Syllabus :
Python Basics: Introduction, Entering Expressions into the Interactive Shell, The Integer, Floating-Point, and String Data Types, String Concatenation and Replication, Storing Values in Variables, Your First Program, Dissecting Your Program.
1.1 Introduction
Python is a general-purpose interpreted, interactive, object-oriented and high-level programming language. It was created by Guido van Rossum during 1985 – 1990. Python is named after a TV Show called ‘Monty Python’s Flying Circus’ and not after Python-the snake. Some of the Features that Makes Python more popular are:
- Python is Simple and Easy to learn and code.
- Python is Free and Open Source. It is freely available at the https://www.python.org/ . Python source code is also available to the public, one can download it, use it, and share it.
- Python is High Level Language and supports both Procedure oriented and Object-Oriented Language concepts along with dynamic memory management.
- Python is portable. Python code can be run on any platforms like Linux, Unix, Mac and Windows.
- Python is extensible and integrated. Python code can be extended and integrated with among other languages like C, C++, Java, etc.
- Python is an interpreted language. Python code is executed line by line at a time and there is no need to compile, which makes debugging easier.
- Python has rich set of libraries for data analytics, machine learning, artificial intelligence, deep learning, mathematical computation, web app development, mobile app development, testing, etc.
- Python is a dynamically typed language. Here the data type for variable is decided at run time. As a result, there is no need to specify the type of variable.
1.2 Why One Should Learn Python Program?
Python Programming is a fun, creative and rewarding activity. Python is one of the most widely used programming language across the world for developing software applications. It is named as one of top picked programming languages of most of the universities and industries. There are several reasons why learning Python programming can be beneficial, including:
- Versatility: Python is a versatile language that can be used for a variety of tasks, such as web development, data analysis, scientific computing, machine learning, and artificial intelligence. It is used by companies such as Google, Instagram, Spotify, and Dropbox.
- Easy to learn: Python has a simple syntax that is easy to read and write, making it a great language for beginners. It also has a large standard library with pre-built modules and functions that can be used to perform various tasks, reducing the amount of code that needs to be written.
- Large community: Python has a large community of developers who contribute to open-source projects, create libraries and frameworks, and offer support and resources for learning. This community provides a wealth of knowledge and resources that can help beginners get started with programming and advanced developers solve complex problems.
- High demand: Python is one of the most popular programming languages in the world, and there is a high demand for developers who know how to use it. Learning Python can open up many job opportunities in various industries, such as tech, finance, healthcare, and more.
- Future-proof: Python has been around for over 30 years and continues to evolve and improve. It is expected to remain relevant in the future and is likely to continue to be used for a wide range of applications.
Overall, learning Python programming can be a valuable skill that can lead to many opportunities and benefits in both personal and professional life.
Reference Video : Top 10 Reasons To Learn Python
Steps to Learn Python Programming Language :
Here are some general steps you can follow to learn the Python programming language:
- Learn the basics: Start by learning the fundamental concepts of Python programming, including data types, variables, operators, control structures, functions, and modules. There are many online resources and books available to help you learn the basics of Python.
- Practice writing code: Once you have a basic understanding of the concepts, start practicing writing code in Python. Start with simple programs, such as a program that prints “Hello, World!” to the console. As you become more comfortable with the language, try writing more complex programs.
- Learn from examples: There are many online resources where you can find examples of Python code, including tutorials, blog posts, and open-source projects. Look for examples that solve problems similar to what you want to achieve and try to understand how they work.
- Join a community: Python has a large and active community of programmers who are eager to help others learn. Join an online programming community, attend coding meetups or workshops, or find a mentor who can help you improve your coding skills.
- Practice regularly: Like any skill, learning to program takes practice. Set aside regular time to practice writing code and experimenting with different techniques. The more you practice, the better you will become.
- Build projects: Once you have a good grasp of the fundamentals of Python programming, start building projects. Choose projects that interest you, such as building a web application, analyzing data, or automating tasks. Building projects will help you apply what you’ve learned and gain practical experience.
Learning program takes time and effort. Be patient with yourself, don’t be afraid to make mistakes, and keep practicing. With persistence and dedication, you can become a skilled Python programmer.
General Steps to teach Python Programming Language
Here are some general steps you can follow to teach Python programming language:
- Introduce the basics: Start by introducing the fundamental concepts of Python programming, including data types, variables, operators, control structures, functions, and modules. Provide examples and exercises to help students understand the concepts.
- Use interactive learning tools: Use interactive learning tools, such as coding challenges, quizzes, and games, to help students practice writing Python code and reinforce their learning.
- Provide real-world examples: Use examples that relate to real-world problems or scenarios to help students understand how Python can be used in practical applications. This can help keep students engaged and motivated.
- Encourage collaboration: Encourage students to work together on programming projects, as this can help them learn from each other and develop problem-solving skills.
- Provide feedback: Provide feedback on students’ code and offer suggestions for improvement. This can help students learn from their mistakes and improve their coding skills.
- Supplement with online resources: There are many online resources available to help students learn Python programming, including tutorials, videos, and interactive coding environments. Use these resources to supplement your own teaching and provide students with additional opportunities to practice and learn.
- Assign projects: Assign projects that challenge students to apply what they’ve learned in creative and practical ways. This can help students build confidence and demonstrate their understanding of the material.
- Assess student progress: Regularly assess student progress to ensure they are understanding the material and making progress. Use quizzes, tests, or project-based assessments to evaluate student understanding and provide feedback.
Teaching Python programming requires patience, persistence, and dedication. Be prepared to adjust your teaching methods based on the needs and progress of your students, and always be willing to learn and improve your own teaching skills.
Applications Of Python :
Python is a versatile programming language that can be used in many different applications. Here are some of the most common uses of Python:
- Web development: Python is used to develop web applications, such as Flask and Django. It is a popular choice for web development because of its simplicity and versatility.
- Data analysis: Python has many libraries for data analysis, such as NumPy, Pandas, and Matplotlib. These libraries can be used to analyze and visualize data.
- Machine learning: Python is used for machine learning and artificial intelligence applications, such as TensorFlow and Keras. These libraries can be used to build and train machine learning models.
- Automation: Python is used to automate tasks, such as web scraping and file handling. It can also be used for system administration tasks, such as server management and network automation.
- Game development: Python is used to develop games, such as Pygame and PyOpenGL. These libraries can be used to create 2D and 3D games.
- Desktop applications: Python is used to create desktop applications, such as music players and text editors. The PyQt and Tkinter libraries can be used to create graphical user interfaces (GUIs).
- Scientific computing: Python is used in scientific computing applications, such as computational biology and physics simulations. The SciPy and NumPy libraries can be used for numerical computation and scientific data analysis.
Overall, Python is a versatile language that can be used in many different applications. Its ease of use, large library of modules and functions, and community support make it an excellent choice for many different types of projects.
1.3 Zen of Python
The Zen of Python is a collection of guiding principles for writing computer programs in the Python language. It was written by Tim Peters, a long-time contributor to the Python community. The Zen of Python can be accessed by typing “import this” in a Python interpreter or code editor.The Zen of Python is made up of 19 aphorisms that encourage simplicity, readability, and practicality in programming. Simple Python code that displays the Zen of Python is as follows:
Source Code :
import this
print this
Output :
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than right now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
Building Blocks :
In the context of programming languages, building blocks refer to the fundamental concepts, components, or elements that form the foundation of the language. These building blocks are used to create more complex programs and applications.
In programming, building blocks are similar to the building blocks used in construction. Just as a construction worker uses bricks, concrete, and steel to build a building, a programmer uses building blocks like variables, data types, operators, and control structures to build software applications.
The concept of building blocks is important because it allows programmers to break down complex problems into smaller, more manageable pieces. By understanding the building blocks of a programming language, programmers can use them to create solutions to specific problems and build more complex software applications.
In summary, building blocks are the basic components of a programming language that are used to create programs and applications. Understanding these building blocks is essential for learning and writing programs in any programming language.
Building Blocks of Python
The building blocks of Python include:
- Variables: Variables are used to store values in Python. They can hold different types of data such as numbers, strings, or lists.
- Data Types: Data types determine the type of data that can be stored in a variable. Common data types in Python include integers, floating-point numbers, strings, lists, and dictionaries.
- Operators: Operators are used to perform mathematical or logical operations on data. Examples include arithmetic operators like +, -, *, and /, and comparison operators like ==, >, and <.
- Control Structures: Control structures are used to control the flow of execution in a program. Examples include if-else statements, while loops, and for loops.
- Functions: Functions are reusable blocks of code that perform a specific task. They can take input arguments and return output values.
- Modules: Modules are pre-written Python code that can be imported into a program to provide additional functionality.
- Comments: Comments are used to add notes to Python code that explain what the code is doing. They are ignored by the Python interpreter.
- Indentation: Python uses indentation to indicate blocks of code. This means that the indentation level of each line of code is important and must be consistent.
Understanding these building blocks is essential for learning and writing Python programs.
1.4 Installing Python:
Before starting the programming in Python, one should install Python interpreter on the computer. To install python one must download the installation package of the required version from the link/ULR : https://www.python.org/. For windows there is a Windows x86-64 executable installer in both 32 bit and 64 bit versions. Latest python release as on 5th September 2020 is Python 3.8.5 .
Here are the steps to install Python on your computer:
- Go to the official Python website at https://www.python.org/.
- Click on the “Downloads” tab at the top of the page.
- Scroll down and choose the latest version of Python that is compatible with your operating system. For example, if you’re using Windows, you may want to download the latest version of Python 3 for Windows.
- Click on the installer file to begin the installation process.
- Follow the prompts in the installer to complete the installation process. You may be asked to choose a destination folder for Python and select optional features to install.
- Once the installation is complete, you should be able to access Python from the command line or through a Python IDE (Integrated Development Environment) like PyCharm or IDLE.
Illustration :
- Launch a web browser then navigate to downloads for Windows. Download the Python 3.8.5.
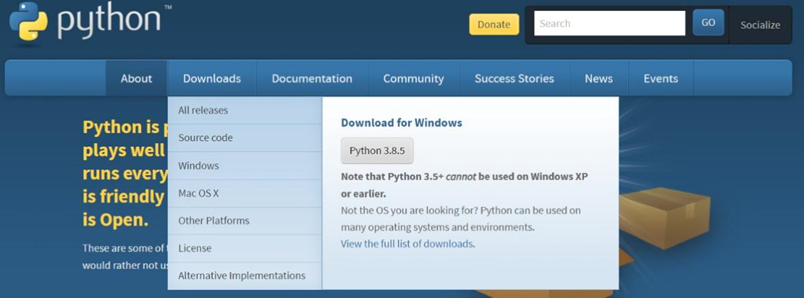
2. When the download completes run the installer
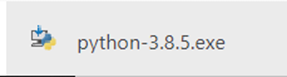
3. Choose to install Now
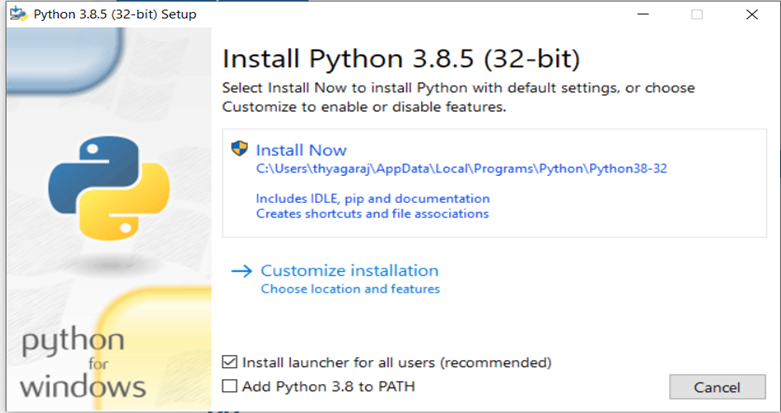
4.After the setup is completely installed you will get Setup was successful message.
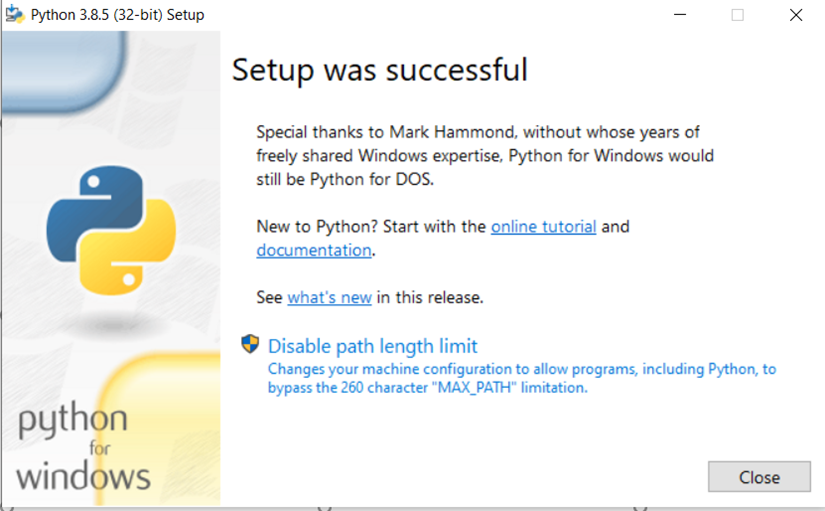
Reference Video : Introduction to Python IDLE | IDLE Installation and Configuration Tutorial | Edureka
Reference Video Link : How to install Python 3.10.0 on Windows 10
1.5 Interacting with Python IDLE (Integrated Development Learning Environment)
To write and run Python code interactively one can either use the command line window (Shell) or the IDLE. IDLE is Integrated Development Learning Environment that comes with Python, that can be used to edit, run, browse, and debug a Python program from a single interface.
1..5.1 Python Shell and Python Editor: Python IDLE can started by clicking its icon created on the desktop or menu item on the START menu -> Python3.8 -> IDLE (Python3.8 32- bit) option as illustrated below:
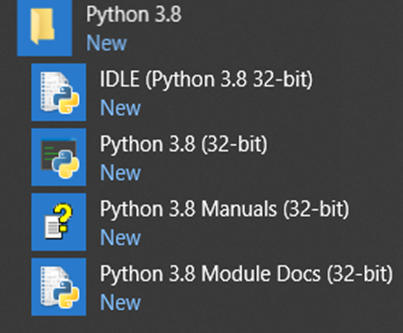
Python IDLE comprises Python shell (Interactive mode) and Python Editor (Script mode).
Python Shell:
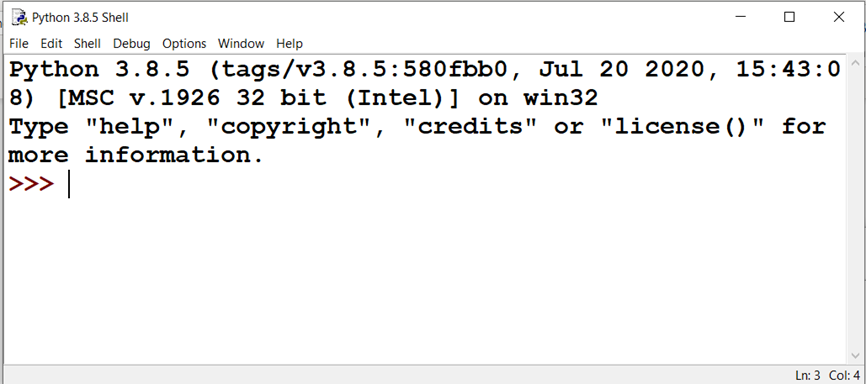
Python Editor:
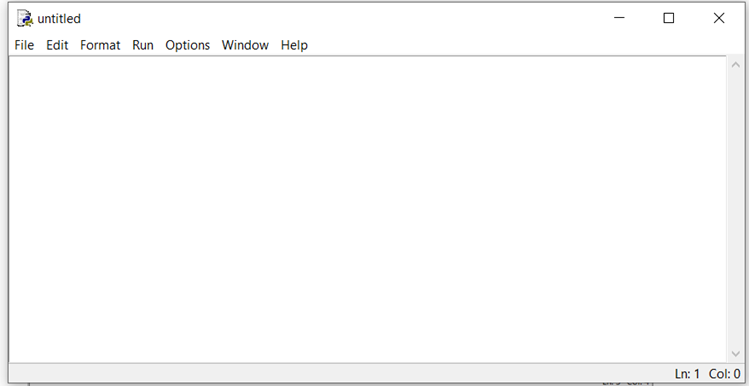
Python IDLE always starts by default with shell. Python shell shows a header message displaying its version and copyright notice. Soon after the header message, the command prompt (>>>) followed by blinking cursor gets displayed, indicating its readiness for accepting user Python commands/instructions /code. The three greater than symbols are called the prompt or Python Command prompt. Python Shell is an interactive window where we can type in the Python code and see the output in the same window as illustrated below:
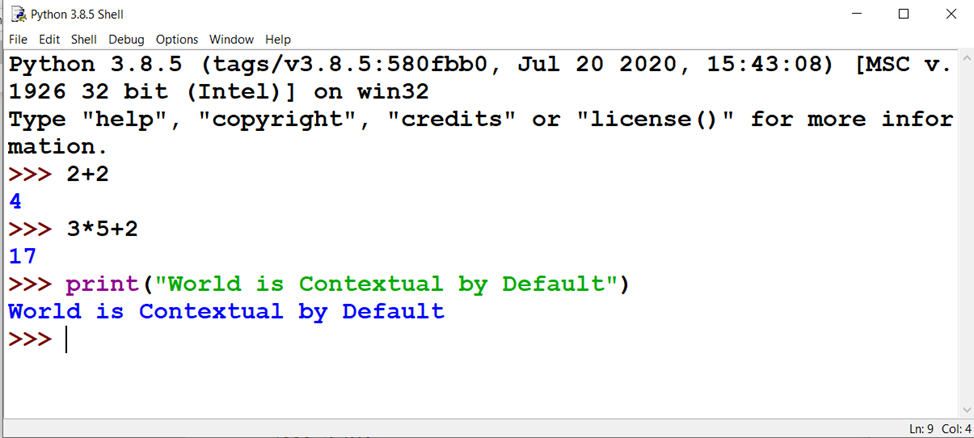
1.5.2 Entering Expressions into the interactive Shell
In Python, expression is the most basic kind of programming instruction in the language. An expression is a combination of values, variables, and operators.
Examples: 17, x, x+17 ,1+2*2 , X**2, x**2 +y**2
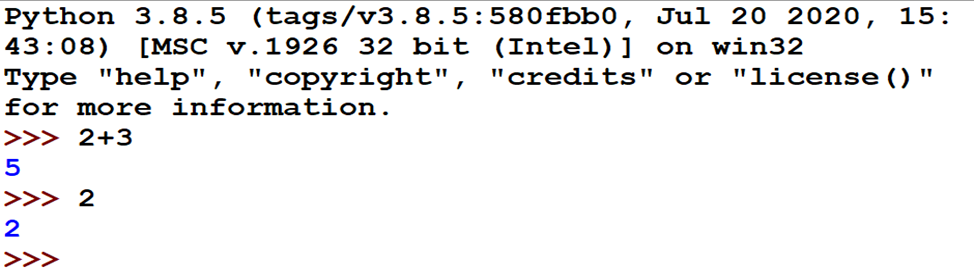
1.6 Value:
A value is a letter or a number assigned to a variable or constant.
Example: 1, 2 , 33.45 ,”USN” and “Hello, World!”.
Value may be of different types. Types are the data types to which the Values belong. Type may int, float, string, complex number, dictionary, list, sets, boolean values , binary numbers ,etc.
type(arg) function returns the data type of the argument as illustrated below :
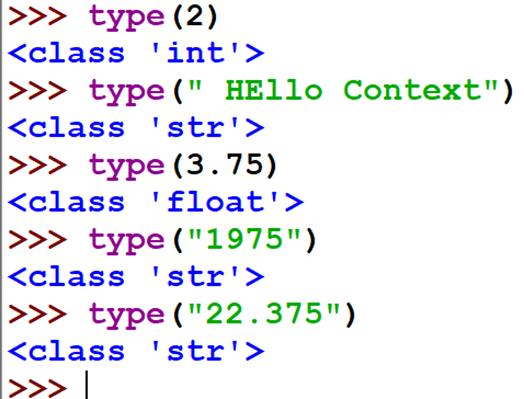
1.7 Data types:
Python supports various data types. Here are some of the most commonly used data types in Python along with examples:
- Numeric Types:
- int: integers with no fractional part, e.g., 42, -5, 0
- float: floating-point numbers with a fractional part, e.g., 3.14, -0.5, 2.0
- Complex (complex): complex number with a real and imaginary part z = 2 + 3j,z2 = 1 – 2j,z3 = 4 + 0j
- Boolean Type:
- bool: represents two values True or False
- Sequence Types:
- str: a sequence of Unicode characters, e.g., “Hello”, ‘world’, “I’m a string”
- list: an ordered sequence of items, e.g., [1, 2, 3], [‘apple’, ‘banana’, ‘cherry’]
- tuple: an ordered, immutable sequence of items, e.g., (1, 2, 3), (‘apple’, ‘banana’, ‘cherry’)
- Set Types:
- set: an unordered collection of unique items, e.g., {1, 2, 3}, {‘apple’, ‘banana’, ‘cherry’}
- Frozen Set (frozenset): fruits = frozenset([“apple”, “banana”, “cherry”])
- Mapping Type:
- dict: a collection of key-value pairs, e.g., {‘name’: ‘John’, ‘age’: 25}
- Other Types:
- bytes: a sequence of bytes, e.g., b’hello’
- bytearray: a mutable sequence of bytes, e.g., bytearray(b’hello’)
- Memoryview (memoryview)
- NoneType: represents the absence of a value, e.g., None
These are the built-in data types in Python. There are also many other data types that are available through Python libraries or can be created by the programmer.
1.8 Variables
In Python, a variable is a named reference to a value that is stored in memory. A variable is created when a value is assigned to it using the assignment operator =
.
Examples :
Message = ‘Python Programming ‘,
p =1000, t= 2, r=3.142,
Si = p*t*r/100,
pi = 3.1415926535897931,
area_of _circle = pi*r*r.
Here are some rules for naming Python variables:
- Variable names should begin with a letter (a-z or A-Z) or an underscore (_). They cannot begin with a number.
- Variable names can only contain letters, numbers, and underscores. Special characters such as !, @, #, etc. are not allowed.
- Variable names are case-sensitive, meaning “myVar” and “myvar” are considered as two different variables.
- Variable names should be descriptive, and they should reflect the purpose of the variable. For example, if a variable stores a person’s age, you could name it “age.”
- Avoid using reserved keywords as variable names. Reserved keywords are words that have a special meaning in Python, such as “if,” “else,” “while,” etc.
- Use lowercase letters for variable names, unless you have a good reason to use uppercase letters.
- If a variable name contains multiple words, use underscores to separate them. For example, if a variable stores a person’s full name, you could name it “full_name.”
Following above rules can make your code more readable and understandable for other developers.
Table: Valid Variable Names and Invalid Variable Names
Valid Variable Names | Invalid Variable Names |
python12 | current- account (hyphens are not allowed) |
Simple | savings account (spaces are not allowed) |
interest_year | 4freinds (can’t begin with a number) |
_rate_of_interest | 19/08/2004 (can’t begin with a number special characters like / are not allowed) |
_spam | 10April_$ (cannot begin with a number and special characters like $ are not allowed) |
HAM | Principle#@ ( special characters like # and @ are not allowed) |
account1234 | ‘bear’ ( special characters like ‘ is not allowed) |
Note:
Variable names are case-sensitive, meaning that velocity, VELOCITY, Velocity and VeloCity are four different variables. It is a Python convention to start your variables with a lowercase letter.
1.10 Storing Values in a Variables :
Values can be stored in a variable using Assignment statement. An assignment statement consists of a variable name , an equal sign and the value to be stored .
Example :
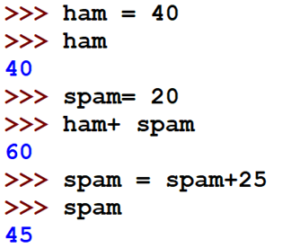
A variable ham is initialized (or created ) the first time a value is stored in it. After that one can use it in expression with other variables and values like ham + spam. When a variable is assigned a new value, old value gets erased or forgotten. This is called overwriting. Following code illustrates the overwriting of string
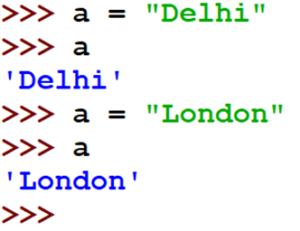
1.11 Sample Program:
To read and print your name and age
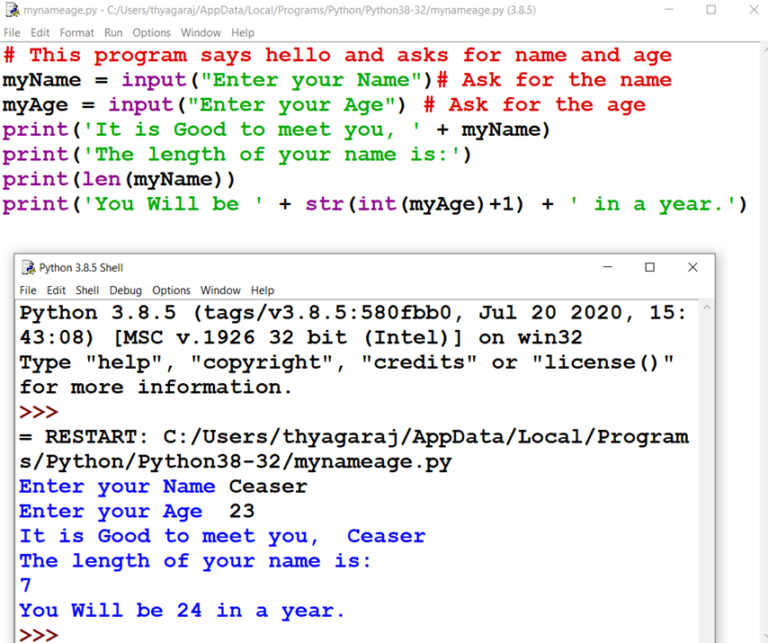
1.12 Dissecting the Sample Program
Sample program comprises executable statements containing comments and built in functions like print () , input () , len() , int() and str()
Comments:
Comments are readable explanation or descriptions that help programmers better understand the intent and functionality of the source code. Comments are completely ignored by interpreter.
Advantages of Using Comments:
- Makes code more readable and understandable.
- Helps to remember why certain blocks of code were written.
- Can also be used to ignore some code while testing other blocks of code.
Single Line Comments in Python:
The hash symbol #is used to write a single line comment.
Example:
# Printing a message
print(“ Enter your Name “)
myName = input (“ Enter Your Name”) # Read your name to myName
Multiline Comments in Python:
1. Using # at the beginning of each line of comment on multiple lines
Example:
# It is a
# multiline
# comment
2. Using String Literals ”’ at the beginning and end of multiple lines
Example:
”’
I am a
Multiline comment!
”’
The print() Function :
The print function is used to display the string value written within pair of double quotes inside the parentheses on the screen .
print(‘It is Good to meet you, ‘ + myName)
print(‘The length of your name is:’)
print(len(myName))
print(‘You Will be ‘ + str(int(myAge)+1) + ‘ in a year.’)
The line print(‘The length of your name is:’) means “Print out the text in the string ‘’The length of your name is:’ . When Python executes the print statement, python interpreter calls the print()function and the string value is being passed to the function. The value within print() is called argument . Quotes within parentheses marks where the string begins and ends ; they are not part of the string value.
The input() function
This function is used to take the input from the user . Whatever the user enter as input, input() function convert it into a string . if you enter an integer value still input() function convert it into a string . The programmer is needed to convert it into an integer in your code using typecasting.
Myname = input(“Enter your name”)
Example:
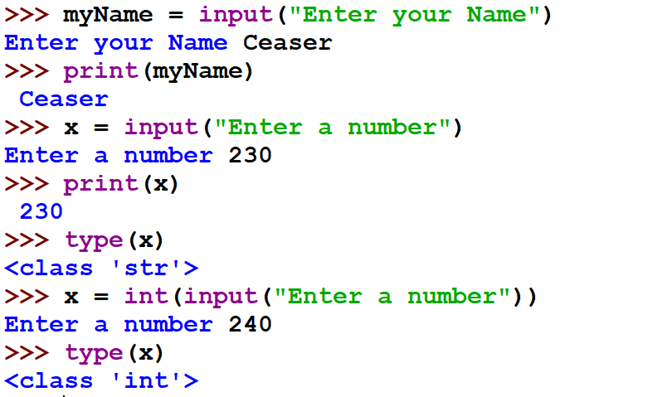
Reading multiple values using input()
Programmer often want as user to enter multiple values in one line . In Python user can take multiple values or inputs in one line by using split() method . It breaks the given input by the specified separator. If separator is not provided then any white space is a separator. Generally, user use a split() method to split a Python string but one can used it in taking multiple input.
Example:
>>> x, y,z = input (“Enter three values”).split()
Enter three values 2 3 4
>>> x
‘2’
>>> y
‘3’
>>> z
‘4’
In order to get the input from the user through the keyboard Python provides a built-in function called input. When this function is called, the program stops and waits for the user to type something. When the user presses Return or Enter, the program resumes and input returns what the user typed as a string.
Example 1: Program to find the simple interest
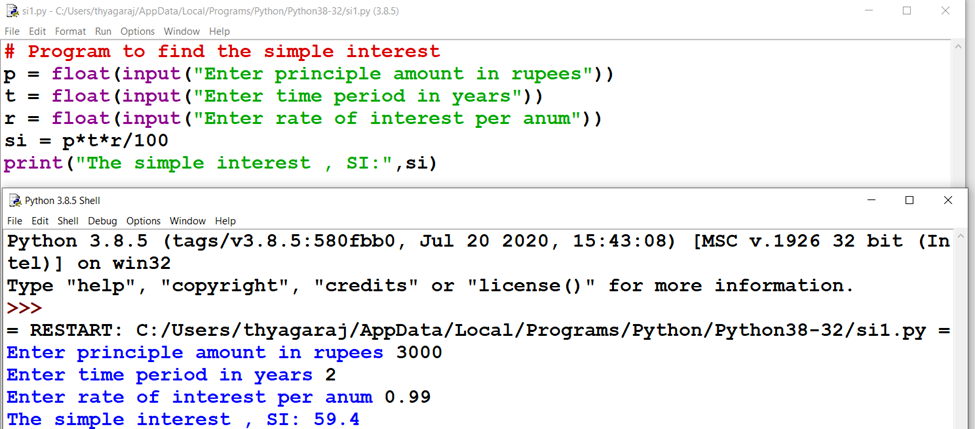
Example 2 : Python Program to Add Two Numbers
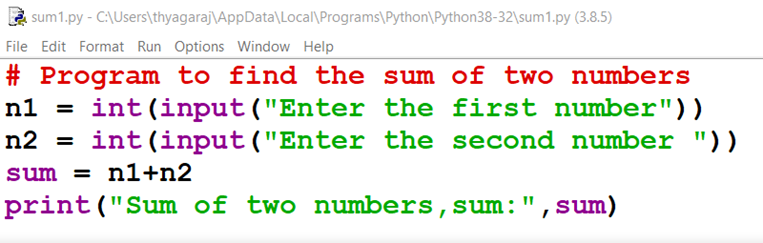
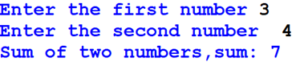
The len() Function
In python len() function is used to find the number of characters in a given string as illustrated below :

The str() , int() and float Functions :
str() function can be used convert integer or floating numbers into string data type.
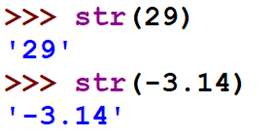
Similarly int() and float() function will evaluate into integer and floating – point forms of the value you pass , respectively.
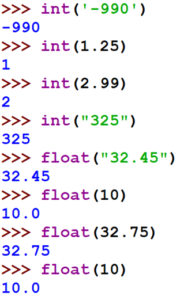
Note that if a values is passed to int() or float that they cannot evaluate as integer or float , Python will display an error message.
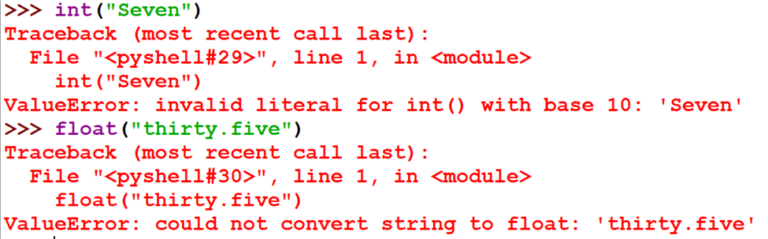
The int() function can be used to round a floating point number down as illustrated below :
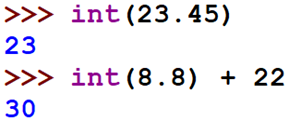
1.13 Operators and operands
- Operators are special symbols that represent computations like addition and multiplication. The values the operator is applied to are called operands.
- The operators +, -, *, /, and ** perform addition, subtraction, multiplication, division, and exponentiation, as in the following examples:
Operator | Operation | Example | Evaluates to |
** | Exponent | 5**3 | 125 |
% | Modulus/Remainder | 33%7 | 5 |
// | Integer Division/Floored quotient | 33//5 | 6 |
/ | Division | 23/7 | 3.2857142857142856 |
* | Multiplication | 7*8 | 56 |
– | Subtraction | 8 – 5 | 3 |
+ | Addition | 7+ 3 | 10 |
Order of operations
- When more than one operator appears in an expression, the order of evaluation depends on the rules of precedence.
PEMDAS order of operation is followed in Python:
- Parentheses have the highest precedence and can be used to force an expression to evaluate in the order you want.
- Exponentiation has the next highest precedence,
- Multiplication and Division have the same precedence, which is higher than
- Addition and Subtraction, which also have the same precedence.
- Operators with the same precedence are evaluated from left to right.
Following examples illustrates the evaluation of expressions by Python interpreter. In each case the programmer must enter the expression , python interpreter evaluates the expression to a single value .
>>> 5+4*3
17
>>> (4+5)*3
27
>>> 12345678*45678
563925879684
>>> 3**5
243
>>> 22//7
3
>>> 22/7
3.142857142857143
>>> 27%5
2
>>>3 + 3
6
>>> (5-2)*((8+4)/(5-2))
12.0
Python interpreter evaluates parts of the expression as per the PEMDAS rule until it becomes a single value as illustrated below :
(5-2)*((8+4)/(5-2))
3 * ((8+4)/(5-2))
3*(12/(5-2))
3*(12/3)
3*4.0
12.0
Note: If you type invalid expressions, python interpreter will not be able to understand it and will display a Syntax Error message as illustrated below:
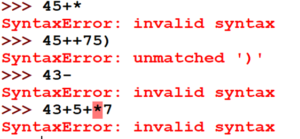
1.14 String Concatenation and Replication
The functionality of the operator may change based on the data types of operand used . For example + which is the addition operator when it operated on two integers or floating – point values , can be used to concatenate two strings as illustrated below :
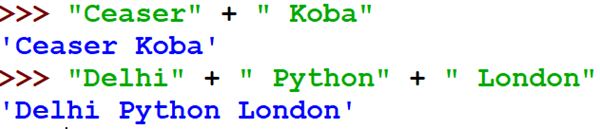
Similarly * operator which is multiplication operator can be used as string replication operator when string is multiplied with a number as illustrated below :

1.15 Python Character Set:
The set of valid characters recognized by Python like letter, digit or any other symbol . The latest version of Python recognizes Unicode character set. Python supports the following character set:
- Letters : A-Z ,a-z
- Digits :0-9
- Special Symbols : space +-/*\**()[]{}//=!= == <> ,”””,;:%!#?$&^ó=@_
- White Spaces : Blank Space, tabs(->), Carriage return , new line , form feed
- Other Characters : All other 256 ACII and Unicode characters
1.16 Python Tokens:
A token (lexical unit) is the smallest element of Python script that is meaningful to the interpreter . Python has following categories of tokens: Identifiers, Keywords , Literals , operators and delimiters.
Identifiers:
Identifiers are names that you give to a variable , class or Function. There are certain rules for naming identifiers similar to the variable declaration rules , such as : No Special character except_ , Keywords are not used as identifiers , the first character of an identifier should be _ underscore or a character , but a number is not valid for identifiers and identifiers are case sensitive .
Literals:
A fixed numeric or non-numeric value is called a literal . Literals may be string, numbers (int, long, float and complex), Boolean (True or False), NONE and Operators.
Operators :
A Symbol or a word that performs some kind of operation on given values and returns the result. There are 7 types of operators available for Python: Arithmetic Operator ,Assignment Operator, Comparison Operator, Logical Operator , Bitwise Operator , Identity Operator and Membership Operator .
Delimiters:
Delimiters are the symbols which can be used as separators of values or to enclose some values. Examples of delimiters are () {} [],;:
Note : Comments and # symbol used to insert a comment is not a token.
Keywords:
The reserved words of Python which have a special fixed meaning for the interpreter are called keywords. No keyword can be used as an identifier or variable names. There are 35 keywords in python as listed below:
Keyword | Description |
and | Logical and operator |
as | Alias |
assert | Used for debugging |
async | Used to make a function asynchronous by adding the async keyword before the function’s regular definition |
await | Used in asynchronous functions to specify a point in the function where control is given back to the event loop for other functions to run. You can use it by placing the await keyword in front of a call to any async function |
break | To break out of a loop |
class | To define a class |
continue | For skipping the statements and conitinuing the next iteration |
def | For defining user defined functions |
del | To delete an object |
elif | Conditional statement, same as else if |
else | Conditional statement |
except | Used in exception handling |
False | Boolean Value |
finally | Used in exception handling , to execute a block of code no matter whether exception is there or not |
for | Used to create for loop – iterative statement |
from | Used to import specific parts of a module |
global | Used to declare global variable |
if | Conditional /decision making statement |
import | Used to import a module or library |
in | Used to check if a value if present in list, tuple, dictionaries , sets ,etc. |
is | To check if two variables are equal |
lambda | Used for defining an anonymous function |
None | Used to represent a null value |
nonlocal | To declare a non-local variable |
not | A logical operator |
or | A logical operator |
pass | A null statement , a statement that will do nothing |
raise | To raise an exception |
return | To exit a function and return a value |
True | Boolean Value |
try | Used in exception handling |
while | For creating a while iterative loop |
with | Used to simplify exception handling |
yield | To end a function , returns a generator |
Following code segment can be used to obtain the list of python keywords :
import keyword
len(keyword.kwlist)
#ouput:
36
print(keyword.kwlist)
#output:
['False', 'None', 'True', '__peg_parser__', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
1.17 Installing Jupyter Notebook
[ source : https://test-jupyter.readthedocs.io/en/latest/install.html ]
Prerequisite: Jupyter requires Python ( Python 3.3 or greater or Python2.7) for installing the Jupyter Notebook. Anaconda distribution is recommended for installing Python and Jupyter .
Installing Jupyter using Anaconda and conda:
To install anaconda, one must download the installation package of the required version from the link/ULR: https://www.anaconda.com/products/individual .
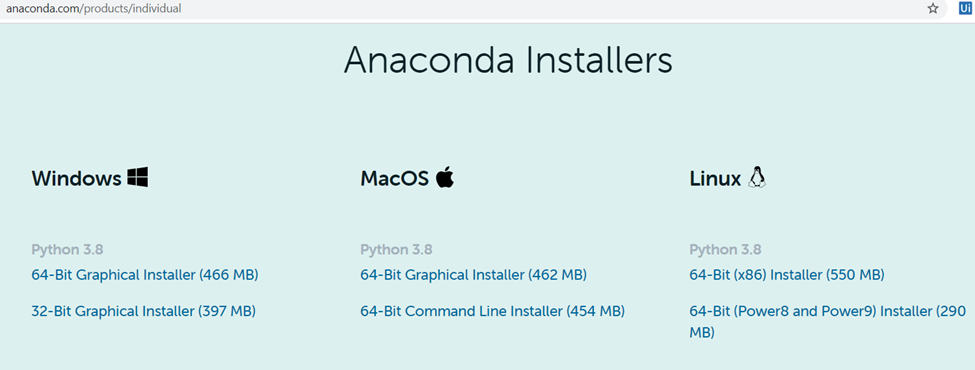
For Windows and for Python 3.8 there is 64 – Bit and 32-Bit Graphical Installer. Once the appropriate Anaconda installer is downloaded and installed successfully , one can find the Jupyter appearing in the Anaconda folder as illustrated below :
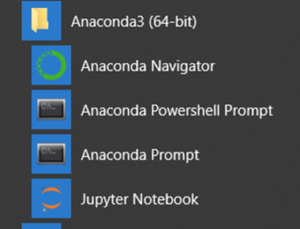
Click the Jupyter Note Book , Server and all Kernels at background will open as illustrated below :
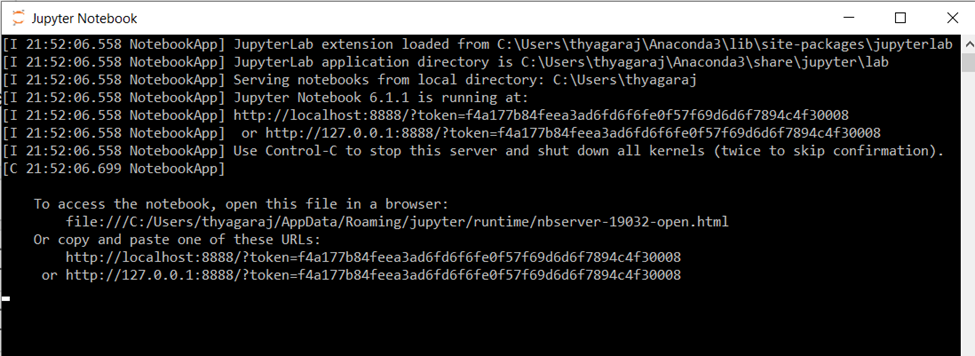
Soon after the Server is launched, Jupyter notebook will get open. Also, one can open the Jupyter notebook by copy and pasting any one of the following URLs as displayed in the message box:
http://localhost:8888/?token=f4a177b84feea3ad6fd6f6fe0f57f69d6d6f7894c4f30008
or http://127.0.0.1:8888/?token=f4a177b84feea3ad6fd6f6fe0f57f69d6d6f7894c4f30008
Jupyter Notebook editor will open as illustrated below:
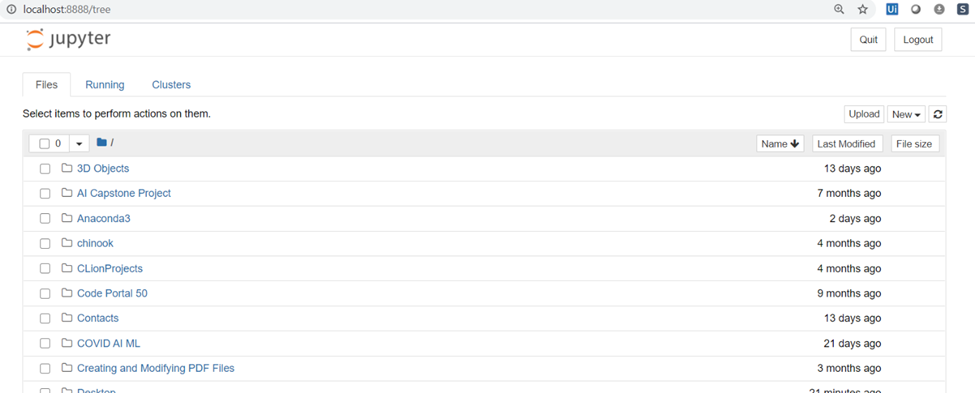
In order to open a new python file, one should click new button and select Python3 , as below :
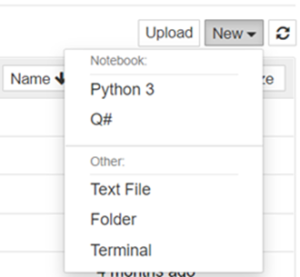
A new untitled Python file with one cell will get open:

Type the python code and execute by clicking Run button :
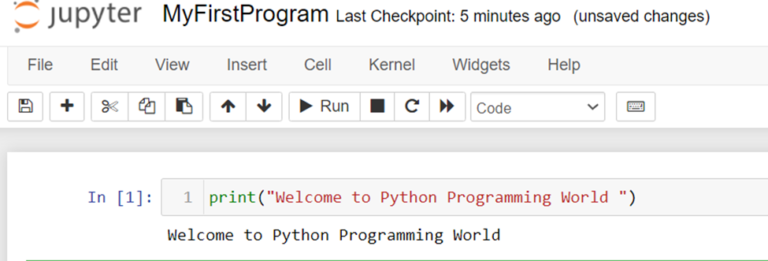
Reference Video Link : How to Install Anaconda and Jupyter Notebook on Windows 10 /11
1.18 Questions for Practice:
- What is Python ? Who created Python?
- What are the features of Python which makes it more popular?
- List out the different jobs available for Python programmer.
- What is the role of programmer? Lis two skills required to become good programmer.
- Discuss, Why Should you learn to write Programs?
- What is Zen of Python?
- Discuss, with snapshots how to install latest version of IDLE Python and Jupyter Python.
- Illustrate with examples how to interact with IDLE Python.
- Explain how mathematical expressions can be executed in interactive shell.
- Illustrate with example how write and execute programs in Jupyter Editor.
- Explain the different components of Jupyter Editor.
- Define Program. Differentiate between Compiler and Interpreter. Give Examples.
- What are Python words and sentences? Explain with an example for each.
- Classify the following list of items into variables, values, operators, strings, and keywords: [List of items: *, + , – , **, < ,> , ‘hello’ , ‘ I am ok . How are you’, -88.8, /, 5, and, is , not , while , for, async, x, si, p , time , rate , velocity , speed, acc , % ,&, ! , ||]
- What are expressions? Illustrate the different types of expressions with examples.
- What are data types? Classify the different data types in python with examples.
- What are Python Variables? What rules one should follow to name the variables.
- Give 5 examples for valid and invalid variables.
- Discuss how to store values in a variable.
- Write a sample program and dissect the program with explanation.
- What are comments? What are the advantages of Comments? Explain the different ways of writing comments.
- Give examples for single and multiline comments.
- Explain the working and usage of print() function with examples.
- Explain the working and usage of input() function with examples.
- Give example to read multiple values using input().
- Define operands and operators. Discuss PEMDAS rules with examples.
- What are keywords? How many keywords are there in current version of Python?
- Write a program to display all keywords in the current version of Python.
- What are Python comments? Explain their importance with programming examples.
- Explain print () , input() and split() functions with example.
- Write a program for the following:
- To read and print a single value in a single line
- To read and print multiple values in a single line
- Explain the following different types of errors: Syntax errors, Semantic errors and Logic Errors.
- Explain the following functions with example: len() , str() , int() , float()
- Predict the out put and justify your answer: (i) -11%9 (ii) 7.7//7 (iii) (200 – 70)*10/5 (iv) not “False” (v) 5*|**2
- List the rules to describe a variable in Python. Demonstrate at least three different types of variables uses with an example program.
- Explain the following :
- Skills necessary for a programmer
- Interactive Mode
- Short circuit evaluation of expression
- Modulus operator.
- Mention three types of errors encountered in python program.
- Define the following with example : Values and Types , Variables , Expressions , Keywords , Statements , Operators and Operands, Order of Operations , Modulus Operators , String operations and Comments.
- What three functions can be used to get the integer, floating-point number, or string version of a value?
1.19 Programs for Practice:
Write and execute python programs for the following :
- To prompt a user for their name and then welcomes them.
- To prompt a user for days and rate per day to compute gross salary.
- To read the following input and display :
- Name :
- USN:
- Roll No:
- Mobile No:
- E-Mail Id:6. Percentage of Marks
- To find the simple interest for a given value of P, T and R. Program should take input from the user.
- To find the compound interest.
- To read two integers and find the sum, diff, mult and div.
- To Convert given Celsius to Fahrenheit temperature.
- To print ascii value of a character.
- To display all the keywords.
- To print the following string in a specific format :””Twinkle, twinkle, little star, How I wonder what you are! Up above the world so high, Like a diamond in the sky. Twinkle, twinkle, little star, How I wonder what you are” .
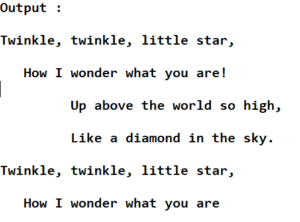
11. To get a python version.
12. To display the current date and time
13. To accept the radius of a circle from the user and compute the area.
14. To print the calendar of a given month and year.
15. To check whether a file exists .
16. To determine if a Python shell is executing in 32 bit or 64 bit mode on OS.
17. To get OS name , platform and release information .
18. To locate Python site packages.
19. To call an external command in python.
20. To get path and name of the file that is currently executing.
21. To parse a string to float or integer.
22. To list all files in a directory in Python.
23. To print without newline or space.
24. To determine profiling of Python programs.
25. To print to stderr.
26. To access environment variables.
27. To get the current username.
28. To find the local IP addresses using Pythons stdlib.
29. To get execution time for a python method.
30. To convert height in meters to centimeters.
31. To Convert all units of time to seconds
32. To convert the distance in feet to inches , yards and miles.
33. To calculate body mass index.
34. Given variables x = 15 and y = 30 , write a Python program to print “15+30=45”.
35. To get the identity of the object
36. To check whether a string is numeric.
37. To get the system time .
38.To clear the screen or terminal
39.To calculate the time runs (difference between start and current time ) of a program.
40.To input integer if not generate error.
********************************************************************************************
Questions and Answers :
1. What is Python ? Who created Python?
Python is a high-level, interpreted programming language that was first released in 1991 by Guido van Rossum. It is designed to be easy to read and write, with a simple and elegant syntax that emphasizes code readability.
Python is a dynamically typed language, meaning that variable types are determined at runtime, and it supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
Python is widely used in various industries, including web development, data science, artificial intelligence, scientific computing, and more. It has a vast and active community of developers who contribute to its development and create numerous libraries and frameworks to extend its capabilities.
Guido van Rossum started the development of Python in the late 1980s while working at the National Research Institute for Mathematics and Computer Science in the Netherlands. He wanted to create a language that was easy to learn and use, with a focus on code readability and simplicity.
Today, Python is one of the most popular programming languages in the world, with a large and growing user base. Its popularity is due to its ease of use, versatility, and wide range of applications.
2. What are the features of Python which makes it more popular?
Python has gained widespread popularity due to its numerous features that make it a powerful and versatile programming language. Some of the features that contribute to Python's popularity are:
Easy to learn and use: Python has a simple and intuitive syntax that is easy to read and write, making it an ideal language for beginners.
Large and active community: Python has a vast and active community of developers who contribute to its development and create numerous libraries and frameworks to extend its capabilities.
Cross-platform compatibility: Python is a cross-platform language, meaning that code written in Python can run on multiple operating systems without any modifications.
Dynamically typed: Python is dynamically typed, meaning that variable types are determined at runtime, making it more flexible and easier to use.
High-level language: Python is a high-level language, meaning that it abstracts away low-level details of the machine, making it easier to write code quickly.
Object-oriented: Python supports object-oriented programming, allowing developers to create reusable and modular code.
Extensive standard library: Python comes with a vast standard library that provides a wide range of functionalities, such as working with files, network programming, database access, and more.
Interpreted: Python is an interpreted language, meaning that code is executed line by line, making it easier to test and debug code.
Scalable: Python can be used for small and large-scale projects alike, and it is scalable due to its support for modules, packages, and third-party libraries.
Data science and machine learning support: Python has gained popularity in the data science and machine learning community due to its extensive libraries such as NumPy, Pandas, Matplotlib, and more.
Overall, these features make Python a popular and versatile language that is used in a wide range of industries, from web development to scientific computing to artificial intelligence.