Syllabus : Functions, def Statements with Parameters, Return Values and return Statements, The None Value, Keyword Arguments and print(), Local and Global Scope, The global Statement, Exception Handling, A Short Program: Guess the Number.
Introduction
In Python, a function is a reusable block of code that performs a specific task. It is a self-contained unit of code that takes one or more inputs, performs a set of operations on those inputs, and returns an output.
There are several types of functions in Python, each with its own unique characteristics and use cases. Here are some of the most common types of functions:
- Built-in Functions: These are functions that are built into the Python language and are always available for use. Examples include
print()
,len()
, andtype()
. - User-defined Functions: These are functions that are created by the programmer to perform a specific task. They are defined using the
def
keyword and can take any number of arguments. - Lambda Functions: These are also known as anonymous functions because they are not given a name. They are defined using the
lambda
keyword and are often used for short, simple operations. - Recursive Functions: These are functions that call themselves repeatedly until a base case is reached. They are often used for tasks that can be broken down into smaller, identical tasks, such as sorting or searching.
- Higher-order Functions: These are functions that take other functions as arguments or return functions as output. They are often used in functional programming paradigms to create more abstract and flexible code.
- Generator Functions: These are functions that use the
yield
keyword to produce a sequence of values. Each time the function is called, it returns the next value in the sequence until there are no more values left to yield. - Decorator Functions: These are functions that modify the behavior of other functions. They are often used for tasks such as logging, caching, or authentication.
- Module Functions : In Python, a module is a file containing Python definitions and statements. The file name is the module name with the suffix
.py
. A module can define functions, classes, and variables, and can be used in other Python programs or modules using theimport
statement. A module function is a function defined within a module that can be accessed and used in other Python programs or modules by importing the module.
Understanding these different types of functions is essential for writing efficient, modular, and flexible code in Python.
A function is a group (or block ) of statements that perform a specific task . Functions run only when it is called. One can pass data into the function in the form of parameters. Function can also return data as a result. Instead of writing a large program as one long sequence of instructions, it can be written as several small function, each performing a specific part of the task. They constitute line of code(s) that are executed sequentially from top to bottom by Python interpreter. A python function is written once and is used / called as many time as required. Functions are the most important building blocks for any application in Python and work on the divide and conquer approach.
In this section following three types of functions will be discussed :
- User Defined Functions
- Built in Functions
- Module Functions
- User Defined Functions :
Functions that is defined by programmer to do certain specific task are referred as user-defined functions. The two main components of user defined functions are :
- Defining a Function
- Calling a Function
1. Defining a function: Function is defined using def keyword in Python.
def fun_name(comma_seperated_parameter_ list):
stmt1
stmt2
stmt3
-------
stmtn
return stmt
Statements below def begin with four spaces. This is called indentation. It is a requirement of Python that the code following a colon must be indented. A function definition consists of the following components:
- Keyword def marks the start of function header
- A function name to uniquely identify it. Function naming follows the same rules as rules of writing identifiers in Python.
- Parameters (arguments) through which we pass values to a function . They are optional.
- A colon ( : ) to mark the end of function header.
- Optional documentation string (docstring) to describe what the function does.
- One or more valid Python statements that make up the function body. Statements must have same indentation level (usually) 4 spaces.
- An optional return statement to return a value from the function .
Example : In the following example a function called cube(n) is defined to compute the cube of any number say n.
def cube(n):
ncube = n**3
return ncube
2. Calling a Function :
In the above example, we have declared a function named cube(n). Now, to use this function, we need to call it. Here’s how we can call the cube() function in Python.
x = cube(3)
print(x)
In the example illustrated below a function sqrsum (n1,n2 ) is defined to calculate and return the sum of squares of two numbers n1 and n2 . The returned value will be stored in the variable called sqsum by assigning the value obtained by calling the function sqrsum( ).
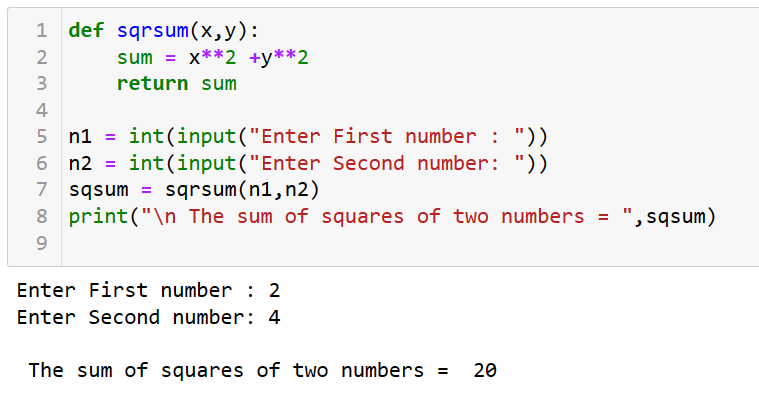
Working of A function
A function in Python is a self-contained block of code that performs a specific task. When a function is called, the program executes the code inside the function, using the input values provided as arguments. Here’s a step-by-step breakdown of how a function works:
- Function definition: First, the function must be defined using the
def
keyword, followed by the function name and the input parameters inside parentheses. The body of the function is then indented and contains the code that performs the desired task. - Function call: When the function is called, the program jumps to the function definition and executes the code inside. The input values provided as arguments are assigned to the corresponding input parameters in the function definition.
- Execution of the function code: The program executes the code inside the function, using the input values passed as arguments. The function can manipulate the input values, perform calculations, call other functions, and perform any other task required to achieve its purpose.
- Return value: If the function returns a value, it uses the
return
keyword to send the value back to the point in the program where the function was called. The returned value can then be assigned to a variable or used in any other way required. - Program flow: Once the function has completed its task and returned any necessary values, the program flow continues from the point where the function was called. If the function has modified any variables or performed any other operations, these changes will be reflected in the rest of the program.
Here’s an example of how to define and use a user-defined function in Python:
def add_numbers(x, y):
"""
This function takes two numbers as input and returns their sum.
"""
return x + y
result = add_numbers(2, 3)
print(result) # Output: 5
In this example, we define a function called add_numbers()
that takes two arguments (x
and y
) and returns their sum using the return
statement. We then call the function with the values 2
and 3
and store the result in the variable result
, which is printed to the console. Here’s another example of a user-defined function that calculates the area of a circle:
import math
def calculate_area_of_circle(radius):
"""
This function takes the radius of a circle as input and returns its area.
"""
area = math.pi * radius ** 2
return area
result = calculate_area_of_circle(5)
print(result) # Output: 78.53981633974483
In this example, we define a function called calculate_area_of_circle()
that takes the radius of a circle as an argument and calculates its area using the math.pi
constant and the **
operator to raise the radius to the power of 2. We then call the function with a radius of 5
and store the result in the variable result
, which is printed to the console.
User-defined functions are a powerful way to create reusable code that can be used in multiple parts of your program. By defining functions to perform specific tasks, you can make your code more organized, easier to read, and more maintainable over time.
Parameters and arguments
Parameters are temporary variable names within functions. The argument can be thought of as the value that is assigned to that temporary variable.
def cube(n):
ncube = n**3
return ncube
x = cube(3)
print(x)
- ‘n‘ here is the parameter for the function ‘cube‘. This means that anywhere we see ‘n‘ within the function will act as a placeholder until number is passed an argument.
- Here 3 is the argument.
- Parameters are used in function definition and arguments are used in function call.
In computer programming, a function is a self-contained block of code that performs a specific task. It takes inputs called arguments and produces outputs. Two important concepts related to functions are parameters and arguments. A parameter is a variable defined in the function’s declaration that specifies the data type and name of the expected input. Parameters act as placeholders for values that will be passed to the function when it is called.
An argument is a value or expression that is passed to a function when it is called. Arguments are passed in the order they are listed in the function’s parameter list. When a function is called, the argument’s value is assigned to the corresponding parameter, and the function uses the parameter to perform its task.
For example, consider the following function that calculates the area of a rectangle:
function calculateArea(width, height) {
return width * height;
}
In this function, width
and height
are parameters that specify the expected inputs of the function. When the function is called, actual values for width
and height
are passed as arguments, like this:
let area = calculateArea(10, 5);
In this example, 10
and 5
are arguments that are passed to the function. The function uses these arguments to calculate the area of a rectangle with a width of 10
and a height of 5
. The resulting value, 50
, is assigned to the variable area
.
In summary, parameters are defined in the function’s declaration and act as placeholders for the expected input, while arguments are the actual values passed to the function when it is called.
Types of Functions based on Parameters and Arguments
- Function without Parameters
A function without parameters is a function that does not take any arguments. It is defined with an empty set of parentheses. Here is an example:
def greet():
print("Hello, World!")
greet() # Output: Hello, World!
In this example, the function greet()
does not take any arguments. When we call this function with greet()
, it simply prints out “Hello, World!” to the console.
Functions without parameters can be useful when we need to perform a fixed set of actions that do not require any external input. For example, we could use a function without parameters to print a welcome message to the user when they start our program:
def welcome_message():
print("Welcome to our program! Let's get started.")
welcome_message() # Output: Welcome to our program! Let's get started.
Functions without parameters can also be used in conjunction with other functions that do take arguments. For example, we could define a function that calculates the average of a list of numbers, and then use a separate function without parameters to print out the result:
def calculate_average(numbers):
total = sum(numbers)
average = total / len(numbers)
return average
def print_average():
numbers = [2, 4, 6, 8, 10]
average = calculate_average(numbers)
print("The average of the numbers is:", average)
print_average() # Output: The average of the numbers is: 6.0
In this example, the calculate_average()
function takes a list of numbers as an argument and returns the average. The print_average()
function does not take any arguments, but it calls the calculate_average()
function to calculate the average of a fixed set of numbers and then prints out the result.
2. Function with parameters but without returning values.
A function with parameters but without returning values is a function that takes one or more arguments, performs some operations using those arguments, but does not return any value. Instead, it typically prints out the result to the console or performs some other action that does not involve returning a value. Here is an example:
def greet(name):
print("Hello, " + name + "!")
greet("John") # Output: Hello, John!
In this example, the function greet()
takes one parameter, name
, and prints out a greeting to the console that includes the value of the name
parameter. When we call this function with greet("John")
, it prints out “Hello, John!” to the console.
Functions with parameters but without returning values can be useful when we need to perform an action that does not involve returning a value. For example, we could use a function with parameters to perform some calculations and then print out the result:
def calculate_average(numbers):
total = sum(numbers)
average = total / len(numbers)
print("The average of the numbers is:", average)
numbers = [2, 4, 6, 8, 10]
calculate_average(numbers) # Output: The average of the numbers is: 6.0
In this example, the calculate_average()
function takes a list of numbers as an argument and calculates the average of those numbers. Instead of returning the average, it prints out the result to the console using the print()
function. We then call this function with a list of numbers [2, 4, 6, 8, 10]
to calculate the average and print it out to the console.
Functions with parameters but without returning values can also be used in conjunction with other functions that do return values. For example, we could define a function that takes two numbers as arguments, adds them together, and then passes the result to a separate function with parameters but without returning values that prints out the result:
def add_numbers(x, y):
return x + y
def print_result(result):
print("The result is:", result)
x = 5
y = 10
result = add_numbers(x, y)
print_result(result) # Output: The result is: 15
In this example, the add_numbers()
function takes two numbers as arguments, adds them together, and returns the result. The print_result()
function takes one parameter, result
, and prints out the result to the console using the print()
function. We then call the add_numbers()
function to add the numbers x
and y
and store the result in the variable result
. We pass this result to the print_result()
function to print it out to the console.
3. Function with parameters and return values
In Python, a function is a block of code that performs a specific task. It can take in parameters (also called arguments) and return a value or multiple values. A parameter is a value that is passed to a function when it is called, while a return value is the value that the function sends back to the caller.
Here’s an example of a function with parameters and a return value in Python
def multiply_numbers(x, y):
result = x * y
return result
In this example, the multiply_numbers
function takes two parameters, x
and y
, and returns their product, result
. To call the function and get the return value, you would use code like this:
product = multiply_numbers(3, 4)
print(product)
This code would output 12
, since the function multiplies 3 and 4 together to get the result of 12, which is then returned and stored in the product
variable. You can also pass in variables as the parameters, like this:
a = 5
b = 6
product = multiply_numbers(a, b)
print(product)
This would output 30
, since the function multiplies the values of a
and b
together to get the result of 30, which is then returned and stored in the product
variable.
4. Function which returns multiple values:
In Python, a function can return multiple values as a tuple. Here’s an example:
def calculate_circle(radius):
circumference = 2 * 3.14 * radius
area = 3.14 * (radius ** 2)
diameter = 2 * radius
return circumference, area, diameter
In this example, the calculate_circle
function takes a radius as a parameter and calculates the circumference, area, and diameter of a circle. Then, the function returns all three values as a tuple. To call this function and get all three return values, you can use the following code:
result = calculate_circle(5)
print(result)
This code will output (31.4, 78.5, 10)
which are the values for circumference, area, and diameter respectively, for a circle with radius of 5. You can also store each return value in separate variables like this:
circumference, area, diameter = calculate_circle(5)
print(circumference)
print(area)
print(diameter)
This code will output:
31.4
78.5
10
which are the values for circumference, area, and diameter respectively, for a circle with radius of 5.
The None-Value
In Python, None
is a special value that represents the absence of a value. It is often used to indicate that a variable or a function has no value or no effect. None is the only value of the None Type data type. (Other programming languages might call this value null, nil, or undefined.) Just like the Boolean True and False values, None must be typed with a capital N.
Here are some examples of using None
in Python:
- Assigning a variable to None:
x = None
This code assigns the value of None
to the variable x
.
- Using None as a default value in function arguments:
def my_function(arg1, arg2=None):
if arg2 is None:
print("arg2 has no value")
else:
print("arg2 has a value of:", arg2)
In this code, the my_function
function takes two arguments, arg1
and arg2
. If arg2
is not provided when calling the function, its default value is set to None
. The function then checks if arg2
has a value of None
and prints a message accordingly.
3. Returning None from a function:
def my_function():
# do some processing
return None
In this code, the my_function
function does some processing but does not return any value of significance. It returns None
to indicate that it has finished its task.
- Comparing to None:
x = None
if x is None:
print("x has no value")
In this code, the variable x
is compared to None
using the is
keyword. If x
has a value of None
, the message “x has no value” is printed.
Overall, None
is a useful value in Python for indicating the absence of a value, and it is commonly used in various contexts such as function arguments, return values, and variable assignments.
Keyword Arguments and print()
Keyword arguments are identified by the keyword put before them in the function call. Keyword arguments are often used for optional parameters. For example, the print( ) function has the optional parameters end and sep to specify what should be printed at the end of its arguments and between its arguments (separating them), respectively. Following examples illustrates the behavior of print with end , without end and with sep.
In Python, keyword arguments are a way to pass arguments to a function by specifying the argument name along with its value. The advantage of using keyword arguments is that they can make the function call more readable and self-documenting.
The print()
function in Python accepts keyword arguments to control its behavior. Here are some examples:
- Using the
end
keyword argument to specify the end character:
print("Hello", end=", ")
print("world!")
Output: Hello, world!
In this code, the end
keyword argument is used to specify that the print statement should end with a comma instead of a newline character.
2. Using the sep
keyword argument to specify the separator character:
print("apple", "banana", "cherry", sep=", ")
Output: apple, banana, cherry
In this code, the sep
keyword argument is used to specify that the separator between the items should be a comma followed by a space.
- Using both
end
andsep
keyword arguments:
print("The", "quick", "brown", "fox", sep="-", end=".")
Output: The-quick-brown-fox.
In this code, the sep
keyword argument is used to specify that the separator between the items should be a hyphen, and the end
keyword argument is used to specify that the print statement should end with a period instead of a newline character.
Keyword arguments can be very useful for controlling the behavior of functions, and they can make the code more readable and self-documenting.
Local and Global Scope
In Python, variables have different scopes, which determine where the variable can be accessed from. The two main scopes are local and global.
Local scope refers to variables that are defined inside a function. These variables can only be accessed from within the function, and they are destroyed when the function returns.
Here is an example:
def my_function():
x = 10
print("Inside the function, x =", x)
my_function()
Output: Inside the function, x = 10
In this code, the x
variable is defined inside the my_function
function, and it can only be accessed from within the function. When the function is called, the value of x
is printed, and then the variable is destroyed when the function returns.
Global scope refers to variables that are defined outside of any function. These variables can be accessed from anywhere in the code, including inside functions.
Here is an example:
x = 10
def my_function():
print("Inside the function, x =", x)
my_function()
print("Outside the function, x =", x)
Output:
Inside the function, x = 10
Outside the function, x = 10
In this code, the x
variable is defined outside of the my_function
function, so it has global scope. The function can access the value of x
, and the variable can also be accessed outside of the function.
It’s important to note that if a variable with the same name is defined both locally and globally, the local variable takes precedence within the function. For example:
x = 10
def my_function():
x = 5
print("Inside the function, x =", x)
my_function()
print("Outside the function, x =", x)
Output:
Inside the function, x = 5
Outside the function, x = 10
In this code, the x
variable is defined both globally and locally. Inside the my_function
function, the local variable x
takes precedence, so the value of x
printed inside the function is 5. However, outside the function, the global variable x
is still 10.
Following example illustrates the difference between local and global variable .
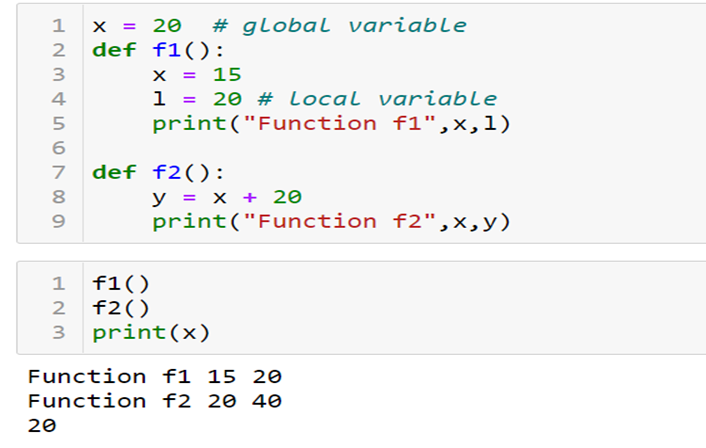
Local variable cannot be used in the global scope
Consider this program which will cause an error when you run it:
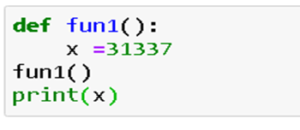
If you run this program the output will look like this
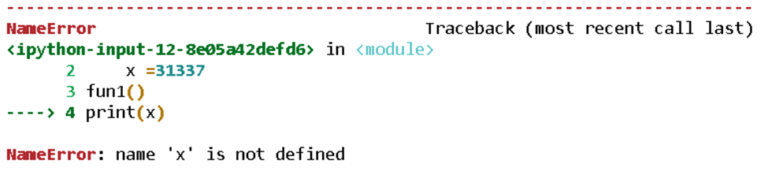
The error happens because the x variable exists only in the local scope created when fun1() is called. Once the program execution returns from fun1(), that local scope is destroyed, and there is no longer a variable named x. So when your program tries to run print(x), Python gives you an error saying that x is not defined. This makes sense if you think about it; when the program execution is in the global scope, no local scopes exist, so there can’t be any local variables. This is why only global variables can be used in the global scope.
Local Scopes Cannot Use Variables in Other Local Scopes
A new local scope is created whenever a function is called, including when a function is called from another function. Consider this program:
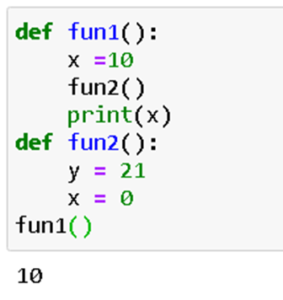
When the program starts the func1() is called and a local scope is created . The local variable x is set to 10. Then fun2() is called and a second local scope is created . Multiple local scopes can exist at the same time . In this new local scope , the local variable y is set to 21 and a local variable x which is different from the one in fun1()’s local scope is also created and set to 0. When fun2() returns the local scope for the call to fun1() still exists here the x variable is set to 10. This is what the programs prints.
The local variables in one function are completely separate the local variable in another function.
Global Variable Can be read from a local scope :
Consider the following program :
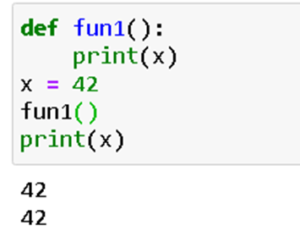
Since there is no parameter named x or any code that assigns x a value in the fun1() function , when x is used in fun1(), Python considers it a reference to the global variable x. This is why 42 is printed when the previous program is run.
Local and Global Variables with the same Name :
One should avoid using local variables that have the same name as a global variable or another local variable. In Python, if a variable with the same name exists both in the local and global scope, then the local variable takes precedence over the global variable within the function. Here’s an example:
x = 10 # Global variable
def my_function():
x = 5 # Local variable with the same name
print("Inside the function, x =", x)
my_function()
print("Outside the function, x =", x)
Output :
Inside the function, x = 5
Outside the function, x = 10
In this example, there is a global variable x
with the value of 10. Inside the my_function
function, a local variable x
is defined with the value of 5. When the function is called, the value of the local variable x
is printed, which is 5. Outside the function, the global variable x
is still 10, because the local variable x
only exists within the function. To access the global variable with the same name within the function, you can use the global
keyword to declare the variable as global. Here’s an example:
x = 10 # Global variable
def my_function():
global x
x = 5 # Updating the global variable with the same name
print("Inside the function, x =", x)
my_function()
print("Outside the function, x =", x)
Output:
Inside the function, x = 5
Outside the function, x = 5
In this example, the global
keyword is used to declare the x
variable as global within the function. This allows us to modify the global variable x
with the same name. When the function is called, the value of the global variable x
is updated to 5, and then the updated value is printed both inside and outside the function.
Global Statement
In Python, the global
statement is used to indicate that a variable is a global variable, and not a local variable within a function.When you define a variable inside a function, by default, it is considered as a local variable. This means that the variable can only be accessed within the function where it was defined. However, if you want to use the variable outside the function, you can use the global
statement to make it a global variable. Here’s an example:
x = 10 # global variable
def my_function():
global x
x = 5 # updating the global variable
print("Inside the function, x =", x)
my_function()
print("Outside the function, x =", x)
Output:
Inside the function, x = 5
Outside the function, x = 5
In this example, the global
statement is used to declare x
as a global variable within the function my_function
. This allows us to modify the global variable x
with the same name. When the function is called, the value of the global variable x
is updated to 5, and then the updated value is printed both inside and outside the function.
It’s important to note that the global
statement should be used with caution. Modifying global variables inside functions can lead to unexpected behavior, especially in large programs where it can be difficult to keep track of all the variables. It’s generally better to use local variables inside functions whenever possible, and only use global variables when absolutely necessary.
There are four rules to tell whether a variable is in a local scope or global scope:
1. If a variable is being used in the global scope (that is, outside of all functions), then it is always a global variable.
2. If there is a global statement for that variable in a function, it is a global variable.
3. Otherwise, if the variable is used in an assignment statement in the function, it is a local variable.
4. But if the variable is not used in an assignment statement, it is a global variable.
Exceptional Handling
Right now, getting an error, or exception, in your Python program means the entire program will crash. You don’t want this to happen in real-world programs. Instead, you want the program to detect errors, handle them, and then continue to run. For example, consider the following program, which has a “divide-byzero” error. Open a new file editor window and enter the following code, saving it as zeroDivide.py:
Exception handling in Python allows you to gracefully handle errors that might occur during the execution of your program. An exception is an error that occurs during the execution of a program, and it can cause the program to terminate abruptly.
Python provides a built-in mechanism for handling exceptions using the try-except statement. Here is an example:
try:
# code that might raise an exception
except ExceptionType:
# code to handle the exception
In this example, the code that might raise an exception is contained within the try block. If an exception of type ExceptionType
is raised, then the code within the except block will be executed. Here is an example that demonstrates how to catch a specific exception:
try:
x = 1 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
In this example, a ZeroDivisionError exception will be raised when we try to divide by zero. The except block catches this exception and prints a message indicating that we cannot divide by zero. You can also catch multiple exceptions by specifying them in a tuple:
try:
# code that might raise an exception
except (ExceptionType1, ExceptionType2):
# code to handle the exception
Finally, you can catch all exceptions by using the Exception class:
try:
# code that might raise an exception
except Exception:
# code to handle the exception
It is important to handle exceptions properly to prevent your program from crashing unexpectedly.
Right now, getting an error, or exception, in your Python program means the entire program will crash. You don’t want this to happen in real-world programs. Instead, you want the program to detect errors, handle them, and then continue to run. For example, consider the following program, which has a “divide-byzero” error. Open a new file editor window and enter the following code, saving it as zeroDivide.py:
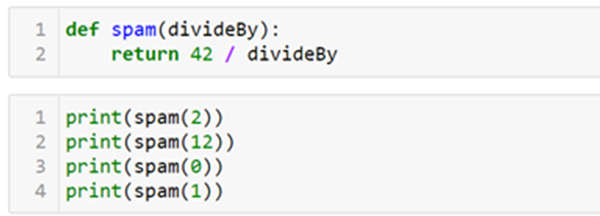
We’ve defined a function called spam, given it a parameter, and then printed the value of that function with various parameters to see what happens. This is the output you get when you run the previous code:
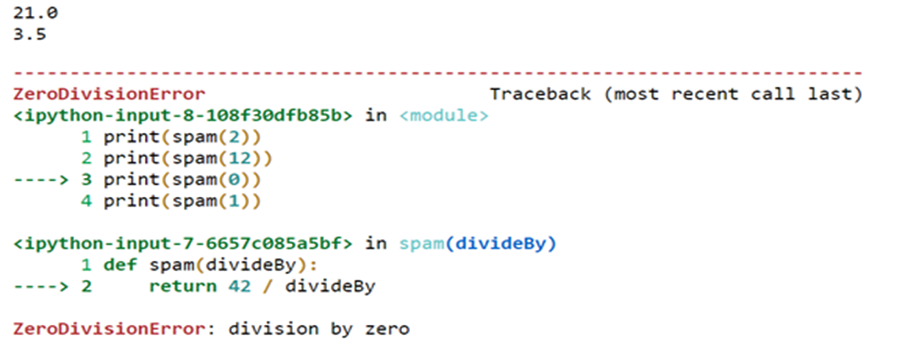
A ZeroDivisionError happens whenever you try to divide a number by zero. From the line number given in the error message, you know that the return statement in spam() is causing an error.
Try and Except :
Errors can be handled with try and except statements. The code that could potentially have an error is put in a try clause. The program execution moves to the start of a following except clause if an error happens. You can put the previous divide-by-zero code in a try clause and have an except clause contain code to handle what happens when this error occurs.
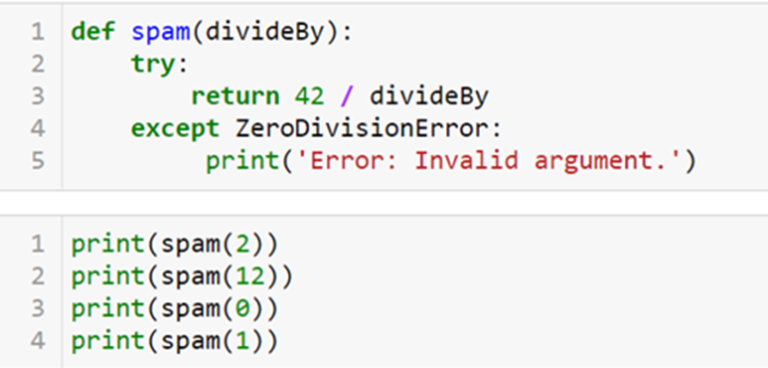
When code in a try clause causes an error, the program execution immediately moves to the code in the except clause. After running that code, the execution continues as normal. The output of the previous program is as follows:
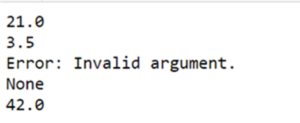
Note that any errors that occur in function calls in a try block will also be caught. Consider the following program, which instead has the spam() calls in the try block:
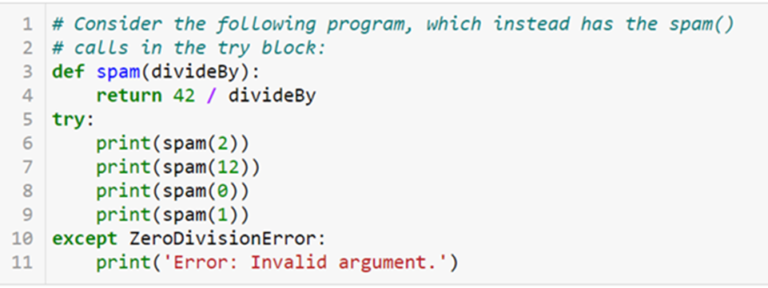
When this program is run, the output looks like this:
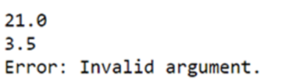
The reason print(spam(1)) is never executed is because once the execution jumps to the code in the except clause, it does not return to the try clause. Instead, it just continues moving down as normal.
ii. Built in functions
Built in functions are the predefined functions that are already available in python. Functions provide efficiency and structure to a programming language . Python has many useful built in functions to make programming easier , faster and more powerful.In Python, there are a large number of built-in functions that are available for use without the need to import any modules. These functions provide a wide range of functionality, including mathematical operations, string manipulation, data type conversion, and much more.
Some of the important built-in functions are listed below :
abs() : Returns the absolute value of a number ascii() : Returns a readable version of an object. Replaces none-ascii characters with escape character bin() : Returns the binary version of a number bool() : Returns the boolean value of the specified object bytearray() :Returns an array of bytes bytes() : Returns a bytes object chr(): Returns a character from the specified Unicode code. classmethod(): Converts a method into a class method complex():Returns a complex number delattr(): Deletes the specified attribute (property or method) from the specified object dict(): Returns a dictionary (Array) dir(): Returns a list of the specified object's properties and methods divmod(): Returns the quotient and the remainder when argument1 is divided by argument2 enumerate(): Takes a collection (e.g. a tuple) and returns it as an enumerate object eval(): Evaluates and executes an expression filter(): Use a filter function to exclude items in an iterable object float() Returns a floating point number format(): Formats a specified value getattr(): Returns the value of the specified attribute (property or method) globals(): Returns the current global symbol table as a dictionary hex(): Converts a number into a hexadecimal value id(): Returns the id of an object input(): Allowing user input int(): Returns an integer number isinstance(): Returns True if a specified object is an instance of a specified object issubclass(): Returns True if a specified class is a subclass of a specified object iter(): Returns an iterator object len(): Returns the length of an object list():Returns a list locals():Returns an updated dictionary of the current local symbol table map(): Returns the specified iterator with the specified function applied to each item max(): Returns the largest item in an iterable min(): Returns the smallest item in an iterable next(): Returns the next item in an iterable object(): Returns a new object oct():Converts a number into an octal open(): Opens a file and returns a file object ord():Convert an integer representing the Unicode of the specified character pow(): Returns the value of x to the power of y print():Prints to the standard output device property(): Gets, sets, deletes a property range(): Returns a sequence of numbers, starting from 0 and increments by 1 (by default) round(): Rounds a numbers set(): Returns a new set object setattr():Sets an attribute (property/method) of an object slice(): Returns a slice object sorted(): Returns a sorted list str(): Returns a string object sum(): Sums the items of an iterator tuple():Returns a tuple type(): Returns the type of an object vars(): Returns the __dict__ property of an object zip(): Returns an iterator, from two or more iterators
here’s an example program that lists out all the built-in functions in Python:
import builtins
for func in dir(builtins):
print(func)
In this program, we import the builtins
module and use the dir()
function to get a list of all the names defined in the module. We then loop through the list and print out each name, which corresponds to a built-in function. Note that this will list all the built-in functions in Python, including those that are rarely used. To narrow down the list to functions that are more commonly used, you can filter the output based on your needs. Also note that the dir()
function also returns other objects besides function names, such as variables and classes, so you might want to filter out anything that is not a function. For example, you could modify the above code like this to only print out function names:
import builtins
for func in dir(builtins):
if callable(getattr(builtins, func)):
print(func)
This version of the program uses the callable()
function to check whether each object returned by dir()
is a callable function, and only prints out the names of functions that pass the test.
Questions for Practice:
- What are the advantages of using functions in a program?
- When the code in the function does executes during definition or calling.
- Discuss How to create a function with example.
- Explain the following with example
- Functions
- Function Call
- Built in Function
- Type Conversion Functions
- Random Numbers
- Math Functions
- Adding new Functions
- Defining and using the new functions
- Flow of execution
- Parameter and Arguments
- Fruitful and void Functions
- Advantages of Functions
- Differentiate between argument and parameter.
- What is the difference between a function and a function call?
- How many global scopes and local scopes are there in Python program?
- What happens to variables in a local scope when the function call returns?
- What is a return value? Can a return value be part of an expression?
- What is the return value of the function which does not have return statement?
- How can you force a variable in a function to refer to the global variable?
- What is the data type of None?
- What does the import allname statement do?
- If you had a function named radio() in a module named car who would you call it after importing car.
- How can you prevent a program from crashing when it gets an error?
- What goes in the try clause? What goes in the except clause?
- Illustrate the flow of execution of a python function with an example program to convert given Celsius to Fahrenheit temperature.
- Explain the function arguments in python.
- Explain call by value and call by reference in python
- Briefly explain about function prototypes
- Define the scope and lifetime of a variable in python
- Point out the uses of default arguments in python
- Generalize the uses of python module.
- Demonstrate how a function calls another function. Justify your Apply answer
- List the syntax for function call with and without arguments.
- Define recursive function
- Define the syntax for passing arguments.
- What are the two parts of function definition give the syntax
- Briefly discuss in detail about function prototyping in python. With suitable example program
- Analyze the difference between local and global variables.
- Explain with an example program to circulate the values of n variables
- Describe in detail about lambda functions or anonymous function.
- Describe in detail about the rules to be followed while using Lambda function.
- Explain with an example program to return the average of its argument
- Explain the various features of functions in python.
- Describe the syntax and rules involved in the return statement in python.
- Write a program to demonstrate the flow of control after the return statement in python.
- Formulate with an example program to pass the list arguments to a function.
- Write a program to perform selection sort from a list of numbers using python.
- Give the use of return () statement with a suitable example.
- What are the advantages and disadvantages of recursion function? A
- Explain the types of function arguments in python
- Explain recursive function. How do recursive function works? Explain with a help of a program
- Illustrate the concept of local and global variables.
- A polygon can be represented by a list of (x, y) pairs where each pair is a tuple: [ (x1, y1), (x2, y2), (x3, y3) , … (xn, yn)]. Write a Recursive function to compute the area of a polygon. This can be accomplished by “cutting off” a triangle, using the fact that a triangle with corners (x1, y1), (x2, y2), (x3, y3) has area (x1y1 + x2y2 + x3y2 – y1x2 –y2x3 – y3x1) / 2.
- What is a lambda function?
- How Do We Write A Function In Python?
- What Is “Call By Value” In Python?
- What Is “Call By Reference” In Python?
- Is It Mandatory For A Python Function To Return A Value? Comment?
- What Does The *Args Do In Python?
- What Does The **Kwargs Do In Python?
- Does Python Have A Main() Method?
- What Is The Purpose Of “End” In Python?
- What Does The Ord() Function Do In Python?
- What are split(), sub(), and subn() methods in Python?
- Describe the syntax for the following functions and explain with an example: a) abs() b) max() c) divmod() d) pow() e) len()
- What are functions in Python and why are they useful?
- How do you define a function in Python and what is the syntax for it?
- What is the difference between parameters and arguments in Python functions?
- How do you call a function in Python and what is the syntax for it?
- What is the difference between a return statement and a print statement in Python functions?
- What is the purpose of docstrings in Python functions and how do you use them?
- What are default arguments in Python functions and how do you use them?
- What are keyword arguments in Python functions and how do you use them?
- What is variable scope in Python and how does it relate to functions?
- What are lambda functions in Python and how do you use them?
- What are recursive functions in Python and how do you use them?
- What is a higher-order function in Python and how does it relate to functions as first-class objects?
- What are decorators in Python and how do you use them to modify the behavior of a function?
- What is the
map()
function in Python and how can it be used to apply a function to every element in a list? - What is the
filter()
function in Python and how can it be used to filter elements from a list based on a condition? - What is the
reduce()
function in Python and how can it be used to reduce a list to a single value using a specified function? - What is the
zip()
function in Python and how can it be used to combine two or more lists into a single list of tuples? - What is the
enumerate()
function in Python and how can it be used to iterate over a list with both the index and the value of each element? - What is the
sorted()
function in Python and how can it be used to sort a list or other iterable? - What is the
any()
andall()
functions in Python and how can they be used to test if any or all elements in an iterable are true?
Programs for Practice:
- Write a program to generate Fibonacci series upto the given limit FIBONACCI(n) function.
- Write a single user defined function named ‘Solve’ that returns the Remainder and Quotient separately on the Console.
- Write a program to find i) The largest of three numbers and ii) check whether the given year is leap year or not with functions.
- Find the area and perimeter of a circle using functions. Prompt the user for input
- Write a Python program using functions to find the value of nPr and nCr without using inbuilt factorial() function.
- Write a program to find the product of two matrices.
- A prime number is an integer greater than 1 that is evenly divisible by only 1 and itself. For example, the number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided by 1, 2, 3, and 6.
- Write a function named isPrime, which takes an integer as an argument and returns True if the argument is a prime number, and False otherwise. Define a function main() and call isPrime() function in main() to display a list of the prime numbers from 100 to 500.
- Write a program that lets the user perform arithmetic operations on two numbers. Your program must be menu driven, allowing the user to select the operation (+, -, *, or /) and input the numbers. Furthermore, your program must consist of following functions:
- Function showChoice: This function shows the options to the user and explains how to enter data.
- Function add: This function accepts two number as arguments and returns sum.
- Function subtract: This function accepts two number as arguments and returns their difference.
- Function mulitiply: This function accepts two number as arguments and returns product.
- Function divide: This function accepts two number as arguments and returns quotient. Define a function main() and call functions in main().
- Write a Python function for the following :
- To find the Max of three numbers.
- To sum all the numbers in a listTo multiply all the numbers in a list.
- To reverse a stringTo calculate the factorial of a number (a non-negative integer).
- The function accepts the number as an argument
- To check whether a number is in a given range
- That accepts a string and calculate the number of upper case letters and lower case letters.
- That takes a list and returns a new list with unique elements of the first list.
- That takes a number as a parameter and check the number is prime or not.
- To print the even numbers from a given list
- To check whether a number is perfect or not.
- That checks whether a passed string is palindrome or not.
- That prints out the first n rows of Pascal’s triangle.
- Write a Python function to create and print a list where the values are square of numbers between 1 and 30 (both included).
- Write a Python program to make a chain of function decorators (bold, italic, underline etc.) in Python.
- Write a Python program to execute a string containing Python code
- Write a Python program to access a function inside a function
- Write a Python program to detect the number of local variables declared in a function.
- Write a function calculation() such that it can accept two variables and calculate the addition and subtraction of it. And also it must return both addition and subtraction in a single return call
- Create a function showEmployee() in such a way that it should accept employee name, and it’s salary and display both, and if the salary is missing in function call it should show it as 9000
- Create an inner function to calculate the addition in the following way
- Create an outer function that will accept two parameters a and b
- Create an inner function inside an outer function that will calculate the addition of a and b
- At last, an outer function will add 5 into addition and return it
- Write a recursive function to calculate the sum of numbers from 0 to 10
- Write a function to calculate area and perimeter of a rectangle.
- Write a function to calculate area and circumference of a circle.
- Write a function to calculate power of a number raised to other. E.g.- ab.
- Write a function to tell user if he/she is able to vote or not.( Consider minimum age of voting to be 18. )
- Print multiplication table of 12 using recursion.
- Write a function to calculate power of a number raised to other ( ab ) using recursion.
- Write a function “perfect()” that determines if parameter number is a perfect number. Use this function in a program that determines and prints all the perfect numbers between 1 and 1000.[An integer number is said to be “perfect number” if its factors, including 1(but not the number itself), sum to the number. E.g., 6 is a perfect number because 6=1+2+3].
- Write a function to check if a number is even or not.
- Write a function to check if a number is prime or not.
- Write a function to find factorial of a number but also store the factorials calculated in a dictionary as done in the Fibonacci series example.
- Write a function to calculate area and perimeter of a rectangle.
- What is the output of the following code segments ?