Dr.Thyagaraju G S and Palguni G T
C is a high-level, general-purpose programming language that was originally developed at Bell Labs by Dennis Ritchie in the 1970s. It is a high-level language that is used to develop system software, applications, and embedded systems. C is a compiled language, which means that the code is compiled into machine-readable code before it can be executed. It is a structured, imperative, procedural, and compiled language that is widely used for system programming, embedded systems, and other applications that require efficient low-level access to hardware and memory.
C has a simple syntax that is easy to learn and understand. It provides low-level access to memory, which makes it useful for system programming, but it also supports high-level constructs such as functions, structures, and pointers. C is known for its efficiency and speed, and is often used for developing operating systems, device drivers, and other system software.
Key Features of C
Here are some key features and concepts of the C programming language:
- Syntax: C has a simple syntax that is easy to learn and read. It uses semicolons to end statements and curly braces to group statements into blocks.
- Data types: C has several built-in data types, including integers, floating-point numbers, characters, and arrays. It also allows users to define their own data types using structures, unions, and enumerations.
- Pointers: Pointers are a fundamental feature of C. They allow users to manipulate memory directly, and are essential for tasks such as dynamic memory allocation and accessing hardware devices.
- Functions: Functions are a core concept in C, and are used to break up code into modular, reusable components. C also supports the use of function pointers, which allow users to pass functions as arguments to other functions.
- Preprocessor: The C preprocessor is a powerful tool that allows users to perform text substitution and conditional compilation. It is often used for tasks such as including header files, defining macros, and performing platform-specific code changes.
- Standard library: C comes with a large standard library that provides a wide range of functions for tasks such as file I/O, string manipulation, and memory management.
- Portability: C is a portable language that can be compiled on a wide range of platforms, from embedded devices to supercomputers.
C has had a major influence on the development of many other programming languages, including C++, Java, and Python. Its low-level access to hardware and memory make it a powerful tool for system-level programming, while its simplicity and portability make it a popular choice for a wide range of applications.
Limitations
Although C is a powerful and widely used programming language, it does have some limitations:
- Lack of built-in support for object-oriented programming: C is not designed as an object-oriented programming language, and it lacks built-in support for classes, objects, and inheritance.
- Manual memory management: C requires manual memory management, which means that the programmer is responsible for allocating and freeing memory for variables and data structures. This can lead to memory leaks and other memory-related issues if not done carefully.
- Lack of bounds checking: C does not provide built-in bounds checking for arrays and pointers, which can lead to buffer overflows and other memory-related issues if not done carefully.
- Limited standard library: C’s standard library is relatively small and lacks some of the high-level functions and data types found in other programming languages.
- Portability issues: C code written for one platform may not necessarily work on another platform without modification, due to differences in hardware, operating systems, and compilers.
Despite these limitations, C remains a popular and powerful programming language that is widely used for a variety of applications, particularly in systems programming, embedded systems, and operating systems.
Structure of C Program
A C program typically consists of several parts, including:
- Preprocessor directives: These are instructions that are processed before the compilation of the code and provides instructions to the compiler to link functions from the system library. They begin with a hash (#) character and may include instructions to include header files, define macros, and perform other tasks.
- Global declarations: These are declarations of variables and functions that are used throughout the program, but are defined outside of the main function.
- The Definition Section defines all symbolic constants.
- Function declarations: These are prototype declarations of functions and sub functions that are used in the program. Functions are blocks of code that perform a specific task and are called by the program when needed.
- Main function: This is the entry point of the program, and it is where the program starts execution. The main function typically calls other functions and contains the main logic of the program. It contains two parts, declaration and executable part.
- Declaration Part declares all the variables used in the executable part.
- There should be at least one statement in the executable part which contains instructions to perform certain task. The declaration and executable part must appear between the opening and closing braces. All statements in the declaration part should end with the semicolon.
- Variables and data types: These are used to store data within the program. C has several built-in data types, such as integers, floating-point numbers, and characters, as well as the ability to create custom data types using structures and unions.
- Statements and expressions: These are the instructions that tell the program what to do. Statements can include assignment statements, control flow statements (such as if-else statements and loops), and function calls.
- Comments: These are used to document the code and explain what it does. Comments begin with two forward slashes (//) for a single-line comment or with /* and end with */ for multi-line comments.
- The Subprogram Section contains all the user defined functions that are called in the main function. The example given below illustrates the structure of C program.
Preprocessor directives: The preprocessor directives are the statements which start with symbol #. These statements instruct the compiler to include some of the files in the beginning of the program. For example, #include<stdio.h>, #include<math.h> and #include<conio.h> are some of the files that the compiler includes in the beginning of the program. The #include<stdio.h> statement tells the compiler to include the standard input/output library or header file. This file has the function definition for built in functions like printf (), scanf() ,etc. Using the preprocessor directives the user can define the constants also. For example
#define MAX 1024
#define PI 3.1417
Here MAX and PI are called symbolic constants.
Global Declaration and function definitions: The variables that are declared before all the functions are called global variables. The global variables can be accessed by all the functions including main function. As we declare the global variables, the functions are also declared here as illustrated below:
#include<stdio.h>
#include<conio.h>
/* Demonstrating Global variables and function */
add_numbers ( ); /* Function declaration */
int value1,value2, value3; /* Declaration of global variables */
add_numbers( )
{
int result2; /* Declaration of local variable*/
value1=5;
value2=3;
value3=2;
result2 = value1 + value2 + value3;
printf (“ Result 2 = %d \n”,result2);
}
main()
{
int result1;
clrscr ( ) ;
value1 = 10;
value2 = 20;
value3 = 30;
result1 = value1 + value2 + value3;
printf("Result 1 = %d\n”,result1);
add_numbers();
getch();
}
Output :
Result 1 = 60
Result 2 = 10
main program header: In C program the execution always starts from the main( ) function and it can be written as follows :
#include<stdio.h>
#include<conio.h>
main() /* the main statement*/
{ --------
}
The main statement instructs the compiler that execution always starts from function main and it is called main program header. The pair of brackets denoted by ( ) must follow after the main. Every C program must have only one main function.
The Body or Action Portion of the Program:Inside every C main program after the header or top line is a set of braces { and } containing a series of C statements which comprise the body. This is called the action portion of the program.
The body of the program contains two essential parts:
- Declaration Section
- Execution Section
Declaration Section: The variables that are used inside the main function or sub function should be declared in the declaration section. The variables can also be initialized during declaration. For example consider the declarations shown below:
main ()
{ int sum = 0;
int a;
float b;
-----------
}
Here the variable sum is declared as an integer variable and it is also initialized to zero. The variable a is declared as an integer variable whereas the variable b is declared as a floating point variable.
Executable Section: This section includes the instructions given to the computer to perform a specific task. An instruction may represent an expression to be evaluated, input/output statement etc. Each executable statement ends with “;”. The instructions can be input or output statements, simple statements such as if statement, for statement etc.
Example:
main()
{
-------
printf(“\n Enter the values of a and b\n”);
scanf (“%d %d”,&a,&b);
sum =a+b;
printf(“ \n The sum =%d \n”, sum);
}
Example 1 :
#include <stdio.h> // Preprocessor directive
int global_var; // Global declaration
void my_function(); // Function declaration
int main() { // Main function
int local_var; // Local variable declaration
printf("Hello, world!\n"); // Statement
my_function(); // Function call
return 0;
}
void my_function() { // Function definition
printf("This is my function\n");
}
Example 2 :
#include <stdio.h> // Preprocessor directive
int main() { // Main function
int num1 = 10; // Variable declaration and initialization
int num2 = 5;
int sum = num1 + num2; // Statement
printf("The sum of %d and %d is %d\n", num1, num2, sum); // Function call
return 0; // Statement
}
In the above example, the program has the following structure:
- Preprocessor directive:
#include <stdio.h>
includes the standard input/output library in the program.
- Main function:
int main() { ... }
is the main function of the program, which is the entry point of the program.
- Variables and data types:
int num1 = 10;
int num2 = 5;
int sum = num1 + num2;
declares and initializes three integer variablesnum1
,num2
, andsum
.
- Statements and expressions:
printf("The sum of %d and %d is %d\n", num1, num2, sum);
is a statement that prints the value ofsum
using theprintf()
function.
- Return statement:
return 0;
is a statement that indicates the program has completed successfully and returns the value0
.
- Comments:
// Preprocessor directive
,// Main function
, and// Variable declaration and initialization
are single-line comments that explain what each section of the code does.
Example 3 :
#include <stdio.h> // Preprocessor directive
int global_var; // Global declaration
void my_function(); // Function declaration
int main() { // Main function
int local_var; // Local variable declaration
printf("Hello, world!\n"); // Statement
my_function(); // Function call
return 0;
}
void my_function() { // Function definition
printf("This is my function\n");
}
In this example, the program has the following sections:
- Preprocessor directives:
#include <stdio.h>
is a preprocessor directive that includes the standard input/output library in the program. - Global declarations:
int global_var;
is a global variable declaration. - Main function:
int main() { ... }
is the main function of the program, which is the entry point of the program. - Functions:
void my_function();
is a function declaration, andvoid my_function() { ... }
is a function definition. - Comments:
// Preprocessor directive
,// Global declaration
,// Main function
,// Local variable declaration
,// Statement
, and// Function definition
are comments that document each section of the code.
Files used in C Programming Language
Files are an important aspect of C programming, as they allow programs to read data from and write data to external sources such as text files, binary files, and even device files. Here are some of the commonly used files in C programming:
- Source code files: These are the files that contain the C source code for the program. Typically, a C program consists of one or more source code files with a .c extension.
- Header files: These files contain function prototypes, constant definitions, and other declarations that are shared across multiple source code files. Header files typically have a .h extension and are included in source code files using the #include preprocessor directive.
- Object files: These are the files that are generated by the compiler when the source code is compiled. Object files have a .o extension on Unix-based systems and a .obj extension on Windows.
- Library files: These are precompiled object files that contain reusable code and functions that can be linked into a program. Library files have a .lib extension on Windows and a .a extension on Unix-based systems.
- Input files: These are files that contain data that is read by the program at runtime. Input files can be text files, binary files, or even device files.
- Output files: These are files that are generated by the program at runtime. Output files can be text files, binary files, or even device files.
- Makefile: This is a file that specifies the dependencies between source code files and provides instructions for compiling and linking the program. Makefiles are commonly used in large projects to automate the build process.
Overall, files are an important aspect of C programming, as they allow programs to interact with external data sources and make programs more flexible and adaptable.
Compilers :
A compiler is a software tool that translates source code written in a programming language into machine code (binary code) that can be executed by a computer’s processor. The compiler takes the source code as input, analyzes it, and generates an executable program or library that can be used to run the program.
The compiler typically goes through several stages during the compilation process, including lexical analysis, syntax analysis, semantic analysis, code optimization, and code generation. During the lexical analysis stage, the compiler breaks the source code into a sequence of tokens based on the language syntax. During the syntax analysis stage, the compiler checks that the code is well-formed according to the language grammar. During the semantic analysis stage, the compiler checks for semantic errors in the code, such as type mismatches and undefined variables. During the code optimization stage, the compiler applies various optimization techniques to the code to improve its performance. Finally, during the code generation stage, the compiler produces machine code that can be executed by the computer.
There are various compilers available for different programming languages, and some popular compilers include:
- GCC (GNU Compiler Collection) – a free and open-source compiler that supports various programming languages including C, C++, and Objective-C.
- Clang – a free and open-source compiler that is designed to be highly compatible with GCC and supports various programming languages including C, C++, and Objective-C.
- Microsoft Visual C++ – a commercial compiler that is part of Microsoft’s Visual Studio development environment for Windows.
- Java Compiler – a compiler for Java programming language.
- Python Compiler – a compiler for Python programming language.
Choosing a compiler depends on several factors, including the programming language, the platform you’re targeting, the performance and optimization capabilities of the compiler, and the cost and licensing requirements of the compiler.
C Compiler
A C compiler is a software tool that translates source code written in the C programming language into machine code (binary code) that can be executed by a computer’s processor. The compiler is responsible for parsing the C code, checking for syntax and semantic errors, generating intermediate code, optimizing the code, and finally generating machine code.
There are several C compilers available, both free and commercial, for various platforms including Windows, Linux, and macOS. Some popular C compilers include:
- GCC (GNU Compiler Collection) – a free and open-source compiler that supports various programming languages including C, C++, and Objective-C.
- Clang – a free and open-source compiler that is designed to be highly compatible with GCC and supports various programming languages including C, C++, and Objective-C.
- Microsoft Visual C++ – a commercial compiler that is part of Microsoft’s Visual Studio development environment for Windows.
- Intel C++ Compiler – a commercial compiler that is designed to optimize code for Intel processors and supports various programming languages including C and C++.
- Turbo C++ – a popular compiler for the DOS operating system that was widely used in the 1990s.
When choosing a C compiler, it’s important to consider factors such as the platform you’re targeting, the performance and optimization capabilities of the compiler, and the cost and licensing requirements of the compiler.
Compiling and executing C program in Linux
- Open a terminal: Open a terminal in Linux. You can do this by clicking on the terminal icon in the system launcher or by using the keyboard shortcut Ctrl+Alt+T.
- Write the C program: Use a text editor such as Nano, Vim or Emacs to write the C program code. For this example, let’s create a simple “Hello World” program. Open a text editor and type in the following code:
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0;
}
3. Save the file with a .c extension: Save the file with a name of your choice and the extension “.c”. For example, let’s name the file “hello.c”.
4. Navigate to the directory containing the C program: Use the “cd” command to navigate to the directory where you saved the C program. For example, if the program is saved in your home directory, you can navigate there by typing:
cd ~
5. Compile the C program: To compile the C program, type “gcc” followed by the name of the C program file, then press Enter. For example, to compile the “hello.c” program, type:
gcc hello.c -o hello
This command tells the gcc compiler to compile the “hello.c” program and output the executable file with the name “hello”.
- Execute the C program: Once the C program has been compiled successfully, you can execute it by typing the name of the executable file that was created during the compilation process. For example, to execute the “hello” program, type:
./hello
Test the C program: If the C program runs without errors, you should see the output “Hello, world!” printed on the terminal.
Compiling and Running a C program using gedit in linux
Step 1: Open or create file using the command :
gedit filename.c
Step 2: Write a program and save the file.
Step 3: Compile the simple programs using the command :
cc filename.c (Note : one can also use gcc filename.c )
If the program contains math functions use the following command
cc filename.c -lm (Note : once can also use gcc filename.c –lm )
Step 4: Execute the program using the command : ./a.out
Compiling and Running a C program on Windows Turbo C:
Step 1: Locate the TC.exe file and open it. You will find it at location C:\TC\BIN\.
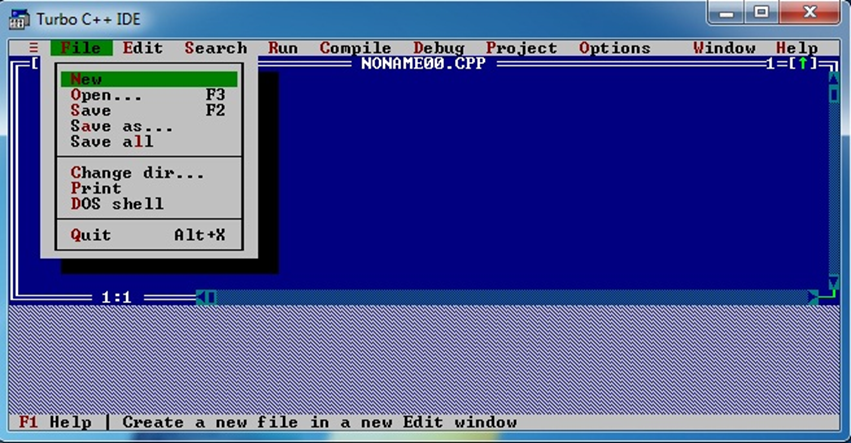
Step 2: File-> New (as shown in above picture) and then write your C program as given below
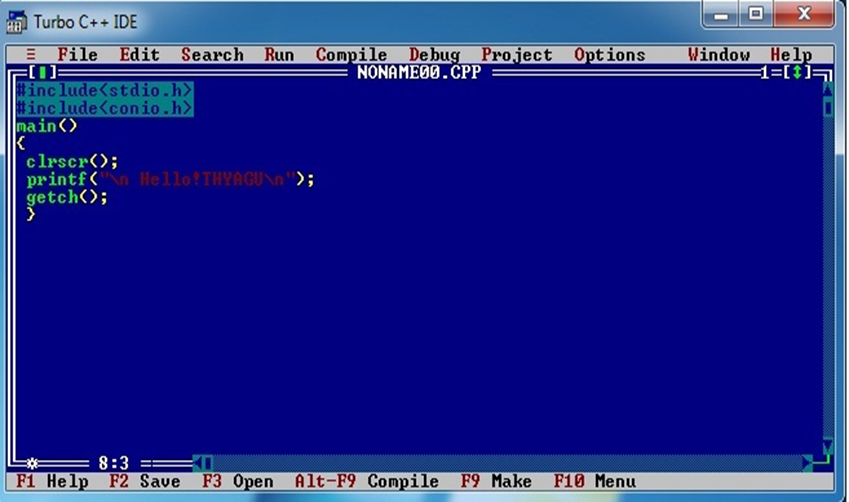
Step 3: Save the program using F2 (OR File-> Save as), remember the extension should be “.c”. In the below screenshot I have given the name as Hello.c.
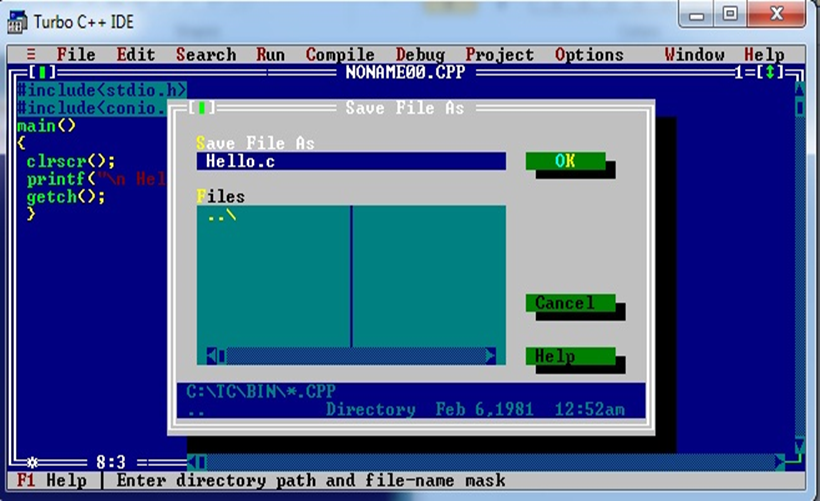
Step 4:Compile the program using Alt + F9 OR Compile-> Compile (as shown in the below screen shot).
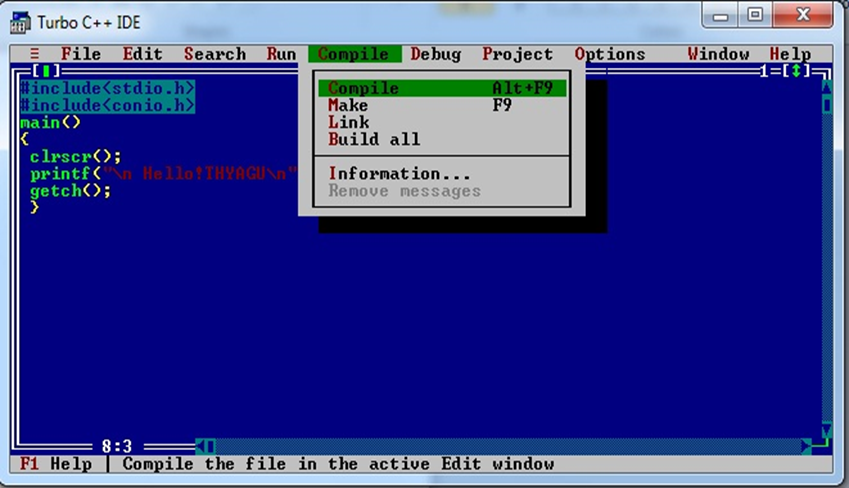
Step 5: Press Ctrl + F9 to Run (or select Run-> Run in menu bar ) the C program.
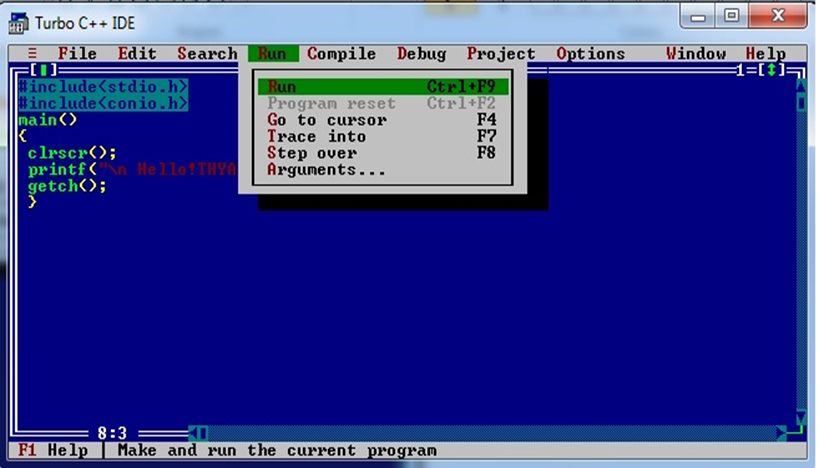
Step 6: Alt + F5 to view the output of the program at the output screen.

Questions for Practice