Authors : Dr.Thyagaraju G S and Palguni G T
Elements of Flow Control:
A programs control flow is the order in which the program’s code executes. The control flow of a python program is regulated by conditional statements, loops, and function calls. Real strength of program is not just running or executing instructions sequentially one after the other, but skipping and iterating instructions based on the conditions. Flow control statements can decide which Python instructions to execute under which conditions.
The control and flow of a program in any programming language can be broadly classified into three categories:
- Sequential
- Selection or Branching
- Iteration or looping
The statements in Python are executed sequentially one after the other from the beginning of the program to the end of a program. This kind of program execution where in the control flows sequentially is termed as sequential execution of program.
In some context it becomes inevitable to skip certain set of statements in order to execute another set of statements. This type of program execution is termed as selective execution. In Python language the selective execution is achieved using the statements known as Branching statements or Conditional control structures or statements.
Also, in certain situations a set of statements has to be executed repeatedly for given number of times. This is achieved with the help of Loop Control constructs or statements. The mechanism of repeating one or more statements execution either for a fixed number of times or until certain condition is satisfied is termed as Looping.
Flow control statements often starts with condition followed by a block of code called clause.
Conditions:
Conditions are Boolean expressions with the boolean values True or False. Flow control statements decides what to do based on the condition whether it is true or false.
Blocks of Code /Clause:
The set of more than one statements grouped with same indentation so that they are syntactically equivalent to a single statement is known as Block or Compound Statement. One can tell when a block begins and ends based on indentation of the statements . Following are three rules for blocks :
- Blocks begin when the indentation increases
- Blocks can have nested blocks
- Blocks end when the indentation decreases to zero
Example1 :
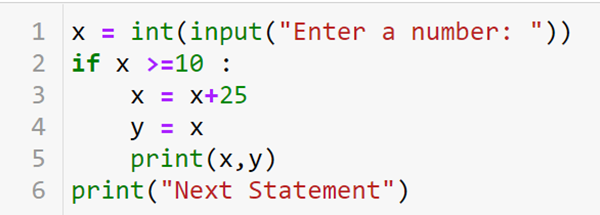
Example 2: Nested Blocks
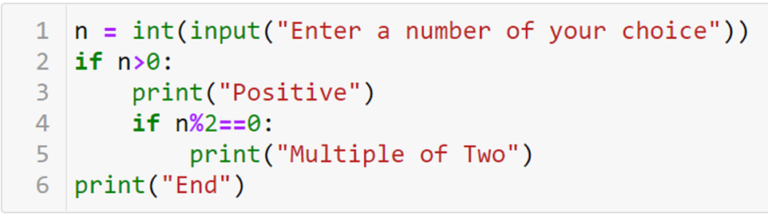
Types of Condition Control Statements
The different two-way selection statements supported by Python language are:
- if statement
- if- else statement
- Nested if else statement
- if – elif ladder
- if statement
In Python, the if
statement is used for conditional execution. It allows you to specify a block of code to be executed if a certain condition is met.
It is basically a two way decision statement and it is used in conjunction with an expression. It is used to execute a set of statements if the condition is true. If the condition is false it skips executing those set of statements. Here is the basic syntax of the if
statement:
if condition:
# code to be executed if the condition is True
The condition in the if
statement can be any expression that evaluates to a boolean value (True
or False
). If the condition is True
, the code block following the if
statement will be executed. If the condition is False
, the code block will be skipped.
Here is an example of using the if
statement:
x = 5
if x > 0:
print("x is positive")
In this example, the condition in the if
statement is x > 0
. Since x
has a value of 5
, which is greater than 0
, the condition is True
, and the code block following the if
statement will be executed. The output of this code will be "x is positive"
.
Example1: Python program to find the largest number using if statement.
numbers = [10, 25, 5, 45, 55, 35]
largest = numbers[0] # initialize largest to the first element in the list
for num in numbers:
if num > largest:
largest = num
print("The largest number is:", largest)
##Output
The largest number is: 55
In this program, we have a list of numbers numbers
, and we initialize the variable largest
to the first number in the list. We then iterate through the rest of the numbers in the list using a for
loop and use an if
statement to check if the current number is larger than the current value of largest
. If it is, we update the value of largest
. Finally, we print out the value of largest
after the loop has finished executing.
num1 = int(input("Enter the first number: "))
num2 = int(input("Enter the second number: "))
largest = num1
if num2 > largest:
largest = num2
print("The largest number is:", largest)
Output :
Enter the first number: 10
Enter the second number: 20
The largest number is: 20
In this program, we use the input()
function to accept two numbers as input from the user and convert them to integers using the int()
function. We then initialize largest
to num1
. We then use an if
statement to check if num2
is greater than largest
. If it is, we set largest
to num2
. Finally, we print out the value of largest
.
This program will prompt the user to enter the two numbers and output the largest of the two.
age = int(input("Enter your age: "))
if age >= 18:
print("You are eligible to vote.")
print("Thank you for checking eligibility.")
###
Enter your age: 22
You are eligible to vote.
Thank you for checking eligibility
2. if else statement
The if-else
statement in Python allows us to execute a block of code if a condition is true and a different block of code if the condition is false. Here’s the syntax of the if-else
statement in Python:
if condition:
# execute this block of code if the condition is true
else:
# execute this block of code if the condition is false
The condition
can be any expression that evaluates to a boolean value (True
or False
). If the condition is true, then the code in the first block is executed, otherwise the code in the second block is executed. Here’s an example of using the if-else
statement in Python:
x = 5
if x > 10:
print("x is greater than 10")
else:
print("x is less than or equal to 10")
# output :
x is less than or equal to 10
Here’s another example that demonstrates the use of the if-else
statement in Python
x = 15
if x % 2 == 0:
print("x is even")
else:
print("x is odd")
## Ouput
x is odd
In this example, we first set the value of x
to 15
. We then use an if-else
statement to check if x
is even or odd. We do this by checking if the remainder of x
divided by 2
is equal to 0
. Since x
is odd and the remainder is not equal to 0
, the condition is false and the code in the second block is executed.
Here’s an example Python program that checks whether a given number is even or odd using if-else
statement:
num = int(input("Enter a number: "))
if num % 2 == 0:
print(num, "is even.")
else:
print(num, "is odd.")
## output
Enter a number: 8
8 is even.
In this program, we first accept a number as input from the user using the input()
function, and convert it to an integer using the int()
function. We then use an if-else
statement to check if the number is even or odd.
We do this by checking if the remainder of the number divided by 2
is equal to 0
. If it is, then the number is even and we print the message “num is even.” If the remainder is not equal to 0
, then the number is odd and we print the message “num is odd.”
This program will output whether the given number is even or odd.
3. nested if else
In Python, we can use nested if-else
statements to execute code blocks based on multiple conditions. A nested if-else
statement is a statement that contains another if-else
statement within it. Here’s the syntax of the nested if-else
statement in Python:
if condition1:
# execute this block of code if condition1 is true
if condition2:
# execute this block of code if both condition1 and condition2 are true
else:
# execute this block of code if condition1 is true and condition2 is false
else:
# execute this block of code if condition1 is false
Here’s an example of using a nested if-else
statement in Python:
x = 10
y = 5
if x > y:
print("x is greater than y")
if x > 0:
print("x is positive")
else:
print("x is negative")
else:
print("x is less than or equal to y")
## output
x is greater than y
x is positive
In this example, we first set the values of x
and y
. We then use a nested if-else
statement to check if x
is greater than y
, and if so, whether x
is positive or negative. The outer if-else
statement checks if x
is greater than y
. If it is, the inner if-else
statement checks if x
is positive or negative. If x
is greater than 0
, then the code block inside the inner if
statement is executed, which prints “x is positive”. If x
is less than or equal to 0
, then the code block inside the inner else
statement is executed, which prints “x is negative”. If x
is not greater than y
, then the code block inside the outer else
statement is executed, which prints “x is less than or equal to y”.
Here’s an example Python program that uses nested if-else
statements to find the largest of three input numbers:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
num3 = float(input("Enter third number: "))
if num1 >= num2:
if num1 >= num3:
largest = num1
else:
largest = num3
else:
if num2 >= num3:
largest = num2
else:
largest = num3
print("The largest number is", largest)
## output
Enter first number: 6
Enter second number: 9
Enter third number: 3
The largest number is 9.0
In this program, we first accept three numbers as input from the user using the input()
function and convert them to floating-point numbers using the float()
function. We then use nested if-else
statements to compare the three numbers and find the largest among them. First, we check if num1
is greater than or equal to num2
. If it is, we then check if num1
is greater than or equal to num3
. If num1
is greater than or equal to both num2
and num3
, then num1
is the largest among the three. Otherwise, if num3
is greater than or equal to num1
, then num3
is the largest among the three.
If num1
is not greater than or equal to num2
, then we check if num2
is greater than or equal to num3
. If it is, then num2
is the largest among the three. Otherwise, if num3
is greater than or equal to num2
, then num3
is the largest among the three. Finally, we print the value of the largest number using the print()
function. This program will output the largest of the three input numbers.
4. if – elif ladder
Cascaded if elif else is a multipath decision statements which consist of chain of if elseif where in the nesting take place only in else block. As soon as one of the conditions controlling the ‘if’ is true , then statement /statements associated with that ‘if’ are executed and the rest of the ladder is bypassed. If condition is false then it checks the first elif condition , if it is found true then first elif is executed. However, if none of the elif ladder is correct then the final else statement will be executed and control flows from top to down.
In Python, an if-elif
ladder is a way of testing multiple conditions using a series of if
and elif
(short for “else if”) statements. It is useful when you want to test for more than two conditions. The syntax for an if-elif
ladder is:
if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
elif condition3:
# code to execute if condition3 is true
else:
# code to execute if none of the above conditions are true
Here is an example program that uses an if-elif
ladder to determine the grade of a student based on their score:
score = int(input("Enter your score: "))
if score >= 90:
grade = "A"
elif score >= 80:
grade = "B"
elif score >= 70:
grade = "C"
elif score >= 60:
grade = "D"
else:
grade = "F"
print("Your grade is", grade)
## output
Enter your score: 87
Your grade is B
Note that the program will work for any integer score entered by the user. Also note that once a condition is true, the code within that condition is executed and the remaining conditions are not evaluated.
In this program, we first accept the score of the student as input using the input()
function and convert it to an integer using the int()
function.We then use an if-elif
ladder to test the score against multiple conditions.If the score is greater than or equal to 90, the student gets an “A”. If the score is between 80 and 89, the student gets a “B”. If the score is between 70 and 79, the student gets a “C”. If the score is between 60 and 69, the student gets a “D”. If the score is less than 60, the student gets an “F”.Finally, we print the grade of the student using the print()
function.This program will output the grade of the student based on their score.
Looping
Looping, also known as iteration, is a programming concept that allows you to execute a block of code repeatedly until a certain condition is met. It is a fundamental concept in programming that allows you to perform repetitive tasks more efficiently and with less code.
In Python, there are two main types of loops:
for
loop: Afor
loop is used to iterate over a sequence of values, such as a list or a string, for a fixed number of times. The syntax of afor
loop in Python is
for variable in sequence:
# code to execute for each element in sequence
Here is an example of a for
loop that prints the numbers from 1 to 10:
for i in range(1, 11):
print(i)
range()
is a built-in Python function that returns a sequence of numbers. It is commonly used in for
loops to execute a block of code a specific number of times. The syntax of the range()
function is:
range(start, stop, step)
where start
is the starting value of the sequence (inclusive), stop
is the stopping value of the sequence (exclusive), and step
is the step size between the values in the sequence. If start
is not provided, it defaults to 0. If step
is not provided, it defaults to 1.
Here are a few examples of how to use the range()
function:
# prints the numbers from 0 to 9
for i in range(10):
print(i)
# prints the numbers from 1 to 10
for i in range(1, 11):
print(i)
# prints the even numbers from 0 to 10
for i in range(0, 11, 2):
print(i)
In the first example, the range()
function is called with a single argument, 10
, which generates a sequence of numbers from 0 to 9. The for
loop then iterates over this sequence and prints each number.
In the second example, the range()
function is called with two arguments, 1
and 11
, which generates a sequence of numbers from 1 to 10. The for
loop then iterates over this sequence and prints each number.
In the third example, the range()
function is called with three arguments, 0
, 11
, and 2
, which generates a sequence of even numbers from 0 to 10. The for
loop then iterates over this sequence and prints each number.
Note that in Python 2.x, the range()
function returns a list, whereas in Python 3.x, it returns a sequence object that generates the numbers on-the-fly. If you need to create a list from the sequence, you can use the list()
function to convert it to a list:
# creates a list of the numbers from 0 to 9
numbers = list(range(10))
The argument of range() function must be integers. The step parameter can be any positive or negative integer other than zero.
Command | Output |
range(10) | [ 0,1,2,3,4,5,6,7,8,9] |
range(1,11) | [ 1,2,3,4,5,6,7,8,9,10] |
range(0,30,5) | [0,5,10,15,20,25] |
range(0,-9,-1) | [0,-1,-2,-3,-4,-5,-6,-7,-8 |
2. while
loop: A while
loop is used to iterate over a block of code as long as a certain condition is true. The syntax of a while
loop in Python is:
while condition:
# code to execute while condition is true
Here is an example of a while
loop that prints the numbers from 1 to 10:
i = 1
while i <= 10:
print(i)
i += 1
In addition to these two main types of loops, Python provides additional loop constructs, such as the break
and continue
statements.
break
statement: Thebreak
statement is used to exit a loop prematurely. When thebreak
statement is executed inside a loop, the loop is terminated immediately and the control is transferred to the next statement after the loop. Here is an example of afor
loop that exits when the value ofi
is equal to 5:
for i in range(1, 11):
if i == 5:
break
print(i)
4.continue
statement: The continue
statement is used to skip the current iteration of a loop and continue with the next iteration. When the continue
statement is executed inside a loop, the remaining statements in the loop body are skipped for the current iteration and the control is transferred to the next iteration. Here is an example of a for
loop that skips the value of i
when it is equal to 5:
for i in range(1, 11):
if i == 5:
continue
print(i)
Infinite Loop
An infinite loop is a loop that runs indefinitely and never terminates, either because it lacks a condition that causes it to stop, or because that condition is never satisfied. This can cause the program to freeze or crash, and is generally considered a programming error.
A loop becomes infinite loop if a condition never becomes FALSE. You must use caution when using while loops because of the possibility that this condition never resolves to a FALSE value. This results in a loop that never ends. Such a loop is called an infinite loop.
An infinite loop might be useful in client/server programming where the server needs to run continuously so that client programs can communicate with it as and when required.
Infinite loops are generally not desired in most programs, as they can cause the program to freeze or crash. However, there are some rare cases where an infinite loop may be intentionally used in a program. Here are a few examples:
- Server programs: In server programs, the main thread may run an infinite loop to listen for incoming connections and process requests. This loop will only exit when the program is shut down.
- Simulation programs: In simulation programs, an infinite loop may be used to continuously update the simulation and display the results to the user.
- Embedded systems: In embedded systems, an infinite loop may be used to keep the system running continuously and respond to input from sensors or other external devices.
It is important to note that in all these cases, the infinite loop is carefully controlled and designed to terminate in specific circumstances, such as when the program is shut down or when a specific condition is met. Infinite loops that are not carefully designed and controlled can cause the program to become unresponsive or crash.
Here is an example of an infinite loop in Python:
while True:
print("This is an infinite loop!")
In this example, the while
loop is set to run forever, because the condition True
is always true. This will cause the program to continuously print the message “This is an infinite loop!” until it is manually stopped.
Another example of an infinite loop is when the loop variable is never updated, causing the loop to run indefinitely. For instance:
i = 0
while i < 5:
print(i)
In this example, the loop will run forever because the value of i
is never updated inside the loop. The loop condition i < 5
will always be true, and the loop will continue to execute indefinitely.
It is important to be careful when writing loops and ensure that they have a clear termination condition to avoid infinite loops.
Nested Loops
In Python, a nested loop is a loop inside another loop. The outer loop runs first and the inner loop runs inside the outer loop. This process continues until the innermost loop is completed. Here is the basic syntax of a nested loop in Python:
for outer_var in outer_sequence:
# Outer loop statements
for inner_var in inner_sequence:
# Inner loop statements
# More outer loop statements
In this example, the outer_sequence
and inner_sequence
variables represent the sequences being looped over in the outer and inner loops, respectively. The outer_var
and inner_var
variables represent the loop variables for each loop. Here is an example of a nested loop that prints the multiplication table from 1 to 10:
for i in range(1, 11):
for j in range(1, 11):
print(i * j, end="\t")
print()
In this example, the outer loop iterates from 1 to 10, while the inner loop also iterates from 1 to 10. For each value of the outer loop variable i
, the inner loop multiplies i
by each value of the inner loop variable j
and prints the result. The end="\t"
parameter in the print()
statement is used to separate the numbers with tabs, while the second print()
statement is used to print a newline character after each row. Nested loops are a powerful tool in programming and can be used to solve a wide variety of problems, such as searching through two-dimensional arrays or creating complex patterns. However, it is important to be careful when using nested loops, as they can be computationally expensive and slow down your program if not used efficiently.
Here’s an example of nested while loops in Python:
i = 1
while i <= 3:
j = 1
while j <= 3:
print(f"i={i}, j={j}")
j += 1
i += 1
# The output of this code will be:
i=1, j=1
i=1, j=2
i=1, j=3
i=2, j=1
i=2, j=2
i=2, j=3
i=3, j=1
i=3, j=2
i=3, j=3
In this example, we have two while loops. The outer while loop starts with the value of i
as 1 and continues while i
is less than or equal to 3. Inside the outer while loop, we have the inner while loop. The inner while loop starts with the value of j
as 1 and continues while j
is less than or equal to 3.
During each iteration of the inner while loop, it prints the current values of i
and j
. Then, it increments j
by 1. Once the inner while loop finishes, the outer while loop increments i
by 1 and starts the inner while loop again. This process continues until i
is no longer less than or equal to 3.
As you can see, the inner while loop executes three times for each iteration of the outer while loop. This results in a total of 9 print statements being executed.
Jump Statements
In Python, jump statements are used to change the control flow of a program. They allow you to skip over certain parts of the code or repeat certain parts of the code based on certain conditions. The three types of jump statements in Python are break
, continue
, and pass
.
break
Statement:
The break
statement is used to terminate a loop prematurely. When a break
statement is encountered inside a loop, the loop is immediately exited and the program continues executing from the next statement following the loop.
Here’s an example of using break
statement in a while loop:
i = 1
while i <= 10:
if i == 5:
break
print(i)
i += 1
#The output of this code will be:
1
2
3
4
In this example, the loop will run until i
becomes equal to 5. When i
becomes 5, the if
statement is true and the break
statement is executed, terminating the loop prematurely. The output of this code will be
continue
Statement:
The continue
statement is used to skip over certain iterations of a loop. When a continue
statement is encountered inside a loop, the current iteration of the loop is immediately stopped and the loop continues executing from the next iteration.
Here’s an example of using continue
statement in a for loop:
for i in range(1, 6):
if i == 3:
continue
print(i)
#The output of this code will be:
1
2
4
5
In this example, the loop will run for the values of i
between 1 and 5. When i
becomes 3, the if
statement is true and the continue
statement is executed, skipping over the current iteration of the loop.
pass
Statement:
The pass
statement is used as a placeholder when you need to include an empty block of code. It does nothing and simply passes over the current line of code.
Here’s an example of using pass
statement in a function:
def my_function():
pass
In this example, the my_function
function contains no code. The pass
statement is used as a placeholder, indicating that there is no code to be executed in this function.
else statement used with loops
The else
statement can be used with both for
and while
loops in Python. The behavior of the else
statement is slightly different depending on which loop type it is used with.
else
Statement withfor
Loop:
When used with a for
loop, the else
statement is executed after the loop has finished iterating over the sequence. If the for
loop completes all iterations without encountering a break
statement, then the else
statement will be executed. If the for
loop is terminated prematurely by a break
statement, the else
statement will not be executed. Here’s an example of using else
statement with a for
loop:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
else:
print("All numbers have been printed")
In this example, the loop iterates over the list of numbers and prints each number. After the loop has completed, the else
statement is executed and the message “All numbers have been printed” is printed to the console.
2. else
Statement with while
Loop:
When used with a while
loop, the else
statement is executed after the loop has finished executing and the condition specified in the while
loop is no longer true. If the while
loop completes all iterations without encountering a break
statement, then the else
statement will be executed. If the while
loop is terminated prematurely by a break
statement, the else
statement will not be executed.
Here’s an example of using else
statement with a while
loop:
i = 0
while i < 5:
print(i)
i += 1
else:
print("The while loop has ended")
In this example, the while
loop will iterate until the value of i
becomes 5. After each iteration of the loop, the value of i
is incremented by 1 and printed to the console. When the value of i
becomes 5, the condition in the while
loop is no longer true and the else
statement is executed, printing the message “The while loop has ended” to the console.
Note that the else
statement with for
and while
loops in Python is optional and can be omitted if not needed.
Pass Statement
The pass
statement is a simple control statement in Python that does nothing. It is used as a placeholder when a statement is required syntactically, but no action is necessary. It is typically used to indicate that the code block is empty or under development.
The pass statement in Python is used when a statement is required syntactically but you do not want any command or code to execute.The pass statement is a null operation; nothing happens when it executes. The pass is also useful in places where your code will eventually go, but has not been written yet (e.g., in stubs for example):
Here’s an example of using pass
:
if x < 0:
# do something here
else:
pass # nothing to do in the else block
In the above example, the else
block is intentionally left blank because there is nothing to be done when x
is not less than zero. So, the pass
statement is used as a placeholder to indicate that the block does not require any action.
Another example where the pass
statement can be useful is when defining a function that is not yet implemented. For example:
def my_function(arg1, arg2):
pass # to be implemented later
In this example, the my_function
is defined with the pass
statement indicating that the function body has not been implemented yet, and will be filled in later.
In summary, the pass
statement is a useful placeholder when you need to indicate that a block of code is intentionally left blank, or when you’re defining a function that you plan to implement later.
Questions for Practice:
- What is Flow Chart ? Give the meaning of different flow chart symbols.
- Write a Flow chart and Python program for the following:
- To find the average of two numbers.
- To find the factorial of a given number N.
- To find the simple interest given the value of P,T and R
- To find the maximum of two numbersTo find the sum of first 50 natural numbers
- What are operators? Explain the following Operators with example:
- Binary Boolean Operators: and, or & not
- Compare == and = operator.
- What is Flow Control? Explain the different Elements of Flow Control?
- Define Block and explain what nested blocks with example are.
- Define condition control statement. Explain the different types of Condition Control Statement.
- Explain with flow chart and programming example , the following condition control statements :
- if
- if – else
- nested if else
- if – elif ladder
- What is iteration or looping ? Describe the different types of looping statements.
- Explain with syntax , flow chart and programming example the following looping operations.
- While loop
- For loop
- Discuss the working of range() function with programming example.
- Give the output of the following :
- range(10)
- range(1,11)
- range(0,30,5)
- range(0,-9,-1)
- What is infinite loop ? Explain with example.
- What are nested Loops ? Explain with examples.
- Discuss the following with examples :
- break
- continue
- else statement with loop
- pass
- What are Python Modules ? Explain with examples how to import Python Modules .
- What is the difference between break and continue statements.
- What is the purpose of else in loop?
- Write logical expressions for the following :
- Either A is greater than B or A is less than C
- Name is Snehith and age is between 18 and 35.
- Place is either Mysore or Bengaluru but not “Dharwad”.
- Convert the following while loop into for loop :
x =10
while (x<20):
print(x+10)
x+=2
20. Explain while and for loop . Write a program to generate Fibonacci series upto the given limit by defining FIBONACCI(n) function.
21. Mention the advantage of continue statement. Write a program to compute only even numbers sum within the given natural number using continue statement.
22. Demonstrate the use of break and continue keywords in looping structures using a snippet code.
23. With syntax explain the finite and infinite looping constructs in python. What is the break and continue statements .[ VTU June /July 2019]
Programming Questions for Practice:
- Construct a logical expressions to represent each of the following conditions :
- Mark is greater than or equal to 100 but less than 70
- Num is between 0 and 5 but not equal to 2
- Answer is either ‘N’ or ‘n’
- Age is greater than or equal to 18 and gender is male
- City is either ‘Kolkata’ or ‘Mumbai’
- Write a program to check if the number of positive or negative and display an appropriate message.
- Write a program to convert temperature in Fahrenheit to Celsius.
- Write a program to display even numbers between 10 and 20.
- Write a program to perform all the mathematical operations of calculator.
- Write a program to accept a number and display the factorial of that number.
- Write a program to convert binary number to decimal number.
- Write a program to find the sum of the digits of a number.
- Write a program to display prime number between 30.
- Write a program to find the best of two test average marks out of three tests marks accepted from the user.
- Write a program to find the largest of three numbers . [ VTU June /July 2019]
- Write a program to check whether the given year is leap or not. [ VTU June /July 2019]
- Write a program to generate and print prime number between 2 to 50.
- Write a program to find those numbers which are divisible by 7 and multiple of 5 , between 1000 and 3000.
- Write a program to guess a number between 1 to 10.
- Write a program that accepts a word from the user and reverse it.
- Write a program to count the number of even and odd numbers from a series of numbers.
- Write a program that prints all the numbers from 0 to 10 except 3, 7 and 10.
- Write a program to generate Fibonacci series between 0 and 50.
- Write a program which iterates the integers from 1 to 50. For multiple of three print “Fizz” instead of the numbers and fro the multiples of five print “Buzz” . For numbers which are multiples of both three and five print “FizzBuzz”.
- Write a program that accepts a string and calculate the number of digits and letters.
- Write a program to check the validity of password input by users.
- Write a program to find the numbers between 100 and 400 where each digit of a number is an even number . The numbers obtained should be printed in a comma-separated sequence.
- Write a program to print alphabet patterns ‘A’ ,D, ‘E’, ‘G’,’L’, ‘T’ and ‘S’ with * symbol.
- Write a python program to create the multiplication table (from 1 to 20) of a number.
- Write a program to print the following patterns :
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
1
22
333
4444
55555
666666
7777777
*
**
***
****
*****
1
12
123
1234
12345
A
BB
CCC
DDDD
EEEEE
********
*******
******
*****
****
***
**
*
12345
1234
123
12
1
*
***
*****
*******
*********
1
2 3 2
3 4 5 4 3
4 5 6 7 6 5 4
5 6 7 8 9 8 7 6 5
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
1
2 3
4 5 6
7 8 9 10
######################################################################