Syllabus :
Lists: The List Data Type, Working with Lists, Augmented Assignment Operators, Methods, Example Program: Magic 8 Ball with a List, List-like Types: Strings and Tuples, References,
In Python, a sequence is an ordered collection of elements, where each element is identified by an index. Each element of a sequence is assigned a number – its position or index. The first index is zero, the second index is one, and so forth.
There are three types of sequences in Python: lists, tuples, and strings.
List Data type
In Python, a list is a collection of ordered and mutable elements. A list can contain elements of different data types, such as integers, floats, strings, and even other lists. Lists are created by enclosing a comma-separated sequence of values within square brackets [ ].
Example:
my_list = [1, 2, 3, "four", 5.0, [6, 7, 8]]
Different ways to create List
In Python, there are different ways to create a list. Here are some examples:
- Using square brackets: You can create a list by enclosing a sequence of elements within square brackets. The elements can be of any data type, and can include integers, floats, strings, and even other lists.
Example:
my_list = [1, 2, 3, "four", 5.0, [6, 7, 8]]
- Using the list() function: You can create a list by passing a sequence of elements to the
list()
function. The sequence can be any iterable object, such as a tuple or a string.
Example:
my_tuple = (1, 2, 3, "four", 5.0)
my_list = list(my_tuple)
3. Using append() method : The append()
method adds a single element to the end of the list.
Example :
my_list = []
my_list.append(1)
my_list.append(2)
my_list.append(3)
print(my_list) # Output: [1, 2, 3]
4. Using extend() method : The extend()
method adds multiple elements to the end of the list by appending each element from the iterable that is passed as an argument.
Example :
my_list = []
my_list.extend([1, 2, 3])
print(my_list) # Output: [1, 2, 3]
In this example, the extend()
method is used to add multiple elements to the end of the list at once. The elements are passed as a list argument to the extend()
method.
Note that the extend()
method can also add elements from other iterable objects, such as tuples or other lists.
my_list = []
my_list.extend((1, 2, 3))
print(my_list) # Output: [1, 2, 3]
other_list = [4, 5, 6]
my_list.extend(other_list)
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
5. Using list comprehension: You can create a list using a list comprehension, which is a concise way to create a list based on an existing sequence.
Example:
my_list = [x for x in range(10)]
This creates a list of integers from 0 to 9.
6. Using the range() function: You can create a list of integers using the range()
function, which generates a sequence of integers based on the given arguments.
Example :
my_list = list(range(1, 10, 2))
This creates a list of odd numbers from 1 to 9.
7. Using the split() method:
You can create a list of strings by splitting a string based on a delimiter. The split()
method splits a string into a list of substrings based on a specified separator.
Example:
my_string = "apple,banana,orange"
my_list = my_string.split(",")
This creates a list of strings containing the fruits “apple”, “banana”, and “orange”.
These are some of the ways to create a list in Python. Depending on the situation, one method may be more appropriate than the others.
Example : Creation of Empty list
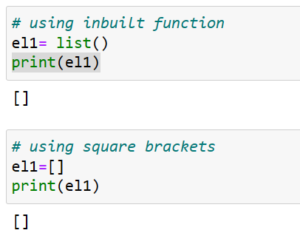
Example : Creation of List with values
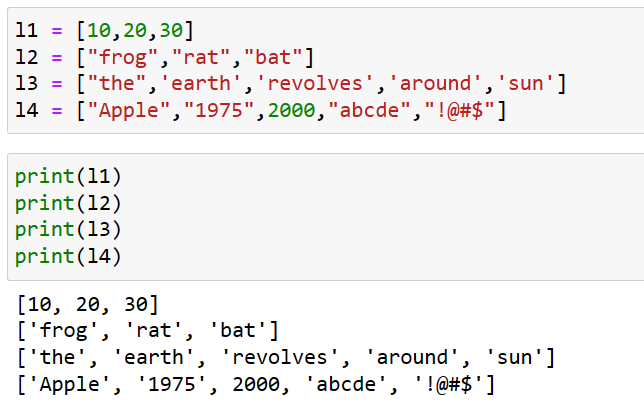
Lists are Mutable
In Python, a list is a collection of items that are ordered and changeable. One key feature of lists is that they are mutable, meaning that their elements can be modified after they are created.
Here’s an example of how a list can be modified in Python:
# create a list of fruits
fruits = ['apple', 'banana', 'orange']
# add a new fruit to the list
fruits.append('strawberry')
print(fruits) # Output: ['apple', 'banana', 'orange', 'strawberry']
# remove an item from the list
fruits.remove('banana')
print(fruits) # Output: ['apple', 'orange', 'strawberry']
# change an item in the list
fruits[0] = 'kiwi'
print(fruits) # Output: ['kiwi', 'orange', 'strawberry']
In this example, we create a list called fruits
containing three elements: 'apple'
, 'banana'
, and 'orange'
. We then use the append()
method to add a new element, 'strawberry'
, to the end of the list. Next, we use the remove()
method to remove the 'banana'
element from the list. Finally, we use indexing to change the first element of the list from 'apple'
to 'kiwi'
.
This example demonstrates that lists are mutable because we are able to add, remove, and modify elements within the list after it has been created. This can be very useful in programming, as it allows us to dynamically update our data structures as needed.
Example for Mutability :
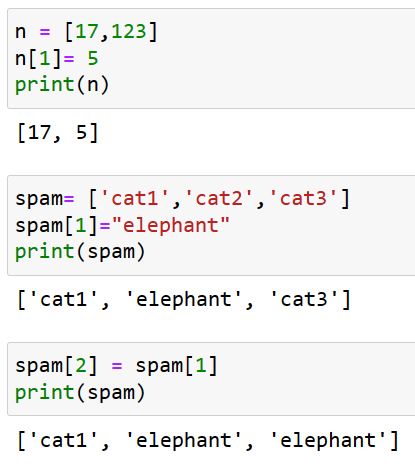
List Concatenation
List concatenation is the process of combining two or more lists into a single list. In Python, there are multiple ways to concatenate lists. Here are some examples:
- Using the ‘+’ operator:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
concatenated_list = list1 + list2
print(concatenated_list) # Output: [1, 2, 3, 4, 5, 6]
In the above example, we have two lists, list1
and list2
. To concatenate them, we use the ‘+’ operator, which creates a new list that contains all the elements of both list1
and list2
.
- Using the
extend()
method:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6
3. Using the append()
method in a loop:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
for item in list2:
list1.append(item)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
In the above example, we have two lists, list1
and list2
. To concatenate them, we use a loop that iterates over each element of list2
and appends it to list1
using the append()
method.
Example :
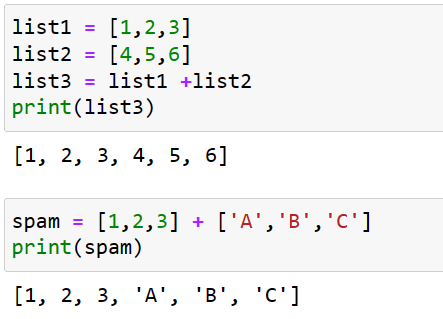
List Replication
List replication is a process of creating a new list by replicating the elements of an existing list multiple times. In Python, list replication can be achieved using the ‘*’ operator. Here are some examples:
- Replicating a list multiple times:
original_list = [1, 2, 3]
replicated_list = original_list * 3
print(replicated_list) # Output: [1, 2, 3, 1, 2, 3, 1, 2, 3]
In the above example, we have an original list original_list
with three elements. We replicate this list three times using the ‘*’ operator, which creates a new list replicated_list
that contains the elements of original_list
repeated three times.
2. Creating a list of a specific length with a single element repeated:
single_element_list = [0] * 5
print(single_element_list) # Output: [0, 0, 0, 0, 0]
In the above example, we create a new list single_element_list
that contains the element 0 repeated five times using the ‘*’ operator. This is a useful technique when you need to create a list of a specific length with a single element repeated.
3. Replicating a list using a variable:
original_list = [1, 2, 3]
n = 4
replicated_list = original_list * n
print(replicated_list) # Output: [1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3]
In the above example, we use a variable n
to specify the number of times we want to replicate the original_list
. The replicated_list
is created by multiplying original_list
by n
using the ‘*’ operator.
Example :
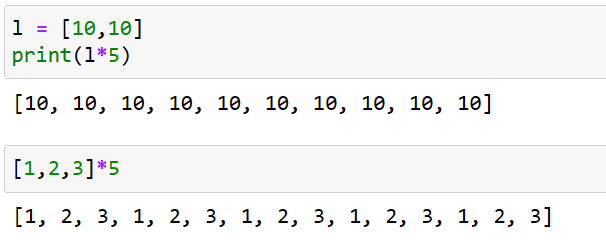
Removing values from lists with del statement
In Python, you can remove elements from a list using the del
statement. The del
statement is a powerful Python keyword that allows you to delete an object, such as a variable or an element in a list. Here are some examples of removing values from lists using the del
statement:
- Removing an element by index:
my_list = [1, 2, 3, 4, 5]
del my_list[1:4]
print(my_list) # Output: [1, 5]
In the above example, we have a list my_list
with five elements. We use the del
statement to remove the elements at indices 1, 2, and 3, which have the values 2, 3, and 4. We do this by using slicing notation [1:4]
, which selects the elements at indices 1, 2, and 3. After deleting the elements, the list contains the remaining elements [1, 5]
.
2. Removing multiple elements by slicing:
my_list = [1, 2, 3, 4, 5]
del my_list[1:4]
print(my_list) # Output: [1, 5]
In the above example, we have a list my_list
with five elements. We use the del
statement to remove the elements at indices 1, 2, and 3, which have the values 2, 3, and 4. We do this by using slicing notation [1:4]
, which selects the elements at indices 1, 2, and 3. After deleting the elements, the list contains the remaining elements [1, 5]
.
3. Removing all elements:
my_list = [1, 2, 3, 4, 5]
del my_list[:]
print(my_list) # Output: []
In the above example, we have a list my_list
with five elements. We use the del
statement to remove all elements of the list by slicing the entire list with [:]
. After deleting all elements, the list is empty, []
.
Note that the del
statement permanently deletes the elements from the list, so you should be careful when using it. Make sure you have a copy of the original list or are sure that you no longer need the deleted elements.
Example :
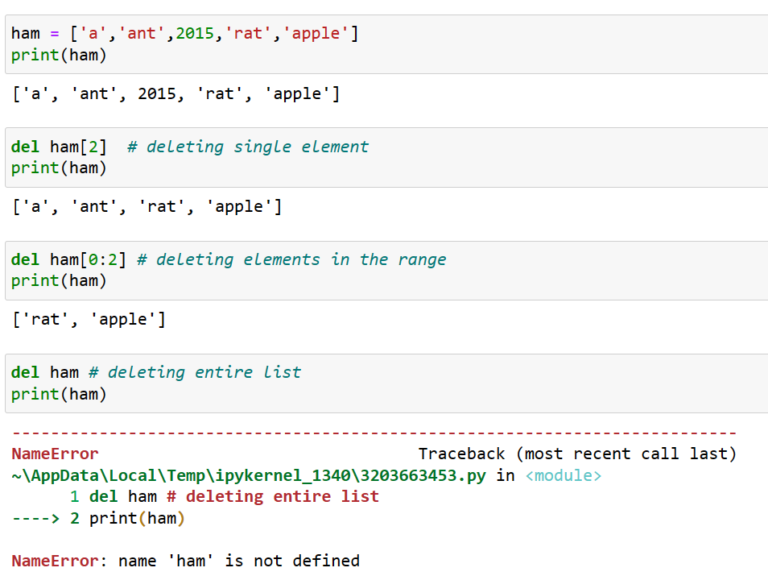
Example :
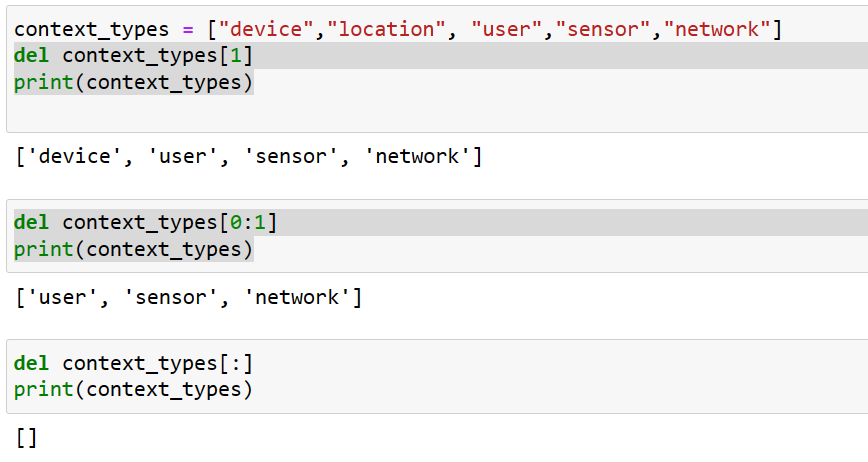
Example :
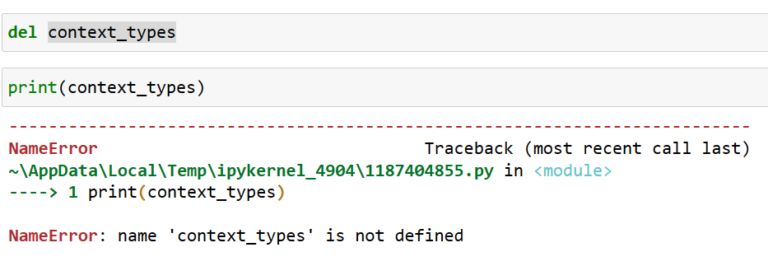
Accessing List values (Getting individual values in lists with indexes.)
One can think List as a relationship between indices and elements. This relationship is called a mapping. Each index ‘maps to’, one of the elements. Python Supports both positive and negative indexing of list elements as illustrated below.
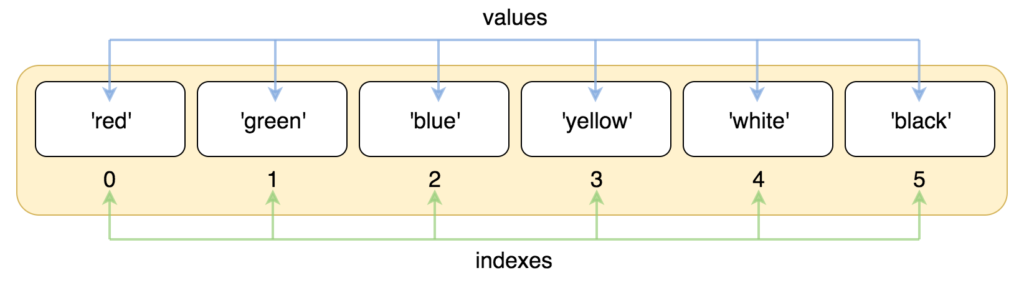
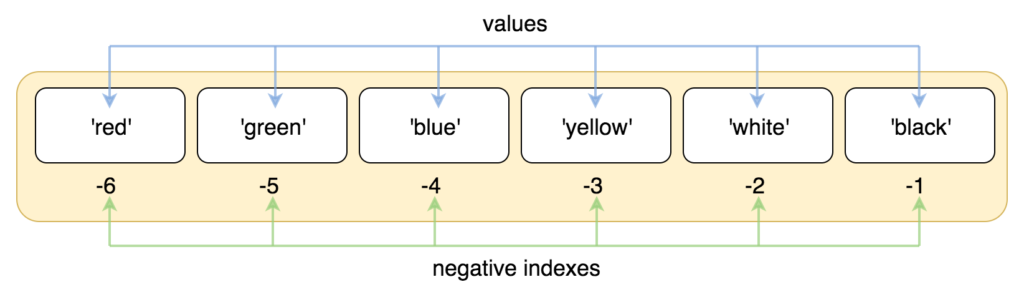
Positive indexing starts from 0 and goes up to n-1, where n is the length of the list. For example:
my_list = [10, 20, 30, 40]
print(my_list[0]) # Output: 10
print(my_list[1]) # Output: 20
print(my_list[2]) # Output: 30
print(my_list[3]) # Output: 40
Negative indexing starts from -1 and goes down to -n, where n is the length of the list. For example:
my_list = [10, 20, 30, 40]
print(my_list[-1]) # Output: 40
print(my_list[-2]) # Output: 30
print(my_list[-3]) # Output: 20
print(my_list[-4]) # Output: 10
Here are some examples that demonstrate the use of positive and negative indexing in Python lists of integers:
my_list = [10, 20, 30, 40, 50]
# Accessing elements using positive indexing
print(my_list[0]) # Output: 10
print(my_list[2]) # Output: 30
# Accessing elements using negative indexing
print(my_list[-1]) # Output: 50
print(my_list[-3]) # Output: 30
# Modifying elements using positive indexing
my_list[1] = 25
print(my_list) # Output: [10, 25, 30, 40, 50]
# Modifying elements using negative indexing
my_list[-2] = 45
print(my_list) # Output: [10, 25, 30, 45, 50]
# Slicing using positive indexing
print(my_list[1:3]) # Output: [25, 30]
# Slicing using negative indexing
print(my_list[-3:-1]) # Output: [30, 45]
In summary, positive indexing starts from 0 and goes up to n-1, while negative indexing starts from -1 and goes down to -n. Positive indexing is used to access or modify elements, while negative indexing is used to access elements from the end of the list. Slicing can be done using both positive and negative indexing.
Example : Accessing through Positive indexes

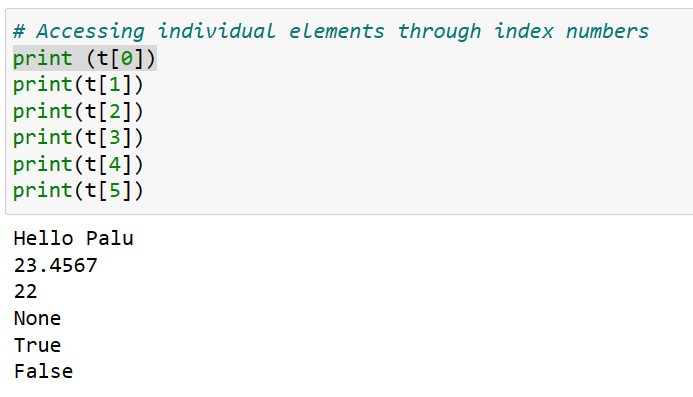
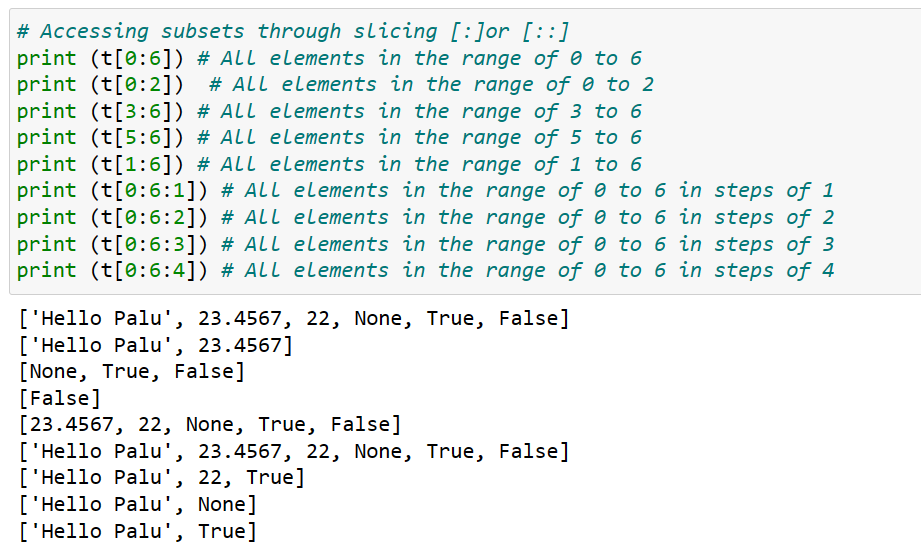
Example : Accessing through Negative indexes
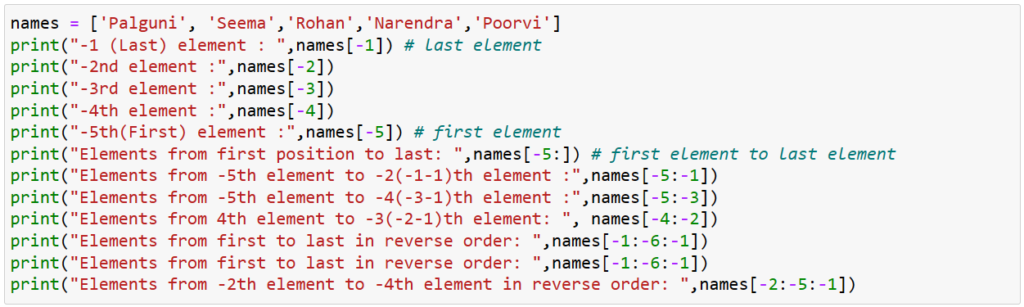
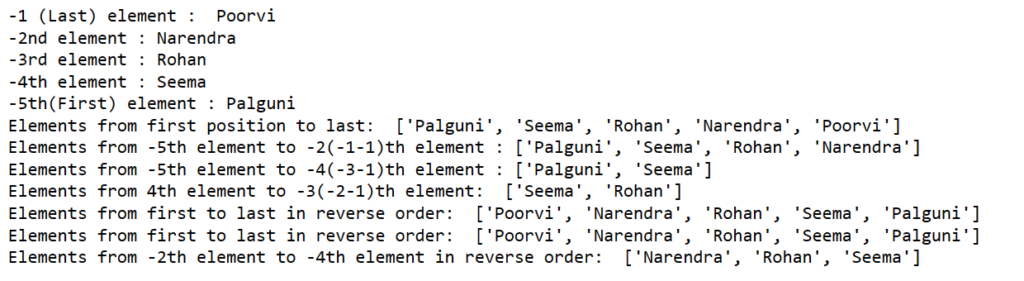
Index Error
An Index Error occurs when you try to access an element in a list using an invalid index, which means the index is either negative, greater than or equal to the length of the list.
Here is an example:
my_list = ['apple', 'banana', 'orange']
print(my_list[3])
In this example, the list my_list
has three elements, with indices 0, 1, and 2. The above code tries to access the element at index 3, which is out of range and will result in an Index Error. The correct way to access the last element of this list would be to use index 2, like this:
print(my_list[2])
This will print the last element of the list, which is ‘orange’.
Example :
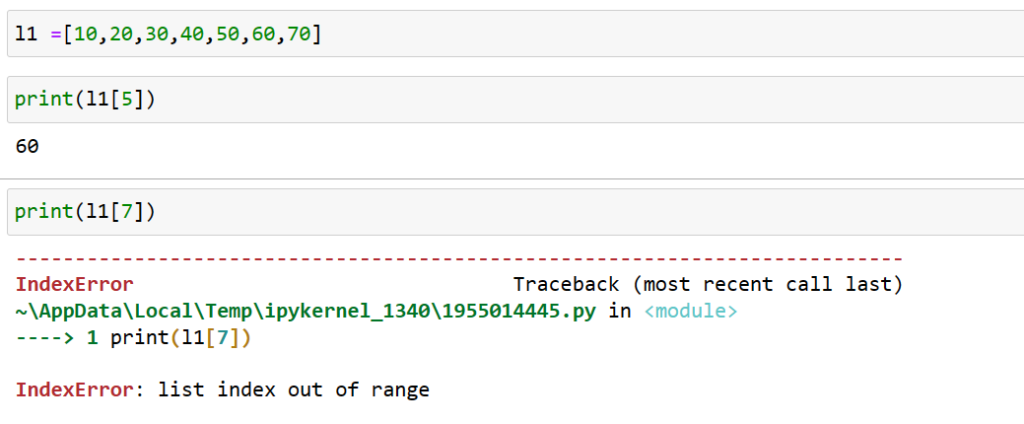
Traversing a list using for Loop
The most common way to traverse the elements of a list is with a for loop. The syntax and example is as illustrated in the example below
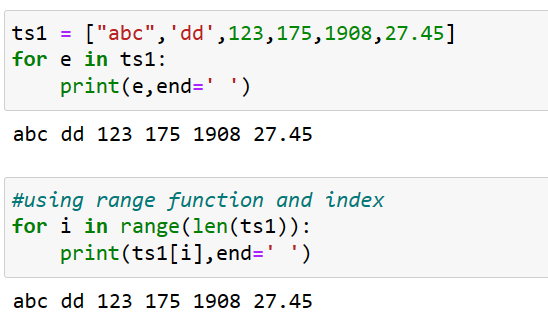
This loop traverses the list and updates each element. len returns the number of elements in the list. range returns a list of indices from 0 to n − 1, where n is the length of the list.
Note 1 : A for loop over an empty list never executes the body.
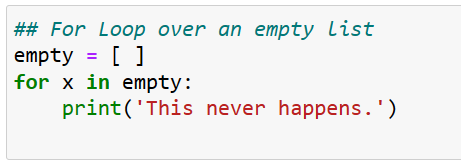
Note 2 : Although a list can contain another list, the nested list still counts as a single element. The length of this list is four:

In Python, a nested list is a list that contains other lists as elements. In other words, a nested list is a list within a list. You can have multiple levels of nesting, which means a list can contain other lists, which in turn can contain more lists and so on.
Here is an example of a simple nested list:
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
In this example, my_list
is a list that contains three other lists as its elements. Each of the inner lists contains three integers.
You can access the elements of a nested list using indexing. For example, to access the element at the first row and second column of my_list
, you would use the following code:
my_list[0][1] # Output: 2
Here, my_list[0]
returns the first list in the nested list, and my_list[0][1]
returns the second element of that list.
You can also use a nested loop to iterate over the elements of a nested list. For example:
for row in my_list:
for item in row:
print(item)
This code will print all the elements of my_list
in order, one by one.
List Slicing /Getting Sublists with Slices
In Python, list slicing is a way to extract a subset of elements from a list. Slicing allows you to extract a contiguous portion of a list, specified by its starting and ending indices. The syntax for slicing a list is as follows:
my_list[start:end:step]
Here, start
is the index of the first element to include in the slice, end
is the index of the first element to exclude from the slice, and step
is the number of elements to skip between each element in the slice. Note that the end
index is exclusive, which means that the element at the end
index is not included in the slice.
Here are some examples of list slicing:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Extract a slice of the first 5 elements
slice1 = my_list[0:5]
print(slice1) # Output: [1, 2, 3, 4, 5]
# Extract a slice of the elements at odd indices
slice2 = my_list[1::2]
print(slice2) # Output: [2, 4, 6, 8, 10]
# Extract a slice of the elements in reverse order
slice3 = my_list[::-1]
print(slice3) # Output: [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
In the first example, we extract a slice of the first 5 elements of my_list
. In the second example, we extract a slice of the elements at odd indices, starting from the second element. In the third example, we extract a slice of all the elements in reverse order.
Note that you can also use negative indices for start
and end
. In this case, the index is counted from the end of the list, with -1
being the index of the last element. For example:
# Extract a slice of the last 3 elements
slice4 = my_list[-3:]
print(slice4) # Output: [8, 9, 10]
This example extracts a slice of the last 3 elements of my_list
.
Examples :
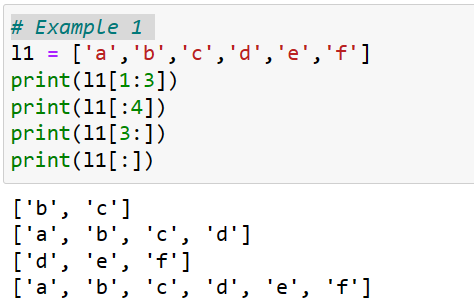

Usage of in and not in membership operators in list
In Python, the in
and not in
operators are used to check if an element is present in a list or not. These operators return a boolean value (True
or False
) depending on whether the element is found in the list or not.
The in
operator checks if an element is present in a list, and returns True
if it is, and False
otherwise. Here is an example:
my_list = ['apple', 'banana', 'orange']
print('banana' in my_list) # Output: True
print('grape' in my_list) # Output: False
In this example, the first print statement checks if the string ‘banana’ is present in the list my_list
, which it is, so it returns True
. The second print statement checks if the string ‘grape’ is present in the list my_list
, which it is not, so it returns False
.
The not in
operator does the opposite of in
. It checks if an element is not present in a list, and returns True
if it is not, and False
otherwise. Here is an example:
my_list = ['apple', 'banana', 'orange']
print('banana' not in my_list) # Output: False
print('grape' not in my_list) # Output:
In this example, the first print statement checks if the string ‘banana’ is not present in the list my_list
, which it is, so it returns False
. The second print statement checks if the string ‘grape’ is not present in the list my_list
, which it is not, so it returns True
.
The in
and not in
operators can be used with other data types as well, such as strings, tuples, and sets, in addition to lists.
Multiple Assignment
In Python, multiple assignment is a feature that allows you to assign multiple values to multiple variables in a single statement. This can be useful when you have multiple values that you want to assign to multiple variables without having to write separate statements for each assignment.
The syntax for multiple assignment is as follows:
a, b, c = 1, 2, 3
In this example, the values 1
, 2
, and 3
are assigned to the variables a
, b
, and c
, respectively. This is equivalent to the following three separate assignment statements:
a = 1
b = 2
c = 3
You can also use multiple assignment to swap the values of two variables without using a temporary variable, like this:
a, b = b, a
In this example, the values of a
and b
are swapped.
Multiple assignment can also be used with lists, tuples, or any other iterable object. For example:
my_list = [1, 2, 3]
a, b, c = my_list
In this example, the values of my_list
are unpacked and assigned to the variables a
, b
, and c
, respectively.
Note that the number of variables on the left-hand side of the assignment must match the number of values on the right-hand side of the assignment, otherwise you will get a ValueError
exception.
Example :
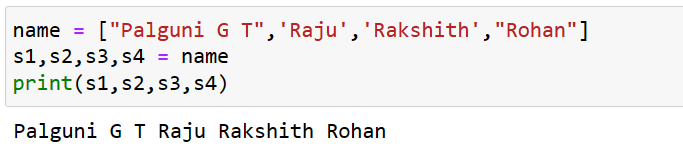
Example :
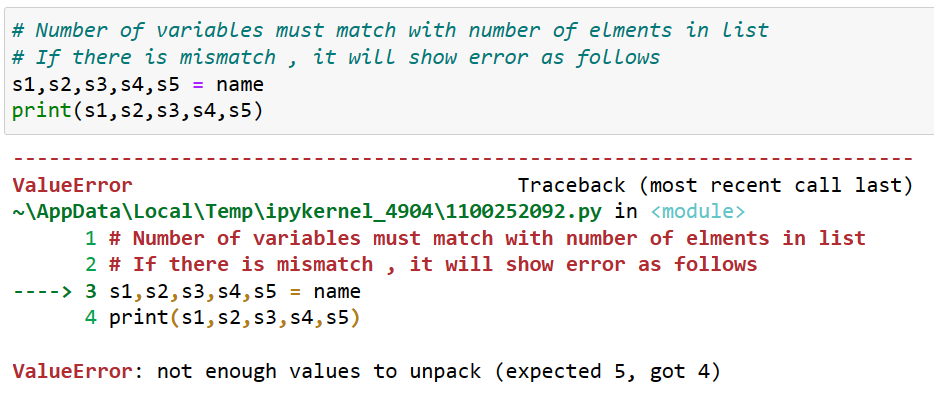
Example :

List Methods
The list data type has several useful methods for finding , adding removing and otherwise manipulating values in a list. Some of the list methods are :
append()
– Adds an element to the end of the listextend()
– Adds one or more elements of an iterable to the end of the listinsert()
– Inserts an element at a specified position in the listremove()
– Removes the first occurrence of an element from the listpop()
– Removes and returns the element at a specified position in the listindex()
– Returns the index of the first occurrence of an element in the listcount()
– Returns the number of times an element appears in the listsort()
– Sorts the elements of the list in ascending orderreverse()
– Reverses the order of the elements in the listcopy()
– Returns a shallow copy of the listclear()
– Removes all elements from the list
append()
append()
is a built-in method in Python’s list class that adds a new element to the end of the list. The syntax for using append()
is straightforward:
list_name.append(element)
Here, list_name
refers to the name of the list you want to modify, and element
is the value you want to append to the list.
Here’s an example that demonstrates the use of append()
:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
In this example, we start with a list [1, 2, 3]
, and then use append()
to add the value 4
to the end of the list. The output shows that the list now contains the elements [1, 2, 3, 4]
.
Note that append()
only adds a single element to the end of the list. If you want to add multiple elements to a list, you can use the extend()
method instead.
Also, it’s worth noting that append()
modifies the original list in place, rather than creating a new list. This means that any references to the original list will also reflect the changes made by append()
.
Example :
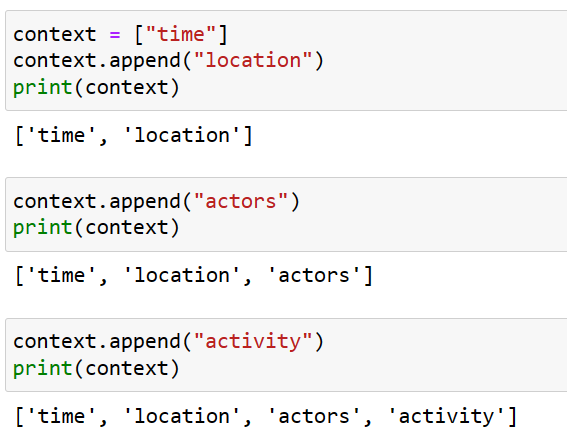
extend()
extend()
is a built-in method in Python’s list class that appends the elements of an iterable to the end of an existing list. The syntax for using extend()
is as follows:
list_name.extend(iterable)
Here, list_name
refers to the name of the list you want to modify, and iterable
is any iterable object, such as a list, tuple, or string, that contains the elements you want to add to the end of the list.
Here’s an example that demonstrates the use of extend()
:
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
In this example, we start with a list [1, 2, 3]
, and then use extend()
to add the elements [4, 5, 6]
to the end of the list. The output shows that the list now contains the elements [1, 2, 3, 4, 5, 6]
.
Note that extend()
can add multiple elements to a list at once, and can be used to extend a list with elements from another list or any other iterable object.
It’s also worth noting that extend()
modifies the original list in place, rather than creating a new list. This means that any references to the original list will also reflect the changes made by extend()
.
insert()
insert()
is a built-in method in Python’s list class that allows you to insert a new element at a specific position in a list. The syntax for using insert()
is as follows:
list_name.insert(index, element)
Here, list_name
refers to the name of the list you want to modify, index
is the position in the list where you want to insert the new element, and element
is the value you want to insert.
Here’s an example that demonstrates the use of insert()
:
my_list = [1, 2, 3]
my_list.insert(1, 4)
print(my_list) # Output: [1, 4, 2, 3]
In this example, we start with a list [1, 2, 3]
, and then use insert()
to add the value 4
at index 1 (i.e., between the values 1 and 2) in the list. The output shows that the list now contains the elements [1, 4, 2, 3]
.
Note that insert()
modifies the original list in place, rather than creating a new list. This means that any references to the original list will also reflect the changes made by insert()
. Also, if you try to insert an element at an index that is outside the range of the list, Python will raise an IndexError
exception.
Example :
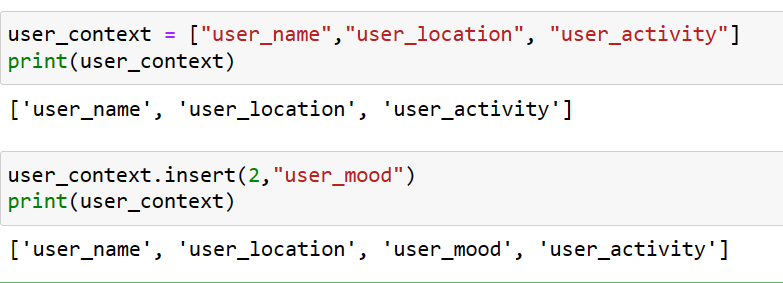
remove()
The remove()
method is a built-in function in Python lists that is used to remove the first occurrence of a specified value from the list. It takes one argument, which is the value to be removed from the list.
Here is the syntax for the remove()
method:
list.remove(value)
where list
is the name of the list and value
is the value to be removed from the list.
Examples:
- Removing an element from a list
fruits = ["apple", "banana", "cherry", "banana"]
fruits.remove("banana")
print(fruits) # Output: ["apple", "cherry", "banana"]
In the above example, the first occurrence of “banana” is removed from the list.
- Removing an element that is not present in the list
fruits = ["apple", "banana", "cherry"]
fruits.remove("mango") # Raises ValueError: list.remove(x): x not in list
In the above example, since “mango” is not present in the list, remove()
method raises a ValueError.
Note that if there are multiple occurrences of the specified value in the list, only the first occurrence will be removed. If the value is not present in the list, a ValueError will be raised.
Example :
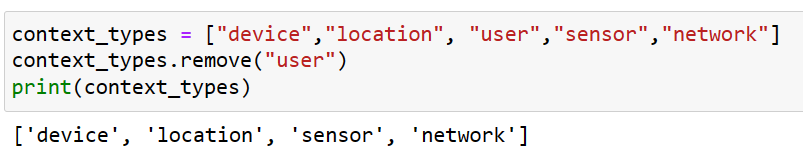
pop()
The pop()
method in Python is a built-in function in lists that removes and returns the element at the specified index. If no index is specified, it removes and returns the last element in the list.
Here is the syntax for the pop()
method:
list.pop([index])
where list
is the name of the list and index
is the index of the element to be removed. The index
parameter is optional. If it is not specified, then pop()
removes and returns the last element of the list.
Examples:
- Removing the last element of a list:
fruits = ["apple", "banana", "cherry"]
last_fruit = fruits.pop()
print(last_fruit) # Output: "cherry"
print(fruits) # Output: ["apple", "banana"]
In the above example, pop()
removes and returns the last element “cherry” from the list. The last_fruit
variable stores the returned value. The updated list after removing the last element is ["apple", "banana"]
.
2. Removing an element at a specific index:
fruits = ["apple", "banana", "cherry"]
second_fruit = fruits.pop(1)
print(second_fruit) # Output: "banana"
print(fruits) # Output: ["apple", "cherry"]
In the above example, pop(1)
removes and returns the element at index 1, which is “banana”. The second_fruit
variable stores the returned value. The updated list after removing the element at index 1 is ["apple", "cherry"]
.
- Removing an element from an empty list:
fruits = []
fruit = fruits.pop() # Raises IndexError: pop from empty list
In the above example, since the list fruits
is empty, calling pop()
method raises an IndexError because there is no element to remove from the list.
Example :
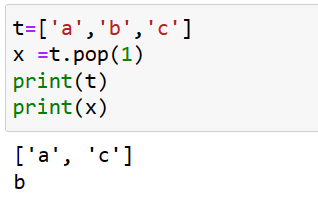
index()
The index()
method is a built-in function in Python lists that returns the index of the first occurrence of a specified element in the list. If the specified element is not in the list, it raises a ValueError.
Here is the syntax for the index()
method:
list.index(element[, start[, end]])
where list
is the name of the list, element
is the element to be searched, start
(optional) is the starting index of the search, and end
(optional) is the ending index of the search.
Examples:
- Finding the index of an element:
fruits = ["apple", "banana", "cherry"]
banana_index = fruits.index("banana")
print(banana_index) # Output: 1
In the above example, index()
method returns the index of the first occurrence of “banana” in the list, which is 1.
- Finding the index of an element within a specified range:
fruits = ["apple", "banana", "cherry", "banana", "orange"]
banana_index = fruits.index("banana", 2, 4)
print(banana_index) # Output: 3
In the above example, index("banana", 2, 4)
searches for the first occurrence of “banana” between the indices 2 and 4 (exclusive). Since the first occurrence of “banana” within this range is at index 3, that value is returned.
- Finding the index of an element that is not in the list:
fruits = ["apple", "banana", "cherry"]
mango_index = fruits.index("mango") # Raises ValueError: 'mango' is not in list
In the above example, since “mango” is not in the list, calling index()
method raises a ValueError.
Example :
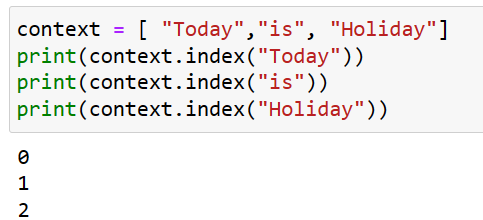
count ()
The count()
method is a built-in function in Python lists that returns the number of times a specified element appears in the list.
Here is the syntax for the count()
method:
list.count(element)
where list
is the name of the list and element
is the element to be counted.
Examples:
- Counting the number of occurrences of an element:
fruits = ["apple", "banana", "cherry", "banana", "orange"]
banana_count = fruits.count("banana")
print(banana_count) # Output: 2
In the above example, count()
method returns the number of occurrences of “banana” in the list, which is 2.
2. Counting the number of occurrences of an element that is not in the list:
fruits = ["apple", "banana", "cherry"]
mango_count = fruits.count("mango")
print(mango_count) # Output: 0
In the above example, count()
method returns 0 since “mango” is not present in the list.
- Counting the number of occurrences of an element in an empty list:
fruits = []
apple_count = fruits.count("apple")
print(apple_count) # Output: 0
In the above example, count()
method returns 0 since the list is empty and “apple” is not present in the list.
sort() method
The sort()
method is a built-in function in Python lists that sorts the elements of a list in ascending or descending order.
Here is the syntax for the sort()
method:
list.sort(key=None, reverse=False)
where list
is the name of the list, key
(optional) is a function that specifies the key to sort by, and reverse
(optional) is a boolean value that determines whether to sort the list in descending order (True) or ascending order (False). The default value of key
is None, which means the elements are compared directly. The default value of reverse
is False.
Examples:
- Sorting a list in ascending order:
fruits = ["banana", "apple", "cherry", "orange"]
fruits.sort()
print(fruits) # Output: ["apple", "banana", "cherry", "orange"]
In the above example, sort()
method sorts the elements of the fruits
list in ascending order.
- Sorting a list in descending order:
fruits = ["banana", "apple", "cherry", "orange"]
fruits.sort(reverse=True)
print(fruits) # Output: ["orange", "cherry", "banana", "apple"]
In the above example, sort(reverse=True)
sorts the elements of the fruits
list in descending order.
- Sorting a list of tuples by a specific element:
students = [("Alice", 25), ("Bob", 18), ("Charlie", 22), ("Dave", 30)]
students.sort(key=lambda x: x[1])
print(students) # Output: [("Bob", 18), ("Charlie", 22), ("Alice", 25), ("Dave", 30)]
In the above example, sort(key=lambda x: x[1])
sorts the students
list of tuples by the second element (i.e., age) in ascending order. The lambda function lambda x: x[1]
specifies the key to sort by.
Note: The sort()
method modifies the original list and does not return a new list. If you want to sort a list without modifying the original list, you can use the sorted()
function instead.
Example :
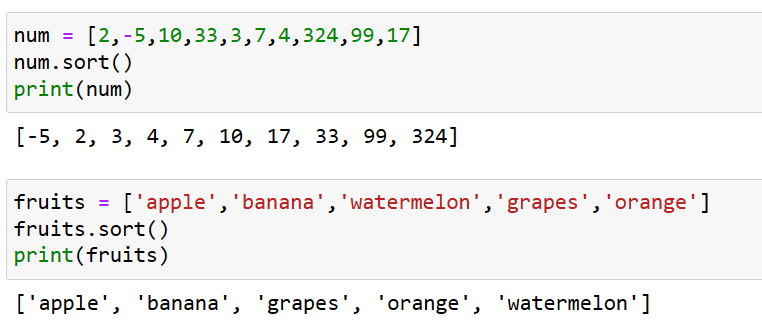
Example :
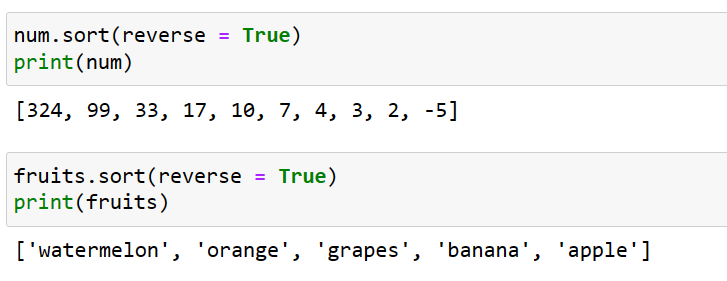
reverse() method
The reverse()
method is a built-in function in Python lists that reverses the order of the elements in a list.
Here is the syntax for the reverse()
method:
list.reverse()
where list
is the name of the list.
Examples:
- Reversing a list:
fruits = ["apple", "banana", "cherry", "orange"]
fruits.reverse()
print(fruits) # Output: ["orange", "cherry", "banana", "apple"]
In the above example, reverse()
method reverses the order of the elements in the fruits
list.
- Reversing an empty list:
fruits = []
fruits.reverse()
print(fruits) # Output: []
In the above example, reverse()
method does not modify an empty list because there are no elements to reverse.
Note: The reverse()
method modifies the original list and does not return a new list. If you want to reverse a list without modifying the original list, you can use the slicing operator [::-1]
instead.
copy() method
The copy()
method is a built-in function in Python lists that returns a shallow copy of the list.
Here is the syntax for the copy()
method:
new_list = list.copy()
where list
is the name of the list that you want to copy and new_list
is the new list that is created as a copy of the original list.
Examples:
- Creating a shallow copy of a list:
fruits = ["apple", "banana", "cherry", "orange"]
fruits_copy = fruits.copy()
print(fruits_copy) # Output: ["apple", "banana", "cherry", "orange"]
In the above example, copy()
method creates a shallow copy of the fruits
list and assigns it to fruits_copy
.
- Modifying the original list does not affect the copied list:
fruits = ["apple", "banana", "cherry", "orange"]
fruits_copy = fruits.copy()
fruits.pop()
print(fruits) # Output: ["apple", "banana", "cherry"]
print(fruits_copy) # Output: ["apple", "banana", "cherry", "orange"]
In the above example, copy()
method creates a shallow copy of the fruits
list and assigns it to fruits_copy
. The pop()
method removes the last element of the fruits
list. However, the fruits_copy
list remains unchanged because it is a copy of the original fruits
list.
clear()
The clear()
method is a built-in function in Python lists that removes all elements from the list. Here is the syntax for the clear()
method:
list.clear()
where list
is the name of the list that you want to clear.
Examples:
- Clearing a list:
fruits = ["apple", "banana", "cherry", "orange"]
fruits.clear()
print(fruits) # Output: []
In the above example, clear()
method removes all elements from the fruits
list.
- Clearing an empty list:
fruits = []
fruits.clear()
print(fruits) # Output: []
In the above example, clear()
method does not modify an empty list because there are no elements to remove.
Lists and Functions
There are a number of built in functions that can be used on lists that allow you to quickly look through a list without writing your own loops. Some of the functions used with lists are len(), max(), min(),sum(),etc. The sum() function only works when the list elements are number . The other functions max(), len(),etc., work with lists of strings and other types that can be comparable.
# len(): Returns the length of a list.
my_list = [1, 2, 3, 4, 5]
print(len(my_list)) # Output: 5
# min(): Returns the minimum value of a list (only if all elements are of the same type).
my_list = [5, 2, 8, 1, 9]
print(min(my_list)) # Output: 1
#max(): Returns the maximum value of a list (only if all elements are of the same type).
my_list = [5, 2, 8, 1, 9]
print(max(my_list)) # Output: 9
# sum(): Returns the sum of all elements in a list (only if all elements are numeric).
my_list = [1, 2, 3, 4, 5]
print(sum(my_list)) # Output: 15
# sorted(): Returns a sorted list.
my_list = [5, 2, 8, 1, 9]
sorted_list = sorted(my_list)
print(sorted_list) # Output: [1, 2, 5, 8, 9]
# reversed(): Returns a reversed list.
my_list = [1, 2, 3, 4, 5]
reversed_list = list(reversed(my_list))
print(reversed_list) # Output: [5, 4, 3, 2, 1]
# list(): Converts an iterable object into a list.
my_tuple = (1, 2, 3, 4, 5)
my_list = list(my_tuple)
print(my_list) # Output: [1, 2, 3, 4, 5]
Python program to compute average of numbers without using list.
# Initialize variables
sum_of_numbers = 0
count_of_numbers = 0
# Keep taking input until the user enters 'done'
while True:
num = input("Enter a number (or 'done' to exit): ")
if num == 'done':
break
sum_of_numbers += int(num)
count_of_numbers += 1
# Compute the average
if count_of_numbers > 0:
average = sum_of_numbers / count_of_numbers
print("Average:", average)
else:
print("No numbers entered.")
Python program to compute average of numbers using list.
# Take input from the user and store it in a list
numbers = []
while True:
num = input("Enter a number (or 'done' to exit): ")
if num == 'done':
break
numbers.append(int(num))
# Compute the average
if len(numbers) > 0:
average = sum(numbers) / len(numbers)
print("Average:", average)
else:
print("No numbers entered.")
Example : For a given list num = [45,22,14,65,97,72] write a python program to replace all the integers divisible by 3 with”ppp” and all integers divisible by 5 with “qqq” and replace all the integers divisible by both 3 and 5 with” pppqqq” and display the output.
num = [45, 22, 14, 65, 97, 72]
# Iterate over the list and replace the numbers
for i in range(len(num)):
if num[i] % 3 == 0 and num[i] % 5 == 0:
num[i] = "pppqqq"
elif num[i] % 3 == 0:
num[i] = "ppp"
elif num[i] % 5 == 0:
num[i] = "qqq"
# Print the modified list
print(num)
Output :
['pppqqq', 22, 14, 'qqq', 97, 'ppp']
Copy and Deep Copy
In Python, copy()
and deepcopy()
are two methods that can be used to create copies of lists (and other objects). The main difference between them is how they handle nested objects (e.g., lists or dictionaries) inside the list being copied.
The copy()
method creates a new list that is a shallow copy of the original list. This means that a new list object is created, but the elements of the new list are references to the same objects as the original list. If the original list contains mutable objects (e.g., lists or dictionaries), changes to these objects in the original list will be reflected in the copied list as well.
On the other hand, the deepcopy()
method creates a completely new list with new objects. This means that even if the original list contains mutable objects, changes to these objects in the original list will not be reflected in the copied list.
Here are some examples that illustrate the differences between copy()
and deepcopy()
:
- Shallow copy using
copy()
method:
list1 = [[1, 2], [3, 4]]
list2 = list1.copy()
print(list1) # Output: [[1, 2], [3, 4]]
print(list2) # Output: [[1, 2], [3, 4]]
list1[0][0] = 5
print(list1) # Output: [[5, 2], [3, 4]]
print(list2) # Output: [[5, 2], [3, 4]]
In the above example, list1
is a nested list with two inner lists. list2
is a shallow copy of list1
using the copy()
method. After modifying the first element of the first inner list in list1
, the same modification is reflected in list2
because both lists reference the same objects.
- Deep copy using
deepcopy()
method:
import copy
list1 = [[1, 2], [3, 4]]
list2 = copy.deepcopy(list1)
print(list1) # Output: [[1, 2], [3, 4]]
print(list2) # Output: [[1, 2], [3, 4]]
list1[0][0] = 5
print(list1) # Output: [[5, 2], [3, 4]]
print(list2) # Output: [[1, 2], [3, 4]]
In the above example, list1
is a nested list with two inner lists. list2
is a deep copy of list1
using the deepcopy()
method. After modifying the first element of the first inner list in list1
, the same modification is not reflected in list2
because list2
is a completely new list with new objects.
In summary, the copy()
method creates a new list with references to the same objects as the original list, while the deepcopy()
method creates a completely new list with new objects. Use copy()
when you want to create a new list that shares the same objects as the original list, and use deepcopy()
when you want to create a completely new list with new objects.
List comprehension
List comprehension is a concise way to create a new list based on an existing list or iterable. It allows you to write a single line of code to create a new list by applying a function or condition to each element of an existing list. Here’s an example:
Suppose we have a list of numbers:
numbers = [1, 2, 3, 4, 5]
We can use list comprehension to create a new list that contains the squares of these numbers:
squares = [num**2 for num in numbers]
print(squares)
Output:
[1, 4, 9, 16, 25]
In this example, we use list comprehension to create a new list squares
that contains the squares of each number in the original list numbers
. The expression num**2
is applied to each element of the list numbers
, and the resulting values are collected into a new list.
List comprehension can also include conditions. Here’s an example that uses list comprehension to create a new list that contains only the even numbers from the original list:
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers)
Output:
[2, 4]
In this example, we add a condition if num % 2 == 0
to the list comprehension, which filters out any numbers that are not even. Only the even numbers are included in the new list even_numbers
.
List comprehension is a powerful feature in Python that allows you to create new lists quickly and easily, and it can help make your code more concise and readable.
Example :
# Creating a list of squares of numbers from 1 to 10
squares = [x ** 2 for x in range(1, 11)]
print(squares)
# Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
# Creating a list of even numbers from a given list:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers)
# Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
#Creating a list of even numbers from a given list:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers)
# Output: [2, 4, 6, 8, 10]
#Creating a list of common elements from two given lists
list1 = [1, 2, 3, 4, 5]
list2 = [3, 4, 5, 6, 7]
common_elements = [x for x in list1 if x in list2]
print(common_elements)
# Output: [3, 4, 5]
#Creating a list of strings from a list of integers:
numbers = [1, 2, 3, 4, 5]
strings = [str(x) for x in numbers]
print(strings)
#Output: ['1', '2', '3', '4', '5']
# Creating a list of tuples from two lists:
list1 = ['apple', 'banana', 'cherry']
list2 = [1, 2, 3]
result = [(x, y) for x in list1 for y in list2]
print(result)
# Output: [('apple', 1), ('apple', 2), ('apple', 3), ('banana', 1), ('banana', 2),
# ('banana', 3), ('cherry', 1), ('cherry', 2), ('cherry', 3)
Questions for Practice
- What is list ? Explain the concepts of list creation and list slicing with examples.
- Define Tuple, List, Strings and Dictionary in Python.
- Explain why lists are called Mutable.
- Discuss the following List operations and functions with examples :
- Accessing ,Traversing and Slicing the List Elements
- + (concatenation) and * (Repetition)
- append, extend, sort, remove and delete
- split and join
- Explain the methods of list data type in Python for the following operations with suitable code snippets for each.
- a. Adding values to a list
- b. Removing values from a list
- c. Finding a value in a list
- d. Sorting the values in a list
- What is list ? Explain the concept of slicing and indexing with proper examples.
- For a given list num = [45,22,14,65,97,72] write a python program to replace all the integers divisible by 3 with”ppp” and all integers divisible by 5 with “qqq” and replace all the integers divisible by both 3 and 5 with” pppqqq” and display the output.
- What are the different LIST methods supported in python . Illustrate all the methods with an example.
- What is a Python list?
- How is a Python list different from other data types in Python?
- Can a Python list contain elements of different data types?
- What is the difference between indexing and slicing in a Python list?
- How does Python handle out of index errors when working with lists?
- How do you create an empty Python list?
- How do you check if a Python list is empty?
- What is the difference between append() and extend() methods for adding elements to a Python list?
- How does the remove() method work in a Python list?
- How do you use the pop() method in a Python list?
- How do you find the index of an element in a Python list?
- How do you count the occurrences of an element in a Python list?
- What is a nested Python list?
- How do you flatten a nested Python list?
- How do you loop through a nested Python list?
- What is a list comprehension in Python?
- How do you filter elements in a Python list using list comprehension?
- How do you use map() and filter() functions with a Python list?
- How do you sort a Python list in descending order?
- How do you remove duplicates from a Python list?
- Write a Python program to create a list of numbers and print the sum of all the elements in the list.
- Write a Python program to create a list of strings and print the longest string in the list.
- Write a Python program to create a list of numbers and remove all the even numbers from the list.
- Write a Python program to create a list of numbers and print the second highest number in the list.
- Write a Python program to create a list of strings and sort the list in alphabetical order.
- Write a Python program to create a list of numbers and find the average of all the elements in the list.
- Write a Python program to create a list of numbers and print the median of the list.
- Write a Python program to create a list of strings and count the number of words in the list that have more than 5 characters.
- Write a Python program to create a list of numbers and find the difference between the largest and smallest elements in the list.
- Write a Python program to create a list of numbers and print the elements that are divisible by 3.
- Write a Python program to create a list of numbers and remove all the elements that are less than 5.
- Write a Python program to create a list of numbers and print the elements that occur more than once in the list.
- Write a Python program to create a list of numbers and sort the list in descending order.
- Write a Python program to create a list of strings and print all the strings that start with the letter “A”.
- Write a Python program to create a list of numbers and find the second smallest number in the list.
- Write a Python program to create a nested list and print all the elements in the list.
- Write a Python program to create a list of strings and use list comprehension to create a new list with only the strings that contain the letter “a”.
- Write a Python program to create a list of numbers and use map() function to add 5 to each element in the list.
- Write a Python program to create a list of numbers and use filter() function to remove all the odd numbers from the list.
- Write a Python program to create a list of numbers and remove all the duplicate elements from the list.
- Write a Python program to create a list of numbers and find the sum of all the elements at even indices.
- Write a Python program to create a list of strings and remove all the strings that contain the letter “e”.
- Write a Python program to create a list of numbers and find the product of all the elements in the list.
- Write a Python program to create a list of strings and print the strings that are palindromes.
- Write a Python program to create a list of numbers and remove all the elements that are not prime numbers.
- Write a Python program to create a list of numbers and find the largest prime number in the list.
- Write a Python program to create a list of strings and print the strings that are anagrams of each other.
- Write a Python program to create a list of numbers and find the kth smallest number in the list.
- Write a Python program to create a list of strings and print the strings that are in title case.
- Write a Python program to create a list of numbers and find the sum of all the elements that are divisible by 3 or 5.
- Write a Python program to create a list of strings and remove all the strings that have more than 3 vowels.
- Write a Python program to create a list of numbers and print the elements that are greater than the average of all the elements in the list.
- Write a Python program to create a list of strings and print the strings that contain both vowels and consonants.
- Write a Python program to create a list of numbers and find the element that appears the most number of times in the list.
- Write a Python program to create a list of strings and print the strings that are in uppercase.
- Write a Python program to create a list of numbers and find the difference between the sum of even numbers and the sum of odd numbers in the list.
- Write a Python program to create a list of strings and remove all the strings that have a length less than 3.
- Write a Python program to create a list of numbers and find the second highest even number in the list.
- Write a Python program to create a list of strings and print the strings that have a length that is a multiple of 3.
- Write a Python program to create a list of numbers and find the number of times the sum of adjacent elements is even in the list.