in Python are those that can be executed in a single step, while compound statements are those that require multiple steps to execute. Simple statements are often used for simple operations, such as assigning values to variables or printing messages to the console, while compound statements are used for more complex operations, such as looping, branching, or exception handling.
6.Variables
In Python, variables are used to store data values that can be used later in the program. Variables are essentially names that refer to specific values in the computer’s memory. The value stored in a variable can be of different data types, such as numbers, strings, lists, tuples, and dictionaries.
# Variables can store values of different types
x = 5
y = "five"
z = True
7.Data Types
Python supports several built-in data types, which include:
- Numeric Types: Numeric types represent numeric values and can be divided into three categories:
# integer
x = 5
# float
y = 3.14
# complex
z = 2 + 3j
2. Boolean Type: The boolean data type represents the truth values True and False.
# boolean
a = True
b = False
3. Sequence Types:
- Strings (str): Strings are sequences of characters enclosed in quotes, such as “hello” or “world”.
- Lists (list): Lists are mutable sequences of objects, which means that you can change their values after they have been created.
- Tuples (tuple): Tuples are similar to lists but are immutable, which means that you cannot change their values after they have been created.
# string
s = "hello world"
# list
l = [1, 2, 3, "four", 5.0]
# tuple
t = (1, 2, 3, "four", 5.0)
4. Set Types:
- Sets (set): Sets are unordered collections of unique objects. Sets are mutable, which means that you can add or remove items from them.
- Frozen sets (frozenset): Frozen sets are similar to sets but are immutable, which means that you cannot change their values after they have been created.
# set
s = {1, 2, 3, 4}
# frozenset
fs = frozenset({1, 2, 3, 4})
5. Mapping Types:
Dictionaries (dict): Dictionaries are unordered collections of key-value pairs. Dictionaries are mutable, which means that you can add or remove items from them.
# Creating a dictionary
my_dict = {"name": "John", "age": 30, "city": "New York"}
# Accessing values
print(my_dict["name"]) # Output: John
print(my_dict.get("age")) # Output: 30
# Changing values
my_dict["age"] = 40
# Adding a new key-value pair
my_dict["country"] = "USA"
# Removing a key-value pair
del my_dict["city"]
# Looping through keys
for key in my_dict:
print(key)
# Looping through values
for value in my_dict.values():
print(value)
# Looping through key-value pairs
for key, value in my_dict.items():
print(key, value)
6. None
In Python, None
is a special built-in value that represents the absence of a value. It is often used as a placeholder or default value when you need to define a variable or function argument but don’t yet know the actual value. Here are some common uses of None
in Python:
- Default values: When defining a function that has an optional argument, you can use
None
as the default value, indicating that if the argument is not provided, it should be treated as if it were not passed at all. For example:
def my_function(arg1, arg2=None):
if arg2 is None:
arg2 = some_default_value
# rest of the function code
In this example, arg2
is an optional argument that defaults to None
if not provided. If arg2
is None
, the function assigns a default value to it.
2. Placeholder values: When defining a variable or data structure that will be assigned a value later, you can use None
as a placeholder value. For example:
my_var = None # this variable will be assigned a value later
my_list = [None] * 10 # this list has 10 elements, all initialized to None
3. Checking for absence of value: You can use None
to check if a variable or function argument has been assigned a value. For example:
if my_var is None:
print
4. Initialization : You can use None
to initialize a variable when you don’t have a value for it yet.
my_var = None
In this example, my_var
is initialized to None
, indicating that it has no value yet. Overall, None
is a useful constant in Python that can be used to represent the absence of a value, and can help make your code more clear and concise
8.Operators
In Python, operators are special symbols or keywords that are used to perform operations on values or variables. Here’s an overview of the different types of operators in Python:
- Arithmetic Operators: Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, division, modulus, and exponentiation. Examples include
+
,-
,*
,/
,%
, and**
. - Comparison Operators: Comparison operators are used to compare two values and return a Boolean value (True or False) depending on whether the comparison is true or false. Examples include
==
,!=
,>
,<
,>=
, and<=
. - Logical Operators: Logical operators are used to combine two or more Boolean expressions and return a Boolean value based on the result. Examples include
and
,or
, andnot
. - Assignment Operators: Assignment operators are used to assign values to variables. Examples include
=
,+=
,-=
,*=
,/=
, and%=
. - Bitwise Operators: Bitwise operators are used to perform operations on the binary representation of values. Examples include
&
(bitwise AND),|
(bitwise OR),^
(bitwise XOR),<<
(left shift), and>>
(right shift). - Membership Operators: Membership operators are used to test whether a value or variable is a member of a sequence or collection. Examples include
in
andnot in
. - Identity Operators: Identity operators are used to test whether two objects or variables refer to the same object in memory. Examples include
is
andis not
.
These are the main categories of operators in Python, and there are many different operators within each category. Understanding these operators is essential for writing effective and efficient Python code.
9.Python Control Statements
In Python, control statements are used to control the flow of execution of a program. They allow the program to make decisions based on conditions, execute code repeatedly, and skip or break out of loops. Here are the three main types of control statements in Python:
- Conditional statements: Conditional statements are used to execute different blocks of code based on whether a condition is true or false. The most commonly used conditional statement in Python is the
if
statement. Other conditional statements includeelif
andelse
. Example:
x = 5
if x < 10:
print("x is less than 10")
elif x > 10:
print("x is greater than 10")
else:
print("x is equal to 10")
2. Loop statements: Loop statements are used to execute a block of code repeatedly. The two most commonly used loop statements in Python are the for
loop and the while
loop. Example:
for i in range(5):
print(i)
while x < 10:
print(x)
x += 1
- Jump statements: Jump statements are used to change the normal flow of a program. The two most commonly used jump statements in Python are
break
andcontinue
. Example:
for i in range(10):
if i == 5:
break
print(i)
for i in range(10):
if i == 5:
continue
print(i)
10.Pass
In Python, pass
is a keyword that is used as a placeholder for an empty code block. It is used when you need to define a function, class, or loop that does nothing, but you need to have some code in that block to avoid a syntax error. The pass
statement does not do anything; it simply serves as a placeholder to tell Python that the code block is intentional left blank. Here’s an example of using pass
in Python:
def my_function():
pass # this function does nothing
In this example, we define a function called my_function
, but since we have not yet implemented the code to do anything in the function, we use pass
as a placeholder. This allows us to define the function without any syntax errors, and we can later fill in the function body with the actual code we want to execute.
Another example could be:
if x > 5:
pass # TODO: implement some code here
else:
print("x is less than or equal to 5")
In this example, we use pass
as a placeholder to remind ourselves that we need to implement some code in the if
block when x
is greater than 5. Overall, pass
is a useful keyword in Python when you need to define a code block that does nothing, but you need to have something there for syntactic reasons.
11.Functions
In Python, a function is a block of reusable code that performs a specific task. Functions are defined using the def
keyword, followed by the function name and a set of parentheses containing any input parameters. The body of the function is indented and can contain any valid Python code. Here is an example of a simple function that takes two arguments and returns their sum:
def add_numbers(a, b):
sum = a + b
return sum
In the above example, the function name is add_numbers
and it takes two input parameters a
and b
. The body of the function calculates the sum of the two input parameters and returns the result using the return
keyword.
To call the function and pass in arguments, you simply use the function name followed by the arguments in parentheses:
result = add_numbers(5, 10)
print(result) # Output: 15
Functions can also have optional parameters with default values, which can be useful in certain situations:
def greet(name, greeting="Hello"):
print(greeting + ", " + name + "!")
In the above example, the function greet
takes two parameters, name
and greeting
, with greeting
having a default value of “Hello”. If the greeting
parameter is not provided, the function will use the default value:
greet("Alice") # Output: Hello, Alice!
greet("Bob", "Hi") # Output: Hi, Bob!
Functions can also return multiple values using tuples:
def get_name_and_age():
name = input("Enter your name: ")
age = int(input("Enter your age: "))
return (name, age)
result = get_name_and_age()
print(result[0]) # Output: "Alice"
print(result[1]) # Output: 25
In the above example, the get_name_and_age
function returns a tuple containing the name
and age
variables. The result
variable is then assigned to this tuple and the individual values can be accessed using their index.
Functions can be passed as arguments to other functions:
def add_numbers(a, b):
return a + b
def multiply_numbers(a, b):
return a * b
def apply_operation(func, a, b):
return func(a, b)
result = apply_operation(add_numbers, 3, 5)
print(result) # Output: 8
result = apply_operation(multiply_numbers, 3, 5)
print(result) # Output: 15
Functions can also be nested within other functions:
def outer_function():
def inner_function():
print("Hello from inner function!")
inner_function()
outer_function() # Output: Hello from inner function!
12.Built in Functions
Python has a large number of built-in functions that can be used without needing to define them first. Here are some commonly used built-in functions in Python:
print()
: Used to display output to the console.len()
: Used to get the length of a sequence (list, tuple, string, etc.).type()
: Used to get the data type of a variable or object.input()
: Used to get input from the user via the console.str()
: Used to convert an object into a string.int()
: Used to convert an object into an integer.float()
: Used to convert an object into a floating-point number.range()
: Used to create a sequence of numbers.list()
: Used to create a list object from a sequence.tuple()
: Used to create a tuple object from a sequence.set()
: Used to create a set object from a sequence.dict()
: Used to create a dictionary object from a sequence of key-value pairs.max()
: Used to find the maximum value in a sequence.min()
: Used to find the minimum value in a sequence.sum()
: Used to find the sum of a sequence of numbers.abs()
: Used to find the absolute value of a number.round()
: Used to round off a number to a specified number of decimal places.ord()
: Used to get the Unicode value of a character.chr()
: Used to get the character corresponding to a Unicode value.enumerate()
: Used to iterate over a sequence and get the index and value of each item.
print()
: Used to display output to the console.
print("Hello, world!")
Output : Hello, world!
2. len()
: Used to get the length of a sequence (list, tuple, string, etc.).
my_list = [1, 2, 3, 4, 5]
print(len(my_list))
Output : 5
3.type()
: Used to get the data type of a variable or object.
my_string = "Hello, world!"
print(type(my_string))
Output : <class 'str'>
4. input()
: Used to get input from the user via the console.
user_input = input("Enter your name: ")
print("Hello, " + user_input + "!")
Output:
Enter your name: Palguni
Hello, Palguni!
5. str()
: Used to convert an object into a string.
my_number = 123
my_string = str(my_number)
print(type(my_string))
Output :
<class 'str'>
6. int()
: Used to convert an object into an integer.
7. range(): The range() function returns a sequence of numbers from start to stop with a given step size.
for i in range(1, 10, 2):
print(i)
8. max() and min(): The max() and min() functions return the maximum and minimum values from a sequence of numbers or characters.
Example:
numbers = [3, 5, 1, 2, 4]
print(max(numbers))
print(min(numbers))
chars = ['a', 'z', 'b', 'y']
print(max(chars))
print(min(chars))
9. abs(): The abs() function returns the absolute value of a number.
Example:
num = -3.14
print(abs(num))
10. round(): The round() function rounds a number to a specified number of decimal places.
Example:
num = 3.14159
print(round(num, 2))
11. sorted(): The sorted() function sorts a sequence of numbers or characters in ascending order.
Example:
numbers = [3, 5, 1, 2, 4]
sorted_numbers = sorted(numbers)
print(sorted_numbers)
chars = ['a', 'z', 'b', 'y']
sorted_chars = sorted(chars)
print(sorted_chars)
12. sum(): The sum() function returns the sum of all the elements in a sequence of numbers.
Example:
numbers = [3, 5, 1, 2, 4]
print(sum(numbers))
13.int(), float(),str(), bool(): These functions are used to convert values to different data types.
x = "5"
print(int(x)) # Output: 5
y = 3.14
print(str(y)) # Output: "3.14"
z = 0
print(bool(z)) # Output: False
These are just a few examples of the built-in functions available in Python. For a complete list, check the Python documentation.
13.Lists
In Python, a list is a collection of elements that are ordered and mutable. Lists are one of the most commonly used data structures in Python, and they can contain elements of different data types, including integers, floats, strings, booleans, and other lists.
Here are some characteristics of lists:
- Lists are ordered, which means that the order of elements in a list is preserved.
- Lists are mutable, which means that you can change the elements in a list after it has been created.
- Lists can contain elements of different data types, including integers, floats, strings, booleans, and other lists.
- Lists can be nested, which means that you can create lists of lists.
Lists are useful when you want to store a collection of related data that can be modified as needed. For example, you might use a list to store the names of students in a class or the temperatures in a particular city over a period of time.
.Here’s the syntax for creating a list in Python:
my_list = [element1, element2, element3, ...]
Examples for creating lists:
# A list of integers
numbers = [1, 2, 3, 4, 5]
# A list of strings
fruits = ['apple', 'banana', 'cherry']
# A list of mixed data types
mixed_list = [1, 'apple', 2.5, True]
# A list of lists
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
You can create an empty list in Python by simply assigning a pair of square brackets []
to a variable, like this:
my_list = []
You can also create an empty list using the list()
constructor, like this:
my_list = list()
You can also create an empty list and add elements to it later:
empty_list = []
empty_list.append(1)
empty_list.append(2)
empty_list.append(3)
print(empty_list) # Output: [1, 2, 3]
You can access elements of a list using their index (starting from 0):
numbers = [1, 2, 3, 4, 5]
print(numbers[0]) # Output: 1
print(numbers[2]) # Output: 3
You can also use negative indexing to access elements from the end of the list:
numbers = [1, 2, 3, 4, 5]
print(numbers[-1]) # Output: 5
print(numbers[-3]) # Output: 3
You can modify elements of a list by assigning a new value to their index:
numbers = [1, 2, 3, 4, 5]
numbers[1] = 10
print(numbers) # Output: [1, 10, 3, 4, 5]
You can add elements to a list using the append()
method:
fruits = ['apple', 'banana', 'cherry']
fruits.append('orange')
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
You can insert elements at a specific index using the insert()
method:
numbers = [1, 2, 3, 4, 5]
numbers.insert(2, 10)
print(numbers) # Output: [1, 2, 10, 3, 4, 5]
You can remove elements from a list using the remove()
method:
fruits = ['apple', 'banana', 'cherry', 'orange']
fruits.remove('banana')
print(fruits) # Output: ['apple', 'cherry', 'orange']
You can also use the pop()
method to remove an element at a specific index:
numbers = [1, 2, 3, 4, 5]
numbers.pop(2)
print(numbers) # Output: [1, 2, 4, 5]
You can check if an element is in a list using the in
keyword:
fruits = ['apple', 'banana', 'cherry']
print('apple' in fruits) # Output: True
print('orange' in fruits) # Output: False
You can sort a list using the sort()
method:
numbers = [3, 1, 4, 2, 5]
numbers.sort()
print(numbers) # Output: [1, 2, 3, 4, 5]
Reversing a list:
my_list.reverse()
print(my_list) # Output: ['two', 'five', 6, 3, 1]
Slicing a list:
print(my_list[1:4]) # Output: ['five', 6, 3]
print(my_list[:3]) # Output: ['two', 'five', 6]
print(my_list[2:]) # Output: [6, 3, 1]
Length of a list:
print(len(my_list)) # Output: 5
14.Tuples
In Python, a tuple is a collection of elements that are ordered and immutable. Tuples are similar to lists, but they cannot be modified once they are created. Tuples are defined using parentheses instead of square brackets.
Here are some characteristics of tuples:
- Tuples are ordered, which means that the order of elements in a tuple is preserved.
- Tuples are immutable, which means that once a tuple is created, you cannot change its elements.
- Tuples can contain elements of different data types, including integers, floats, strings, booleans, and other tuples.
Tuples are useful when you want to group related data together, but you don’t want that data to be modified accidentally. For example, you might use a tuple to represent a point in two-dimensional space, where the first element of the tuple is the x-coordinate and the second element is the y-coordinate.
Here’s the syntax for creating a tuple in Python:
my_tuple = (element1, element2, element3, ...)
Here are some examples of how to create tuples in Python:
# A tuple of integers
my_tuple1 = (1, 2, 3, 4, 5)
# A tuple of strings
my_tuple2 = ('apple', 'banana', 'cherry')
# A tuple of mixed data types
my_tuple3 = (1, 'apple', 2.5, True)
# A tuple with a single element
my_tuple4 = (1,) # Note the comma after the element
# A nested tuple
nested_tuple = ((1, 2), (3, 4), (5, 6))
You can also create an empty tuple:
empty_tuple = ()
You can access elements of a tuple using their index (starting from 0):
my_tuple = ('apple', 'banana', 'cherry')
print(my_tuple[0]) # Output: 'apple'
print(my_tuple[2]) # Output: 'cherry'
You can also use negative indexing to access elements from the end of the tuple:
my_tuple = ('apple', 'banana', 'cherry')
print(my_tuple[-1]) # Output: 'cherry'
print(my_tuple[-2]) # Output: 'banana'
Note that tuples are immutable, which means you cannot modify them once they are created. For example, the following code will raise a TypeError
:
my_tuple = ('apple', 'banana', 'cherry')
my_tuple[1] = 'orange' # Raises TypeError: 'tuple' object does not support item assignment
You can, however, create a new tuple by concatenating existing tuples:
my_tuple1 = ('apple', 'banana', 'cherry')
my_tuple2 = ('orange', 'grape', 'mango')
new_tuple = my_tuple1 + my_tuple2
print(new_tuple) # Output: ('apple', 'banana', 'cherry', 'orange', 'grape', 'mango')
You can also use the tuple()
constructor to create a tuple from an iterable, such as a list:
my_list = [1, 2, 3, 4, 5]
my_tuple = tuple(my_list)
print(my_tuple) # Output: (1, 2, 3, 4, 5)
Finally, you can use tuple unpacking to assign values from a tuple to variables:
my_tuple = ('apple', 'banana', 'cherry')
a, b, c = my_tuple
print(a) # Output: 'apple'
print(b) # Output: 'banana'
print(c) # Output: 'cherry'
This can also be used to swap the values of two variables:
a = 1
b = 2
a, b = b, a
print(a) # Output: 2
print(b) # Output: 1
15.Dictionaries
In Python, a dictionary is a collection of key-value pairs that are unordered and mutable. Dictionaries are sometimes called “associative arrays” or “hash tables” in other programming languages.
Here are some characteristics of dictionaries:
- Dictionaries are unordered, which means that the order of elements in a dictionary is not preserved.
- Dictionaries are mutable, which means that you can change the values associated with a key after it has been created.
- Keys in a dictionary must be unique and immutable, which means that they cannot be changed after they have been created. Common key types include strings and integers.
- Values in a dictionary can be of any data type, including integers, floats, strings, booleans, lists, tuples, and other dictionaries.
Dictionaries are useful when you want to store data that can be accessed quickly by a key. For example, you might use a dictionary to store information about a person, where the keys are the person’s name, age, and address, and the values are the corresponding information.
In Python, dictionaries are defined using curly braces {} and a colon : to separate keys and values. Here’s the general syntax for creating a dictionary:
my_dict = {key1: value1, key2: value2, key3: value3, ...}
Here are some examples of dictionaries in Python:
Example 1: A dictionary of names and ages
person_dict = {'Alice': 25, 'Bob': 30, 'Charlie': 35}
In this example, person_dict
contains three key-value pairs, where the keys are strings representing the names and the values are integers representing the ages.
Example 2: A dictionary of coordinates
point_dict = {'x': 10, 'y': 20}
In this example, point_dict
contains two key-value pairs, where the keys are strings representing the coordinates and the values are integers representing the values of the coordinates.
You can access the values in a dictionary using their keys. For example:
print(person_dict['Alice']) # Output: 25
print(point_dict['y']) # Output: 20
You can also add or modify key-value pairs in a dictionary by assigning a value to a new key, or by assigning a new value to an existing key:
person_dict['David'] = 40 # add a new key-value pair
person_dict['Alice'] = 26 # modify the value associated with an existing key
Finally, you can use various dictionary methods to manipulate dictionaries. For example, you can use the keys()
method to get a list of all the keys in a dictionary, and the values()
method to get a list of all the values in a dictionary.
16.Strings
In Python, a string is a sequence of characters enclosed in quotation marks (either single quotes or double quotes). Strings are a fundamental data type in Python, and they are used to represent textual data.
Here are some characteristics of strings:
- Strings are immutable, which means that once a string is created, you cannot change its contents. However, you can create a new string by concatenating or slicing existing strings.
- Strings are iterable, which means that you can loop over the characters in a string using a
for
loop. - Strings can be indexed and sliced, which means that you can access individual characters or substrings within a string using square brackets [].
In Python, strings are created by enclosing a sequence of characters in either single quotes (‘…’) or double quotes (“…”). Here’s an example:
my_string = "Hello, World!"
In this example, my_string
is a string that contains the characters “Hello, World!”.
You can access individual characters in a string using square brackets [] and an index, where the index starts at 0 for the first character, 1 for the second character, and so on. Here’s an example:
print(my_string[0]) # Output: 'H'
You can also use negative indices to access characters from the end of the string. For example:
print(my_string[-1]) # Output:
You can concatenate strings using the +
operator, or you can repeat a string using the *
operator. Here are some examples:
greeting = "Hello"
name = "Alice"
message = greeting + ", " + name + "!"
print(message) # Output: "Hello, Alice!"
repeat_string = "Python " * 3
print(repeat_string) # Output: "Python Python Python "
You can also use various string methods to manipulate strings, such as upper()
to convert all the characters in a string to uppercase, lower()
to convert all the characters to lowercase, strip()
to remove whitespace from the beginning and end of a string, and many more. Here are some examples:
my_string = " Hello, World! "
print(my_string.strip()) # Output: "Hello, World!"
my_string = "Hello, World!"
print(my_string.upper()) # Output: "HELLO, WORLD!"
In summary, strings are a fundamental data type in Python that are used to represent textual data, and they can be manipulated in many different ways using various string methods.
list of some common string handling methods in Python:
# lower(): Converts all characters in a string to lowercase.
my_string = "Hello, World!"
print(my_string.lower()) # Output: "hello, world!
# upper(): Converts all characters in a string to uppercase.
my_string = "Hello, World!"
print(my_string.upper()) # Output: "HELLO, WORLD!"
# strip(): Removes whitespace from the beginning and end of a string.
my_string = " Hello, World! "
print(my_string.strip()) # Output: "Hello, World!"
# split(): Splits a string into a list of substrings based on a specified delimiter.
my_string = "apple,banana,orange"
print(my_string.split(",")) # Output: ['apple', 'banana', 'orange']
#join(): Joins a list of strings into a single string using a specified delimiter.
my_list = ['apple', 'banana', 'orange']
print(",".join(my_list)) # Output: "apple,banana,orange"
# replace(): Replaces all occurrences of a specified substring in a string with another substring.
my_string = "Hello, World!"
print(my_string.replace("World", "Python")) # Output: "Hello, Python!"
#startswith(): Returns True if a string starts with a specified substring.
my_string = "Hello, World!"
print(my_string.startswith("Hello")) # Output: True
# endswith(): Returns True if a string ends with a specified substring.
my_string = "Hello, World!"
print(my_string.endswith("World!")) # Output: True
#find(): Searches a string for a specified substring and returns the index of the first occurrence (or -1 if not found).
my_string = "Hello, World!"
print(my_string.find("World")) # Output: 7
#count(): Counts the number of occurrences of a specified substring in a string.
my_string = "Hello, World!"
print(my_string.count("l")) # Output: 3
17.File Handling
Files are a way of storing and manipulating data on a computer’s file system. In Python, files are represented as objects, which provide methods for reading and writing data to and from the file.
Python provides built-in functions for working with files, including open()
, close()
, read()
, write()
, and many others.
The open()
function is used to open a file and returns a file object. The function takes two arguments: the name of the file to open (including the path, if necessary), and the mode in which to open the file (e.g., read-only, write-only, or read-write). Here’s an example:
open()
function: This function is used to open a file and returns a file object. The function takes two arguments: the name of the file to open (including the path, if necessary), and the mode in which to open the file (e.g., read-only, write-only, or read-write).
Syntax:
file = open(file_name, mode)
Example:
file = open("myfile.txt", "r")
2.close()
method: This method is used to close an opened file.
# Syntax
file.close()
# Example
file = open("myfile.txt", "r")
# do something with the file
file.close()
3. read()
method: This method is used to read data from a file.
# Syntax:
data = file.read(size)
# Example :
file = open("myfile.txt", "r")
data = file.read()
print(data)
file.close()
4. write()
method: This method is used to write data to a file.
#Syntax:
file.write(data)
#Example :
file = open("myfile.txt", "w")
file.write("Hello, World!")
file.close()
5. readline()
method: This method is used to read a single line from a file.
# Syntax
line = file.readline()
#Example:
file = open("myfile.txt", "r")
line = file.readline()
print(line)
file.close()
6. writelines()
method: This method is used to write a list of lines to a file.
# Syntax
file.writelines(lines)
# Example
file = open("myfile.txt", "w")
lines = ["line 1\n", "line 2\n", "line 3\n"]
file.writelines(lines)
file.close()
18.Object Oriented Programming in Python
Object-oriented programming (OOP) is a programming paradigm that emphasizes the use of objects, which are instances of classes that encapsulate data and behavior. In Python, you can define classes and create objects from them to implement OOP.
Here’s an example of a simple class definition:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
In this example, we define a Person
class that has two attributes (name
and age
) and one method (say_hello
). The __init__
method is a special method that is called when an object of the class is created. It initializes the attributes of the object with the values passed in as arguments. To create an object of the Person
class, we can use the following code:
p = Person("Alice", 30)
In this example, we create a Person
object named p
with the name “Alice” and age 30. We can then call the say_hello
method on the p
object like this:
p.say_hello()
In this example, we create a Person
object named p
with the name “Alice” and age 30. We can then call the say_hello
method on the p
object like this:
p.say_hello()
This will print “Hello, my name is Alice and I am 30 years old.” to the console.
Here’s another example that shows how to define a subclass of an existing class:
class Student(Person):
def __init__(self, name, age, major):
super().__init__(name, age)
self.major = major
def say_hello(self):
print(f"Hello, my name is {self.name} and I am a {self.major} major.")
In this example, we define a Student
class that is a subclass of the Person
class. The Student
class adds a new attribute (major
) and overrides the say_hello
method to print a different message.
To create a Student
object, we can use the following code:
s = Student("Bob", 20, "Computer Science")
In this example, we create a Student
object named s
with the name “Bob”, age 20, and major “Computer Science”.
We can then call the say_hello
method on the s
object like this:
s.say_hello()
This will print “Hello, my name is Bob and I am a Computer Science major.” to the console.
These are just a few examples of how to use object-oriented programming in Python. By defining classes and creating objects, you can encapsulate data and behavior in a way that makes your code more modular, reusable, and easier to maintain.
19.Decorators
Decorators in Python are functions that can modify the behavior of other functions or classes. They allow you to add functionality to existing code without modifying it directly. Decorators are typically used for implementing cross-cutting concerns such as logging, authentication, and caching.
Here’s an example of a simple decorator function that adds logging to a function:
def log(func):
def wrapper(*args, **kwargs):
print(f"Calling function {func.__name__}")
return func(*args, **kwargs)
return wrapper
In this example, the log
function takes another function func
as an argument and returns a new function wrapper
. The wrapper
function logs the name of the function being called and then calls the original function func
with the same arguments.
To use the log
decorator, you simply apply it to a function by placing the @log
decorator above the function definition:
In this example, the add
function is decorated with the log
decorator. When the add
function is called, the log
decorator will be applied and the name of the function will be logged to the console.
Here’s another example of a decorator that adds caching to a function:
def cache(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
else:
result = func(*args)
cache[args] = result
return result
return wrapper
In this example, the cache
decorator maintains a cache of results for the decorated function. When the decorated function is called with the same arguments as before, the cached result is returned instead of recomputing the result.
To use the cache
decorator, you simply apply it to a function by placing the @cache
decorator above the function definition:
@cache
def fibonacci(n):
if n < 2:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
In this example, the fibonacci
function is decorated with the cache
decorator. When the fibonacci
function is called with the same argument as before, the cached result will be returned instead of recomputing the result.
These are just a few examples of how to use decorators in Python. Decorators are a powerful tool that can be used to modify the behavior of functions or classes in a flexible and modular way.
20.Exception Handling
Exception handling in Python is a way to handle errors and exceptions that may occur during program execution. It allows you to gracefully handle errors and prevent your program from crashing.
In Python, you can use the try-except
block to handle exceptions. The try
block contains the code that may raise an exception, while the except
block contains the code to handle the exception. Here’s an example:
try:
num1 = int(input("Enter a number: "))
num2 = int(input("Enter another number: "))
result = num1 / num2
print(f"The result is {result}")
except ZeroDivisionError:
print("Error: You cannot divide by zero.")
except ValueError:
print("Error: You must enter a valid number.")
In this example, the try
block prompts the user to enter two numbers and performs division. If an error occurs, the corresponding except
block is executed. If a ZeroDivisionError
occurs, a message is printed indicating that the user cannot divide by zero. If a ValueError
occurs, a message is printed indicating that the user must enter a valid number.
You can also use the finally
block to execute code after the try
and except
blocks, regardless of whether an exception occurred. Here’s an example:
try:
f = open("file.txt", "r")
data = f.read()
print(data)
except FileNotFoundError:
print("Error: The file does not exist.")
finally:
f.close()
In this example, the try
block attempts to open a file and read its contents. If the file does not exist, a FileNotFoundError
is raised and a message is printed. The finally
block ensures that the file is closed, regardless of whether an exception occurred.
You can also define your own custom exceptions by subclassing the Exception
class. Here’s an example:
class MyException(Exception):
pass
try:
x = 10
if x > 5:
raise MyException("x is too big")
except MyException as e:
print(e)
In this example, a custom exception MyException
is defined by subclassing the Exception
class. The raise
statement is used to raise the MyException
exception with a custom message. The except
block catches the MyException
exception and prints the custom message.
Exception handling is an important part of writing robust and reliable code. By handling exceptions gracefully, you can prevent your program from crashing and provide a better user experience.
21.Modules in Python
In Python, a module is a file that contains Python definitions and statements. It is used to group related code together, so it can be easily imported and reused in other Python programs. Modules can be written in Python itself or in a language like C and loaded dynamically into Python.
A module can contain functions, classes, and variables, which can be accessed using the dot notation after importing the module. For example, if we have a module called my_module.py
, which contains a function called my_function()
, we can import and use it in another Python program like this:
import my_module
result = my_module.my_function()
Python has a large number of built-in modules that provide useful functionality, such as os
for interacting with the operating system, datetime
for working with dates and times, and random
for generating random numbers. Additionally, Python users can create their own modules and share them with others, either by publishing them on the web or by packaging them in libraries.
Here are some examples of Python modules:
random
module – provides functions for generating random numbers, choosing random elements from a list, and shuffling sequences randomly.
import random
# Generate a random integer between 1 and 100
random_number = random.randint(1, 100)
print(random_number)
# Choose a random element from a list
fruits = ["apple", "banana", "cherry"]
random_fruit = random.choice(fruits)
print(random_fruit)
# Shuffle a list randomly
random.shuffle(fruits)
print(fruits)
2. math
module – provides mathematical functions like trigonometric functions, logarithmic functions, and mathematical constants.
import math
# Find the sine of pi/2
sin_value = math.sin(math.pi/2)
print(sin_value)
# Find the logarithm of 1000 to base 10
log_value = math.log10(1000)
print(log_value)
# Find the value of pi
pi_value = math.pi
print(pi_value)
3. os
module – provides functions for interacting with the operating system, such as working with files and directories.
import os
# Create a new directory
os.mkdir("new_directory")
# Check if a file exists
file_exists = os.path.exists("file.txt")
print(file_exists)
# Get the current working directory
current_directory = os.getcwd()
print(current_directory)
4. datetime
module – provides functions for working with dates and times.
import datetime
# Get the current date and time
current_datetime = datetime.datetime.now()
print(current_datetime)
# Create a date object for a specific date
date = datetime.date(2023, 3, 19)
print(date)
# Format a date as a string
date_string = date.strftime("%d-%m-%Y")
print(date_string)
There are many modules available in Python’s standard library that provide a wide range of functionality. Here is a non-exhaustive list of some of the most commonly used modules:
os
– provides a way to interact with the operating system, including accessing the file system, environment variables, and system calls.sys
– provides access to some variables used or maintained by the Python interpreter and functions that interact strongly with the interpreter.math
– provides mathematical functions, including trigonometry, logarithms, and constants like pi.random
– provides functions for generating random numbers, choosing random elements from a list, and shuffling sequences randomly.datetime
– provides classes for working with dates and times.json
– provides functions for encoding and decoding JSON data.csv
– provides classes for working with CSV (comma-separated values) files.sqlite3
– provides a way to interact with SQLite databases.re
– provides support for regular expressions.argparse
– provides a way to parse command-line arguments.collections
– provides alternative data structures like named tuples, deques, and defaultdicts.urllib
– provides functions for working with URLs and URIs.requests
– provides an easy-to-use API for making HTTP requests.pickle
– provides a way to serialize Python objects into a byte stream.threading
– provides classes for working with threads and synchronization primitives like locks and semaphores.logging
– provides a way to log messages from your Python code.time
– provides functions for working with time, including measuring elapsed time and sleeping.socket
– provides a way to work with sockets, including creating client and server applications.subprocess
– provides a way to spawn new processes and interact with them.zipfile
– provides classes for working with ZIP archives.
This is just a small sampling of the many modules available in Python’s standard library. There are many more modules available for specific purposes, such as scientific computing, web development, and data analysis.
22.Type Dispatch
Type dispatching in Python refers to the process of dynamically selecting the appropriate implementation of a function or method based on the types of the arguments passed to it at runtime. This is also known as “runtime polymorphism” or “dynamic dispatch”.
Python provides a built-in mechanism for type dispatching called “duck typing”. In Python, the type of an object is determined by its behavior rather than its explicit type. This means that if two objects have the same behavior, they are considered to be of the same type, even if they are not of the same class.
To illustrate this concept, consider the following example:
def add_numbers(x, y):
return x + y
print(add_numbers(1, 2)) # Output: 3
print(add_numbers(1.5, 2.5)) # Output: 4.0
print(add_numbers("hello", "world")) # Output: helloworld
In this example, the add_numbers
function takes two arguments x
and y
and returns their sum. Notice that the function is able to add both integers and floating-point numbers as well as concatenate two strings without any explicit type annotations. This is possible because Python uses duck typing to determine the types of the arguments at runtime and dynamically selects the appropriate implementation of the +
operator.
Another way to implement type dispatching in Python is through the use of function overloading. Function overloading involves defining multiple functions with the same name but different parameter types or number of parameters. Here is an example:
def add_numbers(x: int, y: int) -> int:
return x + y
def add_numbers(x: float, y: float) -> float:
return x + y
print(add_numbers(1, 2)) # Output: 3
print(add_numbers(1.5, 2.5)) # Output: 4.0
23. Lambda Function
A Lambda function in Python is an anonymous function, meaning it is a function without a name, that can be defined and used in a single line of code. Lambda functions are commonly used in functional programming and are often passed as arguments to higher-order functions.
The syntax of a Lambda function in Python is as follows:
lambda arguments: expression
Where arguments
is a comma-separated list of arguments, and expression
is the operation performed on those arguments. The result of the expression is returned automatically by the function. Here is an example of a Lambda function that returns the sum of two numbers:
addition = lambda x, y: x + y
result = addition(3, 5)
print(result) # output : 8
24. Map Function
The map()
function in Python is a built-in function that applies a specified function to each item of an iterable (e.g. a list, tuple, set) and returns a new iterable object that contains the results.
The basic syntax for the map()
function is as follows:
map(function, iterable)
where function
is the function to be applied to each item of the iterable
. iterable
is the sequence of items to be passed to the function.Here’s an example of using the map()
function to apply a function to a list of numbers:
# Define a function that squares a number
def square(x):
return x**2
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Apply the square function to each item in the list using map()
squared_numbers = map(square, numbers)
# Convert the map object to a list to print the results
print(list(squared_numbers))
This will output:
[1, 4, 9, 16, 25]
In this example, the square()
function is applied to each item in the numbers
list using the map()
function. The resulting map
object is then converted to a list and printed. We can also use lambda functions with map()
to make the code more concise. Here’s an example:
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Apply a lambda function that squares each number to the list using map()
squared_numbers = map(lambda x: x**2, numbers)
# Convert the map object to a list to print the results
print(list(squared_numbers))
This will output the same result as before:
[1,4,9,16,25]
25. Filter Function
The filter()
function in Python is a built-in function used for filtering out elements from an iterable based on a given function’s condition. It returns an iterator that contains the elements from the input iterable that satisfy the given condition.
The syntax of the filter()
function is:
filter(function, iterable)
where:
function
: A function that takes a single argument and returns a Boolean value indicating whether the argument satisfies the filter condition.iterable
: An iterable sequence to be filtered.
Here’s an example that demonstrates how to use the filter()
function:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
def is_even(num):
return num % 2 == 0
even_numbers = list(filter(is_even, numbers))
print(even_numbers)
In this example, we have a list of numbers. We define a function is_even()
that takes a number as an argument and returns True
if the number is even, False
otherwise. We then use the filter()
function to create a new list called even_numbers
that contains only the even numbers from the original list of numbers
.
Output:
[2, 4, 6, 8, 10]
In this example, the filter()
function calls the is_even()
function for each element in the numbers
list. If the is_even()
function returns True
, the element is included in the even_numbers
list. If it returns False
, the element is excluded from the even_numbers
list.
26. match case
The Python match
statement, introduced in Python 3.10, is a new feature that provides a concise and expressive way to perform pattern matching. It allows you to compare a value against multiple patterns and execute different code blocks based on the matched pattern.
Here’s an example to illustrate how the match
statement works:
def process_data(data):
match data:
case 1:
print("Processing data with value 1")
case 2:
print("Processing data with value 2")
case _:
print("Processing data with unknown value")
data1 = 1
process_data(data1) # Output: Processing data with value 1
data2 = 2
process_data(data2) # Output: Processing data with value 2
data3 = 3
process_data(data3) # Output: Processing data with unknown value
In the example above, we define a function called process_data
that takes a parameter data
. Inside the function, we use the match
statement to match the value of data
against different patterns.
In the first case, if the value of data
is 1
, the code block under case 1:
is executed, which prints “Processing data with value 1”.
In the second case, if the value of data
is 2
, the code block under case 2:
is executed, which prints “Processing data with value 2”.
The third case uses _
as a wildcard pattern, which matches any value. If none of the previous patterns match, the code block under case _:
is executed, which prints “Processing data with unknown value”.
The match
statement can handle more complex patterns using various match patterns, such as constant patterns, value patterns, sequence patterns, mapping patterns, and more. It offers a powerful way to write more expressive and readable code for handling different cases or conditions.
27. List Comprehension
List comprehension is a concise way to create lists in Python. It allows you to create a new list by applying an expression to each element of an existing iterable (such as a list, tuple, or range) and optionally applying a filter condition.
The basic syntax of list comprehension is as follows:
new_list = [expression for element in iterable if condition]
Let’s break down the components:
expression
: It represents the operation or transformation to be applied to each element of the iterable. This expression determines what will be included in the new list.element
: It represents the current element being processed from the iterable.iterable
: It is the source iterable from which elements are taken to create the new list.condition
(optional): It is an optional filtering condition that determines whether an element should be included in the new list. Only the elements that satisfy the condition will be included.
Here’s an example to illustrate list comprehension:
# Create a new list of squares of numbers from 0 to 9
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
In the example above, we use list comprehension to create a new list called squares
. The expression x**2
calculates the square of each element x
from the range(10)
iterable. Since there is no filtering condition specified, all the elements from 0 to 9 are included in the new list.
List comprehension can also include an if
condition to filter elements. Here’s an example:
# Create a new list of even numbers from 0 to 9
even_numbers = [x for x in range(10) if x % 2 == 0]
print(even_numbers) # Output: [0, 2, 4, 6, 8]
In this example, the list comprehension includes the condition x % 2 == 0
, which filters out odd numbers. Only the even numbers from 0 to 9 are included in the new list.
List comprehension is a powerful feature that allows you to create new lists in a concise and readable way, combining iteration and transformation operations in a single line of code. It can greatly simplify your code when dealing with transformations and filtering of data.