Boolean Values:
A Boolean value is either true or false. It is named after the British mathematician, George Boole, who first formulated Boolean algebra. In Python the two Boolean Values are True and False and the Python type is bool. Enter the following into the Python shell and observe the output.
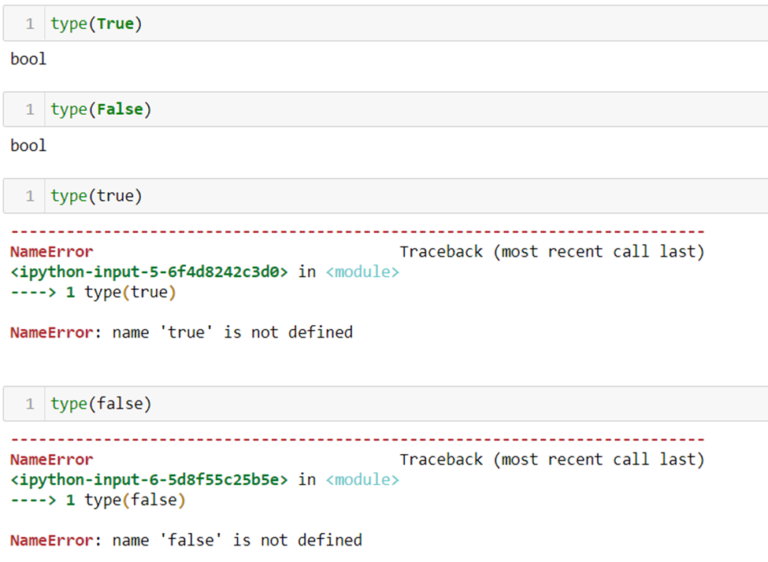
In Python, boolean values are used to represent logical values of True
or False
. These values are often used in conditional statements, loops, and other control structures to control the flow of the program. Here are some examples of using boolean values in Python:
- Boolean values in conditional statements:
age = 18
if age >= 18:
print("You are an adult")
else:
print("You are not an adult")
In the example above, the age
variable is checked if it is greater than or equal to 18, and if it is, the program prints “You are an adult”, otherwise, it prints “You are not an adult”.
2. Boolean values in loops:
fruits = ["apple", "banana", "cherry", "orange"]
for fruit in fruits:
if fruit == "banana":
print("I found a banana!")
break
else:
print("No banana found :(")
In the example above, the program loops through a list of fruits and checks if each fruit is a banana. If it finds a banana, it prints “I found a banana!” and exits the loop using the break
statement.
- Boolean values in functions:
def is_even(number):
return number % 2 == 0
print(is_even(4)) # Output: True
print(is_even(3)) # Output: False
In the example above, the is_even()
function checks if a given number is even or not by checking if the remainder of the number divided by 2 is equal to 0. It returns True
if the number is even, and False
otherwise. Boolean values are a fundamental part of Python programming and are used in a wide range of applications.
Boolean Expressions
A Boolean expression is an expression that evaluated to produce a result which is a Boolean value. For example, the operator == tests if two values are equal. It produces (or yields) a Boolean value:
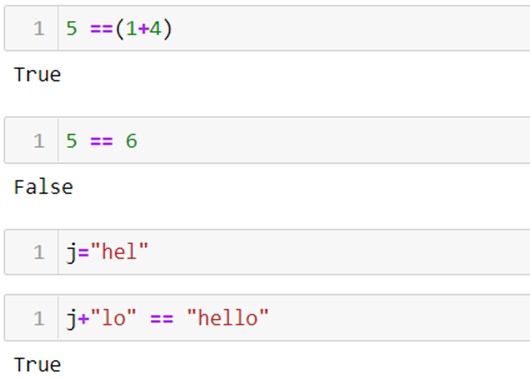
In the first statement the two operands evaluate to equal values, so the expression evaluates to True; in the second statement, 5 is not equal to 6 we get False.
Boolean Operators
In Python, boolean operators and expressions are used to combine boolean values and perform logical operations on them. There are three boolean operators in Python: and
, or
, and not
. Here are some examples of using boolean operators and expressions in Python:
and
operator: Theand
operator returnsTrue
if both operands areTrue
, otherwise, it returnsFalse
.
x = 5
y = 10
if x > 0 and y > 0:
print("Both x and y are positive")
else:
print("At least one of x and y is not positive")
In the example above, the and
operator is used to check if both x
and y
are positive. Since both variables are greater than 0, the program prints “Both x and y are positive”.
or
operator:
The or
operator returns True
if at least one of the operands is True
, otherwise, it returns False
.
x = 5
y = -10
if x > 0 or y > 0:
print("At least one of x and y is positive")
else:
print("Neither x nor y is positive")
In the example above, the or
operator is used to check if at least one of x
and y
is positive. Since x
is positive, the program prints “At least one of x and y is positive”.
not
operator:
The not
operator returns the opposite of a boolean value. If the value is True
, it returns False
, and vice versa.
x = 5
if not x > 0:
print("x is not positive")
else:
print("x is positive")
In the example above, the not
operator is used to check if x
is not positive. Since x
is positive, the program prints “x is positive”.
Boolean expressions can also be used to create more complex boolean logic by combining multiple boolean values and operators. Here’s an example:
x = 5
y = 10
if (x > 0 and y > 0) or (x < 0 and y < 0):
print("Both x and y have the same sign")
else:
print("x and y have opposite signs")
In the example above, the program checks if x
and y
have the same sign by using the and
and or
operators to combine boolean expressions.